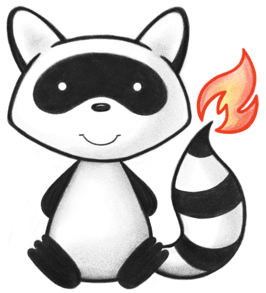
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.interceptor.api; 021 022import ca.uhn.fhir.model.base.resource.BaseOperationOutcome; 023import ca.uhn.fhir.rest.annotation.Read; 024import ca.uhn.fhir.rest.annotation.Search; 025import ca.uhn.fhir.rest.server.exceptions.AuthenticationException; 026import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 027import ca.uhn.fhir.validation.ValidationResult; 028import jakarta.annotation.Nonnull; 029import org.apache.commons.lang3.Validate; 030import org.hl7.fhir.instance.model.api.IBaseConformance; 031 032import java.io.Writer; 033import java.util.Arrays; 034import java.util.Collections; 035import java.util.HashSet; 036import java.util.List; 037import java.util.Set; 038 039import static ca.uhn.fhir.interceptor.api.IInterceptorBroadcaster.*; 040 041/** 042 * Value for {@link Hook#value()} 043 * <p> 044 * Hook pointcuts are divided into several broad categories: 045 * <ul> 046 * <li>INTERCEPTOR_xxx: Hooks on the interceptor infrastructure itself</li> 047 * <li>CLIENT_xxx: Hooks on the HAPI FHIR Client framework</li> 048 * <li>SERVER_xxx: Hooks on the HAPI FHIR Server framework</li> 049 * <li>SUBSCRIPTION_xxx: Hooks on the HAPI FHIR Subscription framework</li> 050 * <li>STORAGE_xxx: Hooks on the storage engine</li> 051 * <li>VALIDATION_xxx: Hooks on the HAPI FHIR Validation framework</li> 052 * <li>JPA_PERFTRACE_xxx: Performance tracing hooks on the JPA server</li> 053 * </ul> 054 * </p> 055 */ 056public enum Pointcut implements IPointcut { 057 058 /** 059 * <b>Interceptor Framework Hook:</b> 060 * This pointcut will be called once when a given interceptor is registered 061 */ 062 INTERCEPTOR_REGISTERED(void.class), 063 064 /** 065 * <b>Client Hook:</b> 066 * This hook is called before an HTTP client request is sent 067 * <p> 068 * Hooks may accept the following parameters: 069 * <ul> 070 * <li> 071 * ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request 072 * </li> 073 * <li> 074 * ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request 075 * </li> 076 * </ul> 077 * </p> 078 * Hook methods must return <code>void</code>. 079 */ 080 CLIENT_REQUEST( 081 void.class, "ca.uhn.fhir.rest.client.api.IHttpRequest", "ca.uhn.fhir.rest.client.api.IRestfulClient"), 082 083 /** 084 * <b>Client Hook:</b> 085 * This hook is called after an HTTP client request has completed, prior to returning 086 * the results to the calling code. Hook methods may modify the response. 087 * <p> 088 * Hooks may accept the following parameters: 089 * <ul> 090 * <li> 091 * ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request 092 * </li> 093 * <li> 094 * ca.uhn.fhir.rest.client.api.IHttpResponse - The details of the response 095 * </li> 096 * <li> 097 * ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request 098 * </li> 099 * <li> 100 * ca.uhn.fhir.rest.client.api.ClientResponseContext - Contains an IHttpRequest, an IHttpResponse, and an IRestfulClient 101 * and also allows the client to mutate the contained IHttpResponse 102 * </li> 103 * </ul> 104 * </p> 105 * Hook methods must return <code>void</code>. 106 */ 107 CLIENT_RESPONSE( 108 void.class, 109 "ca.uhn.fhir.rest.client.api.IHttpRequest", 110 "ca.uhn.fhir.rest.client.api.IHttpResponse", 111 "ca.uhn.fhir.rest.client.api.IRestfulClient", 112 "ca.uhn.fhir.rest.client.api.ClientResponseContext"), 113 114 /** 115 * <b>Server Hook:</b> 116 * This hook is called when a server CapabilityStatement is generated for returning to a client. 117 * <p> 118 * This pointcut will not necessarily be invoked for every client request to the `/metadata` endpoint. 119 * If caching of the generated CapabilityStatement is enabled, a new CapabilityStatement will be 120 * generated periodically and this pointcut will be invoked at that time. 121 * </p> 122 * <p> 123 * Hooks may accept the following parameters: 124 * <ul> 125 * <li> 126 * org.hl7.fhir.instance.model.api.IBaseConformance - The <code>CapabilityStatement</code> resource that will 127 * be returned to the client by the server. Interceptors may make changes to this resource. The parameter 128 * must be of type <code>IBaseConformance</code>, so it is the responsibility of the interceptor hook method 129 * code to cast to the appropriate version. 130 * </li> 131 * <li> 132 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to 133 * be processed 134 * </li> 135 * <li> 136 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that 137 * is about to be processed. This parameter is identical to the RequestDetails parameter above but will only 138 * be populated when operating in a RestfulServer implementation. It is provided as a convenience. 139 * </li> 140 * </ul> 141 * </p> 142 * Hook methods may an instance of a new <code>CapabilityStatement</code> resource which will replace the 143 * one that was supplied to the interceptor, or <code>void</code> to use the original one. If the interceptor 144 * chooses to modify the <code>CapabilityStatement</code> that was supplied to the interceptor, it is fine 145 * for your hook method to return <code>void</code> or <code>null</code>. 146 */ 147 SERVER_CAPABILITY_STATEMENT_GENERATED( 148 IBaseConformance.class, 149 "org.hl7.fhir.instance.model.api.IBaseConformance", 150 "ca.uhn.fhir.rest.api.server.RequestDetails", 151 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 152 153 /** 154 * <b>Server Hook:</b> 155 * This hook is called before any other processing takes place for each incoming request. It may be used to provide 156 * alternate handling for some requests, or to screen requests before they are handled, etc. 157 * <p> 158 * Note that any exceptions thrown by this method will not be trapped by HAPI (they will be passed up to the server) 159 * </p> 160 * <p> 161 * Hooks may accept the following parameters: 162 * <ul> 163 * <li> 164 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 165 * </li> 166 * <li> 167 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 168 * </li> 169 * </ul> 170 * </p> 171 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 172 * This is generally the right thing to do. If your interceptor is providing a response rather than 173 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 174 * no further processing will occur and no further interceptors will be called. 175 */ 176 SERVER_INCOMING_REQUEST_PRE_PROCESSED( 177 boolean.class, "jakarta.servlet.http.HttpServletRequest", "jakarta.servlet.http.HttpServletResponse"), 178 179 /** 180 * <b>Server Hook:</b> 181 * This hook is invoked upon any exception being thrown within the server's request processing code. This includes 182 * any exceptions thrown within resource provider methods (e.g. {@link Search} and {@link Read} methods) as well as 183 * any runtime exceptions thrown by the server itself. This also includes any {@link AuthenticationException} 184 * thrown. 185 * <p> 186 * Hooks may accept the following parameters: 187 * <ul> 188 * <li> 189 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 190 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 191 * pulled out of the servlet request. Note that the bean 192 * properties are not all guaranteed to be populated, depending on how early during processing the 193 * exception occurred. 194 * </li> 195 * <li> 196 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 197 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 198 * pulled out of the servlet request. Note that the bean 199 * properties are not all guaranteed to be populated, depending on how early during processing the 200 * exception occurred. This parameter is identical to the RequestDetails parameter above but will 201 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 202 * </li> 203 * <li> 204 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 205 * </li> 206 * <li> 207 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 208 * </li> 209 * <li> 210 * ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException - The exception that was thrown 211 * </li> 212 * </ul> 213 * </p> 214 * <p> 215 * Implementations of this method may choose to ignore/log/count/etc exceptions, and return <code>true</code> or 216 * <code>void</code>. In 217 * this case, processing will continue, and the server will automatically generate an {@link BaseOperationOutcome 218 * OperationOutcome}. Implementations may also choose to provide their own response to the client. In this case, they 219 * should return <code>false</code>, to indicate that they have handled the request and processing should stop. 220 * </p> 221 */ 222 SERVER_HANDLE_EXCEPTION( 223 boolean.class, 224 "ca.uhn.fhir.rest.api.server.RequestDetails", 225 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 226 "jakarta.servlet.http.HttpServletRequest", 227 "jakarta.servlet.http.HttpServletResponse", 228 "ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException"), 229 230 /** 231 * <b>Server Hook:</b> 232 * This method is immediately before the handling method is selected. Interceptors may make changes 233 * to the request that can influence which handler will ultimately be called. 234 * <p> 235 * Hooks may accept the following parameters: 236 * <ul> 237 * <li> 238 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 239 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 240 * pulled out of the servlet request. 241 * Note that the bean properties are not all guaranteed to be populated at the time this hook is called. 242 * </li> 243 * <li> 244 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 245 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 246 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 247 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 248 * </li> 249 * <li> 250 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 251 * </li> 252 * <li> 253 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 254 * </li> 255 * </ul> 256 * <p> 257 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 258 * This is generally the right thing to do. 259 * If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you 260 * must return <code>false</code>. In this case, no further processing will occur and no further interceptors 261 * will be called. 262 * </p> 263 * <p> 264 * Hook methods may also throw {@link AuthenticationException} if they would like. This exception may be thrown 265 * to indicate that the interceptor has detected an unauthorized access 266 * attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. 267 * 268 * @since 5.4.0 269 */ 270 SERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED( 271 boolean.class, 272 "ca.uhn.fhir.rest.api.server.RequestDetails", 273 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 274 "jakarta.servlet.http.HttpServletRequest", 275 "jakarta.servlet.http.HttpServletResponse"), 276 277 /** 278 * <b>Server Hook:</b> 279 * This method is called just before the actual implementing server method is invoked. 280 * <p> 281 * Hooks may accept the following parameters: 282 * <ul> 283 * <li> 284 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 285 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 286 * pulled out of the servlet request. Note that the bean 287 * properties are not all guaranteed to be populated, depending on how early during processing the 288 * exception occurred. 289 * </li> 290 * <li> 291 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 292 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 293 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 294 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 295 * </li> 296 * <li> 297 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 298 * </li> 299 * <li> 300 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 301 * </li> 302 * </ul> 303 * <p> 304 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 305 * This is generally the right thing to do. 306 * If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you 307 * must return <code>false</code>. In this case, no further processing will occur and no further interceptors 308 * will be called. 309 * </p> 310 * <p> 311 * Hook methods may also throw {@link AuthenticationException} if they would like. This exception may be thrown 312 * to indicate that the interceptor has detected an unauthorized access 313 * attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. 314 */ 315 SERVER_INCOMING_REQUEST_POST_PROCESSED( 316 boolean.class, 317 "ca.uhn.fhir.rest.api.server.RequestDetails", 318 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 319 "jakarta.servlet.http.HttpServletRequest", 320 "jakarta.servlet.http.HttpServletResponse"), 321 322 /** 323 * <b>Server Hook:</b> 324 * This hook is invoked before an incoming request is processed. Note that this method is called 325 * after the server has begun preparing the response to the incoming client request. 326 * As such, it is not able to supply a response to the incoming request in the way that 327 * SERVER_INCOMING_REQUEST_PRE_PROCESSED and {@link #SERVER_INCOMING_REQUEST_POST_PROCESSED} are. 328 * At this point the request has already been passed to the handler so any changes 329 * (e.g. adding parameters) will not be considered. 330 * If you'd like to modify request parameters before they are passed to the handler, 331 * use {@link Pointcut#SERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED} or {@link Pointcut#SERVER_INCOMING_REQUEST_POST_PROCESSED}. 332 * If you are attempting to modify a search before it occurs, use {@link Pointcut#STORAGE_PRESEARCH_REGISTERED}. 333 * <p> 334 * Hooks may accept the following parameters: 335 * <ul> 336 * <li> 337 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 338 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 339 * pulled out of the servlet request. Note that the bean 340 * properties are not all guaranteed to be populated, depending on how early during processing the 341 * exception occurred. 342 * </li> 343 * <li> 344 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 345 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 346 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 347 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 348 * </li> 349 * <li> 350 * ca.uhn.fhir.rest.api.RestOperationTypeEnum - The type of operation that the FHIR server has determined that the client is trying to invoke 351 * </li> 352 * </ul> 353 * </p> 354 * <p> 355 * Hook methods must return <code>void</code> 356 * </p> 357 * <p> 358 * Hook methods method may throw a subclass of {@link BaseServerResponseException}, and processing 359 * will be aborted with an appropriate error returned to the client. 360 * </p> 361 */ 362 SERVER_INCOMING_REQUEST_PRE_HANDLED( 363 void.class, 364 "ca.uhn.fhir.rest.api.server.RequestDetails", 365 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 366 "ca.uhn.fhir.rest.api.RestOperationTypeEnum"), 367 368 /** 369 * <b>Server Hook:</b> 370 * This method is called when a resource provider method is registered and being bound 371 * by the HAPI FHIR Plain Server / RestfulServer. 372 * <p> 373 * Hooks may accept the following parameters: 374 * <ul> 375 * <li> 376 * ca.uhn.fhir.rest.server.method.BaseMethodBinding - The method binding. 377 * </li> 378 * </ul> 379 * <p> 380 * Hook methods may modify the method binding, replace it, or return <code>null</code> to cancel the binding. 381 * </p> 382 */ 383 SERVER_PROVIDER_METHOD_BOUND( 384 "ca.uhn.fhir.rest.server.method.BaseMethodBinding", "ca.uhn.fhir.rest.server.method.BaseMethodBinding"), 385 386 /** 387 * <b>Server Hook:</b> 388 * This method is called upon any exception being thrown within the server's request processing code. This includes 389 * any exceptions thrown within resource provider methods (e.g. {@link Search} and {@link Read} methods) as well as 390 * any runtime exceptions thrown by the server itself. This hook method is invoked for each interceptor (until one of them 391 * returns a non-<code>null</code> response or the end of the list is reached), after which 392 * {@link #SERVER_HANDLE_EXCEPTION} is 393 * called for each interceptor. 394 * <p> 395 * This may be used to add an OperationOutcome to a response, or to convert between exception types for any reason. 396 * </p> 397 * <p> 398 * Implementations of this method may choose to ignore/log/count/etc exceptions, and return <code>null</code>. In 399 * this case, processing will continue, and the server will automatically generate an {@link BaseOperationOutcome 400 * OperationOutcome}. Implementations may also choose to provide their own response to the client. In this case, they 401 * should return a non-<code>null</code>, to indicate that they have handled the request and processing should stop. 402 * </p> 403 * <p> 404 * Hooks may accept the following parameters: 405 * <ul> 406 * <li> 407 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 408 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 409 * pulled out of the servlet request. Note that the bean 410 * properties are not all guaranteed to be populated, depending on how early during processing the 411 * exception occurred. 412 * </li> 413 * <li> 414 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 415 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 416 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 417 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 418 * </li> 419 * <li> 420 * java.lang.Throwable - The exception that was thrown. This will often be an instance of 421 * {@link BaseServerResponseException} but will not necessarily be one (e.g. it could be a 422 * {@link NullPointerException} in the case of a bug being triggered. 423 * </li> 424 * <li> 425 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 426 * </li> 427 * <li> 428 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 429 * </li> 430 * </ul> 431 * <p> 432 * Hook methods may return a new exception to use for processing, or <code>null</code> if this interceptor is not trying to 433 * modify the exception. For example, if this interceptor has nothing to do with exception processing, it 434 * should always return <code>null</code>. If this interceptor adds an OperationOutcome to the exception, it 435 * should return an exception. 436 * </p> 437 */ 438 SERVER_PRE_PROCESS_OUTGOING_EXCEPTION( 439 BaseServerResponseException.class, 440 "ca.uhn.fhir.rest.api.server.RequestDetails", 441 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 442 "java.lang.Throwable", 443 "jakarta.servlet.http.HttpServletRequest", 444 "jakarta.servlet.http.HttpServletResponse"), 445 446 /** 447 * <b>Server Hook:</b> 448 * This method is called after the server implementation method has been called, but before any attempt 449 * to stream the response back to the client. Interceptors may examine or modify the response before it 450 * is returned, or even prevent the response. 451 * <p> 452 * Hooks may accept the following parameters: 453 * <ul> 454 * <li> 455 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 456 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 457 * pulled out of the servlet request. 458 * </li> 459 * <li> 460 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 461 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 462 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 463 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 464 * </li> 465 * <li> 466 * org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be returned. This parameter may be <code>null</code> for some responses. 467 * </li> 468 * <li> 469 * ca.uhn.fhir.rest.api.server.ResponseDetails - This object contains details about the response, including the contents. Hook methods may modify this object to change or replace the response. 470 * </li> 471 * <li> 472 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 473 * </li> 474 * <li> 475 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 476 * </li> 477 * </ul> 478 * </p> 479 * <p> 480 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 481 * This is generally the right thing to do. If your interceptor is providing a response rather than 482 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 483 * no further processing will occur and no further interceptors will be called. 484 * </p> 485 * <p> 486 * Hook methods may also throw {@link AuthenticationException} to indicate that the interceptor 487 * has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 488 * will be returned to the client. 489 */ 490 SERVER_OUTGOING_RESPONSE( 491 boolean.class, 492 "ca.uhn.fhir.rest.api.server.RequestDetails", 493 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 494 "org.hl7.fhir.instance.model.api.IBaseResource", 495 "ca.uhn.fhir.rest.api.server.ResponseDetails", 496 "jakarta.servlet.http.HttpServletRequest", 497 "jakarta.servlet.http.HttpServletResponse"), 498 499 /** 500 * <b>Server Hook:</b> 501 * This method is called when a stream writer is generated that will be used to stream a non-binary response to 502 * a client. Hooks may return a wrapped writer which adds additional functionality as needed. 503 * 504 * <p> 505 * Hooks may accept the following parameters: 506 * <ul> 507 * <li> 508 * java.io.Writer - The response writing Writer. Typically a hook will wrap this writer and layer additional functionality 509 * into the wrapping writer. 510 * </li> 511 * <li> 512 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 513 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 514 * pulled out of the servlet request. 515 * </li> 516 * <li> 517 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 518 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 519 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 520 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 521 * </li> 522 * </ul> 523 * </p> 524 * <p> 525 * Hook methods should return a {@link Writer} instance that will be used to stream the response. Hook methods 526 * should not throw any exception. 527 * </p> 528 * 529 * @since 5.0.0 530 */ 531 SERVER_OUTGOING_WRITER_CREATED( 532 Writer.class, 533 "java.io.Writer", 534 "ca.uhn.fhir.rest.api.server.RequestDetails", 535 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 536 537 /** 538 * <b>Server Hook:</b> 539 * This method is called after the server implementation method has been called, but before any attempt 540 * to stream the response back to the client, specifically for GraphQL requests (as these do not fit 541 * cleanly into the model provided by {@link #SERVER_OUTGOING_RESPONSE}). 542 * <p> 543 * Hooks may accept the following parameters: 544 * <ul> 545 * <li> 546 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 547 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 548 * pulled out of the servlet request. 549 * </li> 550 * <li> 551 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 552 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 553 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 554 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 555 * </li> 556 * <li> 557 * java.lang.String - The GraphQL query 558 * </li> 559 * <li> 560 * java.lang.String - The GraphQL response 561 * </li> 562 * <li> 563 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 564 * </li> 565 * <li> 566 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 567 * </li> 568 * </ul> 569 * </p> 570 * <p> 571 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 572 * This is generally the right thing to do. If your interceptor is providing a response rather than 573 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 574 * no further processing will occur and no further interceptors will be called. 575 * </p> 576 * <p> 577 * Hook methods may also throw {@link AuthenticationException} to indicate that the interceptor 578 * has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 579 * will be returned to the client. 580 */ 581 SERVER_OUTGOING_GRAPHQL_RESPONSE( 582 boolean.class, 583 "ca.uhn.fhir.rest.api.server.RequestDetails", 584 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 585 "java.lang.String", 586 "java.lang.String", 587 "jakarta.servlet.http.HttpServletRequest", 588 "jakarta.servlet.http.HttpServletResponse"), 589 590 /** 591 * <b>Server Hook:</b> 592 * This method is called when an OperationOutcome is being returned in response to a failure. 593 * Hook methods may use this hook to modify the OperationOutcome being returned. 594 * <p> 595 * Hooks may accept the following parameters: 596 * <ul> 597 * <li> 598 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 599 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 600 * pulled out of the servlet request. Note that the bean 601 * properties are not all guaranteed to be populated, depending on how early during processing the 602 * exception occurred. 603 * </li> 604 * <li> 605 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 606 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 607 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 608 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 609 * </li> 610 * <li> 611 * org.hl7.fhir.instance.model.api.IBaseOperationOutcome - The OperationOutcome resource that will be 612 * returned. 613 * </ul> 614 * <p> 615 * Hook methods must return <code>void</code> 616 * </p> 617 */ 618 SERVER_OUTGOING_FAILURE_OPERATIONOUTCOME( 619 void.class, 620 "ca.uhn.fhir.rest.api.server.RequestDetails", 621 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 622 "org.hl7.fhir.instance.model.api.IBaseOperationOutcome"), 623 624 /** 625 * <b>Server Hook:</b> 626 * This method is called after all processing is completed for a request, but only if the 627 * request completes normally (i.e. no exception is thrown). 628 * <p> 629 * This pointcut is called after the response has completely finished, meaning that the HTTP response to the client 630 * may or may not have already completely been returned to the client by the time this pointcut is invoked. Use caution 631 * if you have timing-dependent logic, since there is no guarantee about whether the client will have already moved on 632 * by the time your method is invoked. If you need a guarantee that your method is invoked before returning to the 633 * client, consider using {@link #SERVER_OUTGOING_RESPONSE} instead. 634 * </p> 635 * <p> 636 * Hooks may accept the following parameters: 637 * <ul> 638 * <li> 639 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 640 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 641 * pulled out of the servlet request. 642 * </li> 643 * <li> 644 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 645 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 646 * pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment. 647 * </li> 648 * </ul> 649 * </p> 650 * <p> 651 * This method must return <code>void</code> 652 * </p> 653 * <p> 654 * This method should not throw any exceptions. Any exception that is thrown by this 655 * method will be logged, but otherwise not acted upon (i.e. even if a hook method 656 * throws an exception, processing will continue and other interceptors will be 657 * called). Therefore it is considered a bug to throw an exception from hook methods using this 658 * pointcut. 659 * </p> 660 */ 661 SERVER_PROCESSING_COMPLETED_NORMALLY( 662 void.class, 663 new ExceptionHandlingSpec().addLogAndSwallow(Throwable.class), 664 "ca.uhn.fhir.rest.api.server.RequestDetails", 665 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 666 667 /** 668 * <b>Server Hook:</b> 669 * This method is called after all processing is completed for a request, regardless of whether 670 * the request completed successfully or not. It is called after {@link #SERVER_PROCESSING_COMPLETED_NORMALLY} 671 * in the case of successful operations. 672 * <p> 673 * Hooks may accept the following parameters: 674 * <ul> 675 * <li> 676 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 677 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 678 * pulled out of the servlet request. 679 * </li> 680 * <li> 681 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 682 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 683 * pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment. 684 * </li> 685 * </ul> 686 * </p> 687 * <p> 688 * This method must return <code>void</code> 689 * </p> 690 * <p> 691 * This method should not throw any exceptions. Any exception that is thrown by this 692 * method will be logged, but otherwise not acted upon (i.e. even if a hook method 693 * throws an exception, processing will continue and other interceptors will be 694 * called). Therefore it is considered a bug to throw an exception from hook methods using this 695 * pointcut. 696 * </p> 697 */ 698 SERVER_PROCESSING_COMPLETED( 699 void.class, 700 new ExceptionHandlingSpec().addLogAndSwallow(Throwable.class), 701 "ca.uhn.fhir.rest.api.server.RequestDetails", 702 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 703 704 /** 705 * <b>Subscription Hook:</b> 706 * Invoked whenever a persisted resource has been modified and is being submitted to the 707 * subscription processing pipeline. This method is called before the resource is placed 708 * on any queues for processing and executes synchronously during the resource modification 709 * operation itself, so it should return quickly. 710 * <p> 711 * Hooks may accept the following parameters: 712 * <ul> 713 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 714 * </ul> 715 * </p> 716 * <p> 717 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 718 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 719 * returns <code>false</code>, subscription processing will not proceed for the given resource; 720 * </p> 721 */ 722 SUBSCRIPTION_RESOURCE_MODIFIED(boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 723 724 /** 725 * <b>Subscription Hook:</b> 726 * Invoked any time that a resource is matched by an individual subscription, and 727 * is about to be queued for delivery. 728 * <p> 729 * Hooks may make changes to the delivery payload, or make changes to the 730 * canonical subscription such as adding headers, modifying the channel 731 * endpoint, etc. 732 * </p> 733 * Hooks may accept the following parameters: 734 * <ul> 735 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 736 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 737 * <li>ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult</li> 738 * </ul> 739 * <p> 740 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 741 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 742 * returns <code>false</code>, delivery will be aborted. 743 * </p> 744 */ 745 SUBSCRIPTION_RESOURCE_MATCHED( 746 boolean.class, 747 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 748 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", 749 "ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult"), 750 751 /** 752 * <b>Subscription Hook:</b> 753 * Invoked whenever a persisted resource was checked against all active subscriptions, and did not 754 * match any. 755 * <p> 756 * Hooks may accept the following parameters: 757 * <ul> 758 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks should not modify this parameter as changes will not have any effect.</li> 759 * </ul> 760 * </p> 761 * <p> 762 * Hooks should return <code>void</code>. 763 * </p> 764 */ 765 SUBSCRIPTION_RESOURCE_DID_NOT_MATCH_ANY_SUBSCRIPTIONS( 766 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 767 768 /** 769 * <b>Subscription Hook:</b> 770 * Invoked immediately before the delivery of a subscription, and right before any channel-specific 771 * hooks are invoked (e.g. {@link #SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY}. 772 * <p> 773 * Hooks may make changes to the delivery payload, or make changes to the 774 * canonical subscription such as adding headers, modifying the channel 775 * endpoint, etc. 776 * </p> 777 * Hooks may accept the following parameters: 778 * <ul> 779 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 780 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 781 * </ul> 782 * <p> 783 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 784 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 785 * returns <code>false</code>, processing will be aborted. 786 * </p> 787 */ 788 SUBSCRIPTION_BEFORE_DELIVERY( 789 boolean.class, 790 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 791 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 792 793 /** 794 * <b>Subscription Hook:</b> 795 * Invoked immediately after the delivery of a subscription, and right before any channel-specific 796 * hooks are invoked (e.g. {@link #SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY}. 797 * <p> 798 * Hooks may accept the following parameters: 799 * </p> 800 * <ul> 801 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 802 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 803 * </ul> 804 * <p> 805 * Hooks should return <code>void</code>. 806 * </p> 807 */ 808 SUBSCRIPTION_AFTER_DELIVERY( 809 void.class, 810 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 811 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 812 813 /** 814 * <b>Subscription Hook:</b> 815 * Invoked immediately after the attempted delivery of a subscription, if the delivery 816 * failed. 817 * <p> 818 * Hooks may accept the following parameters: 819 * </p> 820 * <ul> 821 * <li>java.lang.Exception - The exception that caused the failure. Note this could be an exception thrown by a SUBSCRIPTION_BEFORE_DELIVERY or SUBSCRIPTION_AFTER_DELIVERY interceptor</li> 822 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage - the message that triggered the exception</li> 823 * <li>java.lang.Exception</li> 824 * </ul> 825 * <p> 826 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 827 * <code>void</code> or <code>true</code>, processing will continue normally, meaning that 828 * an exception will be thrown by the delivery mechanism. This typically means that the 829 * message will be returned to the processing queue. If the method 830 * returns <code>false</code>, processing will be aborted and no further action will be 831 * taken for the delivery. 832 * </p> 833 */ 834 SUBSCRIPTION_AFTER_DELIVERY_FAILED( 835 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", "java.lang.Exception"), 836 837 /** 838 * <b>Subscription Hook:</b> 839 * Invoked immediately after the delivery of a REST HOOK subscription. 840 * <p> 841 * When this hook is called, all processing is complete so this hook should not 842 * make any changes to the parameters. 843 * </p> 844 * Hooks may accept the following parameters: 845 * <ul> 846 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 847 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 848 * </ul> 849 * <p> 850 * Hooks should return <code>void</code>. 851 * </p> 852 */ 853 SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY( 854 void.class, 855 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 856 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 857 858 /** 859 * <b>Subscription Hook:</b> 860 * Invoked immediately before the delivery of a REST HOOK subscription. 861 * <p> 862 * Hooks may make changes to the delivery payload, or make changes to the 863 * canonical subscription such as adding headers, modifying the channel 864 * endpoint, etc. 865 * </p> 866 * Hooks may accept the following parameters: 867 * <ul> 868 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 869 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 870 * </ul> 871 * <p> 872 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 873 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 874 * returns <code>false</code>, processing will be aborted. 875 * </p> 876 */ 877 SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY( 878 boolean.class, 879 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 880 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 881 882 /** 883 * <b>Subscription Hook:</b> 884 * Invoked immediately after the delivery of MESSAGE subscription. 885 * <p> 886 * When this hook is called, all processing is complete so this hook should not 887 * make any changes to the parameters. 888 * </p> 889 * Hooks may accept the following parameters: 890 * <ul> 891 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 892 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 893 * </ul> 894 * <p> 895 * Hooks should return <code>void</code>. 896 * </p> 897 */ 898 SUBSCRIPTION_AFTER_MESSAGE_DELIVERY( 899 void.class, 900 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 901 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 902 903 /** 904 * <b>Subscription Hook:</b> 905 * Invoked immediately before the delivery of a MESSAGE subscription. 906 * <p> 907 * Hooks may make changes to the delivery payload, or make changes to the 908 * canonical subscription such as adding headers, modifying the channel 909 * endpoint, etc. 910 * Furthermore, you may modify the outgoing message wrapper, for example adding headers via ResourceModifiedJsonMessage field. 911 * </p> 912 * Hooks may accept the following parameters: 913 * <ul> 914 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 915 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 916 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage</li> 917 * 918 * </ul> 919 * <p> 920 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 921 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 922 * returns <code>false</code>, processing will be aborted. 923 * </p> 924 */ 925 SUBSCRIPTION_BEFORE_MESSAGE_DELIVERY( 926 boolean.class, 927 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 928 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", 929 "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage"), 930 931 /** 932 * <b>Subscription Hook:</b> 933 * Invoked whenever a persisted resource (a resource that has just been stored in the 934 * database via a create/update/patch/etc.) is about to be checked for whether any subscriptions 935 * were triggered as a result of the operation. 936 * <p> 937 * Hooks may accept the following parameters: 938 * <ul> 939 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 940 * </ul> 941 * </p> 942 * <p> 943 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 944 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 945 * returns <code>false</code>, processing will be aborted. 946 * </p> 947 */ 948 SUBSCRIPTION_BEFORE_PERSISTED_RESOURCE_CHECKED( 949 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 950 951 /** 952 * <b>Subscription Hook:</b> 953 * Invoked whenever a persisted resource (a resource that has just been stored in the 954 * database via a create/update/patch/etc.) has been checked for whether any subscriptions 955 * were triggered as a result of the operation. 956 * <p> 957 * Hooks may accept the following parameters: 958 * <ul> 959 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 960 * </ul> 961 * </p> 962 * <p> 963 * Hooks should return <code>void</code>. 964 * </p> 965 */ 966 SUBSCRIPTION_AFTER_PERSISTED_RESOURCE_CHECKED( 967 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 968 969 /** 970 * <b>Subscription Hook:</b> 971 * Invoked immediately after an active subscription is "registered". In HAPI FHIR, when 972 * a subscription 973 * <p> 974 * Hooks may make changes to the canonicalized subscription and this will have an effect 975 * on processing across this server. Note however that timing issues may occur, since the 976 * subscription is already technically live by the time this hook is called. 977 * </p> 978 * Hooks may accept the following parameters: 979 * <ul> 980 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 981 * </ul> 982 * <p> 983 * Hooks should return <code>void</code>. 984 * </p> 985 */ 986 SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_REGISTERED( 987 void.class, "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription"), 988 989 /** 990 * <b>Subscription Hook:</b> 991 * Invoked immediately after an active subscription is "registered". In HAPI FHIR, when 992 * a subscription 993 * <p> 994 * Hooks may make changes to the canonicalized subscription and this will have an effect 995 * on processing across this server. Note however that timing issues may occur, since the 996 * subscription is already technically live by the time this hook is called. 997 * </p> 998 * No parameters are currently supported. 999 * <p> 1000 * Hooks should return <code>void</code>. 1001 * </p> 1002 */ 1003 SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_UNREGISTERED(void.class), 1004 1005 /** 1006 * <b>Storage Hook:</b> 1007 * Invoked when a resource is being deleted in a cascaded delete. This means that 1008 * some other resource is being deleted, but per use request or other 1009 * policy, the given resource (the one supplied as a parameter to this hook) 1010 * is also being deleted. 1011 * <p> 1012 * Hooks may accept the following parameters: 1013 * </p> 1014 * <ul> 1015 * <li> 1016 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1017 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1018 * pulled out of the servlet request. Note that the bean 1019 * properties are not all guaranteed to be populated, depending on how early during processing the 1020 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1021 * known, such as while processing searches</b> 1022 * </li> 1023 * <li> 1024 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1025 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1026 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1027 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1028 * </li> 1029 * <li> 1030 * ca.uhn.fhir.jpa.util.DeleteConflictList - Contains the details about the delete conflicts that are 1031 * being resolved via deletion. The source resource is the resource that will be deleted, and 1032 * is a cascade because the target resource is already being deleted. 1033 * </li> 1034 * <li> 1035 * org.hl7.fhir.instance.model.api.IBaseResource - The actual resource that is about to be deleted via a cascading delete 1036 * </li> 1037 * </ul> 1038 * <p> 1039 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1040 * which case the delete should be rolled back. 1041 * </p> 1042 */ 1043 STORAGE_CASCADE_DELETE( 1044 void.class, 1045 "ca.uhn.fhir.rest.api.server.RequestDetails", 1046 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1047 "ca.uhn.fhir.jpa.api.model.DeleteConflictList", 1048 "org.hl7.fhir.instance.model.api.IBaseResource"), 1049 1050 /** 1051 * <b>Subscription Topic Hook:</b> 1052 * Invoked whenever a persisted resource (a resource that has just been stored in the 1053 * database via a create/update/patch/etc.) is about to be checked for whether any subscription topics 1054 * were triggered as a result of the operation. 1055 * <p> 1056 * Hooks may accept the following parameters: 1057 * <ul> 1058 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 1059 * </ul> 1060 * </p> 1061 * <p> 1062 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 1063 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 1064 * returns <code>false</code>, processing will be aborted. 1065 * </p> 1066 */ 1067 SUBSCRIPTION_TOPIC_BEFORE_PERSISTED_RESOURCE_CHECKED( 1068 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 1069 1070 /** 1071 * <b>Subscription Topic Hook:</b> 1072 * Invoked whenever a persisted resource (a resource that has just been stored in the 1073 * database via a create/update/patch/etc.) has been checked for whether any subscription topics 1074 * were triggered as a result of the operation. 1075 * <p> 1076 * Hooks may accept the following parameters: 1077 * <ul> 1078 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 1079 * </ul> 1080 * </p> 1081 * <p> 1082 * Hooks should return <code>void</code>. 1083 * </p> 1084 */ 1085 SUBSCRIPTION_TOPIC_AFTER_PERSISTED_RESOURCE_CHECKED( 1086 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 1087 1088 /** 1089 * <b>Storage Hook:</b> 1090 * Invoked when we are about to <a href="https://smilecdr.com/docs/fhir_repository/creating_data.html#auto-create-placeholder-reference-targets">Auto-Create a Placeholder Reference</a>. 1091 * Hooks may modify/enhance the placeholder reference target that is about to be created, or 1092 * reject the creation of the resource, which generally means that the transaction will be 1093 * rejected instead (because of the invalid reference). 1094 * <p> 1095 * Hooks may accept the following parameters: 1096 * </p> 1097 * <ul> 1098 * <li> 1099 * ca.uhn.fhir.storage.interceptor.AutoCreatePlaceholderReferenceTargetRequest - Contains details about the placeholder that is about to be created, including the source resource whose reference is being fulfilled, as well as the candidate placeholder resource that will be created. 1100 * </li> 1101 * <li> 1102 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1103 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1104 * pulled out of the servlet request. 1105 * </li> 1106 * <li> 1107 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1108 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1109 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1110 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1111 * </li> 1112 * </ul> 1113 * <p> 1114 * Hooks may return <code>void</code> (in which case the placeholder creation will proceed as normal), 1115 * an object of type 1116 * <code>ca.uhn.fhir.storage.interceptor.AutoCreatePlaceholderReferenceTargetResponse</code> 1117 * (in which case the response object can approve or reject the creation), 1118 * and can throw exceptions (which will trigger an appropriate error message being returned 1119 * to the client). 1120 * </p> 1121 */ 1122 STORAGE_PRE_AUTO_CREATE_PLACEHOLDER_REFERENCE( 1123 "ca.uhn.fhir.storage.interceptor.AutoCreatePlaceholderReferenceTargetResponse", 1124 "ca.uhn.fhir.storage.interceptor.AutoCreatePlaceholderReferenceTargetRequest", 1125 "ca.uhn.fhir.rest.api.server.RequestDetails", 1126 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1127 1128 /** 1129 * <b>Storage Hook:</b> 1130 * Invoked when a Bulk Export job is being kicked off, but before any permission checks 1131 * have been done. 1132 * This hook can be used to modify or update parameters as need be before 1133 * authorization/permission checks are done. 1134 * <p> 1135 * Hooks may accept the following parameters: 1136 * </p> 1137 * <ul> 1138 * <li> 1139 * ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off 1140 * </li> 1141 * <li> 1142 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1143 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1144 * pulled out of the servlet request. Note that the bean 1145 * properties are not all guaranteed to be populated, depending on how early during processing the 1146 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1147 * known, such as while processing searches</b> 1148 * </li> 1149 * <li> 1150 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1151 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1152 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1153 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1154 * </li> 1155 * </ul> 1156 * <p> 1157 * Hooks should return <code>void</code>, and can throw exceptions. 1158 * </p> 1159 */ 1160 STORAGE_PRE_INITIATE_BULK_EXPORT( 1161 void.class, 1162 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1163 "ca.uhn.fhir.rest.api.server.RequestDetails", 1164 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1165 1166 /** 1167 * <b>Storage Hook:</b> 1168 * Invoked when a Bulk Export job is being kicked off. Hook methods may modify 1169 * the request, or raise an exception to prevent it from being initiated. 1170 * This hook is not guaranteed to be called before permission checks, and so 1171 * anu implementers should be cautious of changing the options in ways that would 1172 * affect permissions. 1173 * <p> 1174 * Hooks may accept the following parameters: 1175 * </p> 1176 * <ul> 1177 * <li> 1178 * ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off 1179 * </li> 1180 * <li> 1181 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1182 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1183 * pulled out of the servlet request. Note that the bean 1184 * properties are not all guaranteed to be populated, depending on how early during processing the 1185 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1186 * known, such as while processing searches</b> 1187 * </li> 1188 * <li> 1189 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1190 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1191 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1192 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1193 * </li> 1194 * </ul> 1195 * <p> 1196 * Hooks should return <code>void</code>, and can throw exceptions. 1197 * </p> 1198 */ 1199 STORAGE_INITIATE_BULK_EXPORT( 1200 void.class, 1201 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1202 "ca.uhn.fhir.rest.api.server.RequestDetails", 1203 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1204 1205 /** 1206 * <b>Storage Hook:</b> 1207 * Invoked when a Bulk Export job is being processed. If any hook method is registered 1208 * for this pointcut, the hook method will be called once for each resource that is 1209 * loaded for inclusion in a bulk export file. Hook methods may modify 1210 * the resource object and this modification will affect the copy that is stored in the 1211 * bulk export data file (but will not affect the original). Hook methods may also 1212 * return <code>false</code> in order to request that the resource be filtered 1213 * from the export. 1214 * <p> 1215 * Hooks may accept the following parameters: 1216 * </p> 1217 * <ul> 1218 * <li> 1219 * ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters - The details of the job being kicked off 1220 * </li> 1221 * <li> 1222 *org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be included in the file 1223 * </li> 1224 * </ul> 1225 * <p> 1226 * Hooks methods may return <code>false</code> to indicate that the resource should be 1227 * filtered out. Otherwise, hook methods should return <code>true</code>. 1228 * </p> 1229 * 1230 * @since 6.8.0 1231 */ 1232 STORAGE_BULK_EXPORT_RESOURCE_INCLUSION( 1233 boolean.class, 1234 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1235 "org.hl7.fhir.instance.model.api.IBaseResource"), 1236 1237 /** 1238 * <b>Storage Hook:</b> 1239 * Invoked when a set of resources are about to be deleted and expunged via url like {@code http://localhost/Patient?active=false&_expunge=true}. 1240 * <p> 1241 * Hooks may accept the following parameters: 1242 * </p> 1243 * <ul> 1244 * <li> 1245 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1246 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1247 * pulled out of the servlet request. Note that the bean 1248 * properties are not all guaranteed to be populated, depending on how early during processing the 1249 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1250 * known, such as while processing searches</b> 1251 * </li> 1252 * <li> 1253 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1254 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1255 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1256 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1257 * </li> 1258 * <li> 1259 * java.lang.String - Contains the url used to delete and expunge the resources 1260 * </li> 1261 * </ul> 1262 * <p> 1263 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1264 * which case the delete expunge will not occur. 1265 * </p> 1266 */ 1267 STORAGE_PRE_DELETE_EXPUNGE( 1268 void.class, 1269 "ca.uhn.fhir.rest.api.server.RequestDetails", 1270 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1271 "java.lang.String"), 1272 1273 /** 1274 * <b>Storage Hook:</b> 1275 * Invoked when a batch of resource pids are about to be deleted and expunged via url like {@code http://localhost/Patient?active=false&_expunge=true}. 1276 * <p> 1277 * Hooks may accept the following parameters: 1278 * </p> 1279 * <ul> 1280 * <li> 1281 * java.lang.String - the name of the resource type being deleted 1282 * </li> 1283 * <li> 1284 * java.util.List - the list of Long pids of the resources about to be deleted 1285 * </li> 1286 * <li> 1287 * java.util.concurrent.atomic.AtomicLong - holds a running tally of all entities deleted so far. 1288 * If the pointcut callback deletes any entities, then this parameter should be incremented by the total number 1289 * of additional entities deleted. 1290 * </li> 1291 * <li> 1292 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1293 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1294 * pulled out of the servlet request. Note that the bean 1295 * properties are not all guaranteed to be populated, depending on how early during processing the 1296 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1297 * known, such as while processing searches</b> 1298 * </li> 1299 * <li> 1300 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1301 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1302 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1303 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1304 * </li> 1305 * <li> 1306 * java.lang.String - Contains the url used to delete and expunge the resources 1307 * </li> 1308 * </ul> 1309 * <p> 1310 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1311 * which case the delete expunge will not occur. 1312 * </p> 1313 */ 1314 STORAGE_PRE_DELETE_EXPUNGE_PID_LIST( 1315 void.class, 1316 "java.lang.String", 1317 "java.util.List", 1318 "java.util.concurrent.atomic.AtomicLong", 1319 "ca.uhn.fhir.rest.api.server.RequestDetails", 1320 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1321 1322 /** 1323 * <b>Storage Hook:</b> 1324 * Invoked when one or more resources may be returned to the user, whether as a part of a READ, 1325 * a SEARCH, or even as the response to a CREATE/UPDATE, etc. 1326 * <p> 1327 * This hook is invoked when a resource has been loaded by the storage engine and 1328 * is being returned to the HTTP stack for response. This is not a guarantee that the 1329 * client will ultimately see it, since filters/headers/etc may affect what 1330 * is returned but if a resource is loaded it is likely to be used. 1331 * Note also that caching may affect whether this pointcut is invoked. 1332 * </p> 1333 * <p> 1334 * Hooks will have access to the contents of the resource being returned 1335 * and may choose to make modifications. These changes will be reflected in 1336 * returned resource but have no effect on storage. 1337 * </p> 1338 * Hooks may accept the following parameters: 1339 * <ul> 1340 * <li> 1341 * ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails - Contains details about the 1342 * specific resources being returned. 1343 * </li> 1344 * <li> 1345 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1346 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1347 * pulled out of the servlet request. Note that the bean 1348 * properties are not all guaranteed to be populated, depending on how early during processing the 1349 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1350 * known, such as while processing searches</b> 1351 * </li> 1352 * <li> 1353 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1354 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1355 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1356 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1357 * </li> 1358 * </ul> 1359 * <p> 1360 * Hooks should return <code>void</code>. 1361 * </p> 1362 */ 1363 STORAGE_PREACCESS_RESOURCES( 1364 void.class, 1365 "ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails", 1366 "ca.uhn.fhir.rest.api.server.RequestDetails", 1367 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1368 1369 /** 1370 * <b>Storage Hook:</b> 1371 * Invoked when the storage engine is about to check for the existence of a pre-cached search 1372 * whose results match the given search parameters. 1373 * <p> 1374 * Hooks may accept the following parameters: 1375 * </p> 1376 * <ul> 1377 * <li> 1378 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked 1379 * </li> 1380 * <li> 1381 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1382 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1383 * pulled out of the servlet request. Note that the bean 1384 * properties are not all guaranteed to be populated, depending on how early during processing the 1385 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1386 * known, such as while processing searches</b> 1387 * </li> 1388 * <li> 1389 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1390 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1391 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1392 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1393 * </li> 1394 * </ul> 1395 * <p> 1396 * Hooks may return <code>boolean</code>. If the hook method returns 1397 * <code>false</code>, the server will not attempt to check for a cached 1398 * search no matter what. 1399 * </p> 1400 */ 1401 STORAGE_PRECHECK_FOR_CACHED_SEARCH( 1402 boolean.class, 1403 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 1404 "ca.uhn.fhir.rest.api.server.RequestDetails", 1405 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1406 1407 /** 1408 * <b>Storage Hook:</b> 1409 * Invoked when a search is starting, prior to creating a record for the search. 1410 * <p> 1411 * Hooks may accept the following parameters: 1412 * </p> 1413 * <ul> 1414 * <li> 1415 * ca.uhn.fhir.rest.server.util.ICachedSearchDetails - Contains the details of the search that 1416 * is being created and initialized. Interceptors may use this parameter to modify aspects of the search 1417 * before it is stored and executed. 1418 * </li> 1419 * <li> 1420 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1421 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1422 * pulled out of the servlet request. Note that the bean 1423 * properties are not all guaranteed to be populated, depending on how early during processing the 1424 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1425 * known, such as while processing searches</b> 1426 * </li> 1427 * <li> 1428 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1429 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1430 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1431 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1432 * </li> 1433 * <li> 1434 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked. This can be modified. 1435 * </li> 1436 * <li> 1437 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition associated with the request (or {@literal null} if the server is not partitioned) 1438 * </li> 1439 * </ul> 1440 * <p> 1441 * Hooks should return <code>void</code>. 1442 * </p> 1443 */ 1444 STORAGE_PRESEARCH_REGISTERED( 1445 void.class, 1446 "ca.uhn.fhir.rest.server.util.ICachedSearchDetails", 1447 "ca.uhn.fhir.rest.api.server.RequestDetails", 1448 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1449 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 1450 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 1451 1452 /** 1453 * <b>Storage Hook:</b> 1454 * Invoked when one or more resources may be returned to the user, whether as a part of a READ, 1455 * a SEARCH, or even as the response to a CREATE/UPDATE, etc. 1456 * <p> 1457 * This hook is invoked when a resource has been loaded by the storage engine and 1458 * is being returned to the HTTP stack for response. 1459 * This is not a guarantee that the 1460 * client will ultimately see it, since filters/headers/etc may affect what 1461 * is returned but if a resource is loaded it is likely to be used. 1462 * Note also that caching may affect whether this pointcut is invoked. 1463 * </p> 1464 * <p> 1465 * Hooks will have access to the contents of the resource being returned 1466 * and may choose to make modifications. These changes will be reflected in 1467 * returned resource but have no effect on storage. 1468 * </p> 1469 * Hooks may accept the following parameters: 1470 * <ul> 1471 * <li> 1472 * ca.uhn.fhir.rest.api.server.IPreResourceShowDetails - Contains the resources that 1473 * will be shown to the user. This object may be manipulated in order to modify 1474 * the actual resources being shown to the user (e.g. for masking) 1475 * </li> 1476 * <li> 1477 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1478 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1479 * pulled out of the servlet request. Note that the bean 1480 * properties are not all guaranteed to be populated, depending on how early during processing the 1481 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1482 * known, such as while processing searches</b> 1483 * </li> 1484 * <li> 1485 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1486 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1487 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1488 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1489 * </li> 1490 * </ul> 1491 * <p> 1492 * Hooks should return <code>void</code>. 1493 * </p> 1494 */ 1495 STORAGE_PRESHOW_RESOURCES( 1496 void.class, 1497 "ca.uhn.fhir.rest.api.server.IPreResourceShowDetails", 1498 "ca.uhn.fhir.rest.api.server.RequestDetails", 1499 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1500 1501 /** 1502 * <b>Storage Hook:</b> 1503 * Invoked before a resource will be created, immediately before the resource 1504 * is persisted to the database. 1505 * <p> 1506 * Hooks will have access to the contents of the resource being created 1507 * and may choose to make modifications to it. These changes will be 1508 * reflected in permanent storage. 1509 * </p> 1510 * Hooks may accept the following parameters: 1511 * <ul> 1512 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1513 * <li> 1514 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1515 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1516 * pulled out of the servlet request. Note that the bean 1517 * properties are not all guaranteed to be populated, depending on how early during processing the 1518 * exception occurred. 1519 * </li> 1520 * <li> 1521 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1522 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1523 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1524 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1525 * </li> 1526 * <li> 1527 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1528 * </li> 1529 * </ul> 1530 * <p> 1531 * Hooks should return <code>void</code>. 1532 * </p> 1533 */ 1534 STORAGE_PRESTORAGE_RESOURCE_CREATED( 1535 void.class, 1536 "org.hl7.fhir.instance.model.api.IBaseResource", 1537 "ca.uhn.fhir.rest.api.server.RequestDetails", 1538 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1539 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1540 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 1541 1542 /** 1543 * <b>Storage Hook:</b> 1544 * Invoked before client-assigned id is created. 1545 * <p> 1546 * Hooks will have access to the contents of the resource being created 1547 * so that client-assigned ids can be allowed/denied. These changes will 1548 * be reflected in permanent storage. 1549 * </p> 1550 * Hooks may accept the following parameters: 1551 * <ul> 1552 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1553 * <li> 1554 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1555 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1556 * pulled out of the servlet request. Note that the bean 1557 * properties are not all guaranteed to be populated, depending on how early during processing the 1558 * exception occurred. 1559 * </li> 1560 * </ul> 1561 * <p> 1562 * Hooks should return <code>void</code>. 1563 * </p> 1564 */ 1565 STORAGE_PRESTORAGE_CLIENT_ASSIGNED_ID( 1566 void.class, "org.hl7.fhir.instance.model.api.IBaseResource", "ca.uhn.fhir.rest.api.server.RequestDetails"), 1567 1568 /** 1569 * <b>Storage Hook:</b> 1570 * Invoked before a resource will be updated, immediately before the resource 1571 * is persisted to the database. 1572 * <p> 1573 * Hooks will have access to the contents of the resource being updated 1574 * (both the previous and new contents) and may choose to make modifications 1575 * to the new contents of the resource. These changes will be reflected in 1576 * permanent storage. 1577 * </p> 1578 * <p> 1579 * <b>NO-OPS:</b> If the client has submitted an update that does not actually make any changes 1580 * (i.e. the resource they include in the PUT body is identical to the content that 1581 * was already stored) the server may choose to ignore the update and perform 1582 * a "NO-OP". In this case, this pointcut is still invoked, but {@link #STORAGE_PRECOMMIT_RESOURCE_UPDATED} 1583 * will not be. Hook methods for this pointcut may make changes to the new contents of the 1584 * resource being updated, and in this case the NO-OP will be cancelled and 1585 * {@link #STORAGE_PRECOMMIT_RESOURCE_UPDATED} will also be invoked. 1586 * </p> 1587 * Hooks may accept the following parameters: 1588 * <ul> 1589 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource being updated</li> 1590 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The new contents of the resource being updated</li> 1591 * <li> 1592 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1593 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1594 * pulled out of the servlet request. Note that the bean 1595 * properties are not all guaranteed to be populated, depending on how early during processing the 1596 * exception occurred. 1597 * </li> 1598 * <li> 1599 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1600 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1601 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1602 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1603 * </li> 1604 * <li> 1605 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1606 * </li> 1607 * </ul> 1608 * <p> 1609 * Hooks should return <code>void</code>. 1610 * </p> 1611 */ 1612 STORAGE_PRESTORAGE_RESOURCE_UPDATED( 1613 void.class, 1614 "org.hl7.fhir.instance.model.api.IBaseResource", 1615 "org.hl7.fhir.instance.model.api.IBaseResource", 1616 "ca.uhn.fhir.rest.api.server.RequestDetails", 1617 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1618 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1619 1620 /** 1621 * <b>Storage Hook:</b> 1622 * Invoked before a resource will be deleted, immediately before the resource 1623 * is removed from the database. 1624 * <p> 1625 * Hooks will have access to the contents of the resource being deleted 1626 * and may choose to make modifications related to it. These changes will be 1627 * reflected in permanent storage. 1628 * </p> 1629 * Hooks may accept the following parameters: 1630 * <ul> 1631 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted</li> 1632 * <li> 1633 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1634 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1635 * pulled out of the servlet request. Note that the bean 1636 * properties are not all guaranteed to be populated, depending on how early during processing the 1637 * exception occurred. 1638 * </li> 1639 * <li> 1640 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1641 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1642 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1643 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1644 * </li> 1645 * <li> 1646 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1647 * </li> 1648 * </ul> 1649 * <p> 1650 * Hooks should return <code>void</code>. 1651 * </p> 1652 */ 1653 STORAGE_PRESTORAGE_RESOURCE_DELETED( 1654 void.class, 1655 "org.hl7.fhir.instance.model.api.IBaseResource", 1656 "ca.uhn.fhir.rest.api.server.RequestDetails", 1657 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1658 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1659 1660 /** 1661 * <b>Storage Hook:</b> 1662 * Invoked before a resource will be created, immediately before the transaction 1663 * is committed (after all validation and other business rules have successfully 1664 * completed, and any other database activity is complete. 1665 * <p> 1666 * Hooks will have access to the contents of the resource being created 1667 * but should generally not make any 1668 * changes as storage has already occurred. Changes will not be reflected 1669 * in storage, but may be reflected in the HTTP response. 1670 * </p> 1671 * Hooks may accept the following parameters: 1672 * <ul> 1673 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1674 * <li> 1675 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1676 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1677 * pulled out of the servlet request. Note that the bean 1678 * properties are not all guaranteed to be populated, depending on how early during processing the 1679 * exception occurred. 1680 * </li> 1681 * <li> 1682 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1683 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1684 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1685 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1686 * </li> 1687 * <li> 1688 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1689 * </li> 1690 * <li> 1691 * Boolean - Whether this pointcut invocation was deferred or not(since 5.4.0) 1692 * </li> 1693 * <li> 1694 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1695 * </li> 1696 * </ul> 1697 * <p> 1698 * Hooks should return <code>void</code>. 1699 * </p> 1700 */ 1701 STORAGE_PRECOMMIT_RESOURCE_CREATED( 1702 void.class, 1703 "org.hl7.fhir.instance.model.api.IBaseResource", 1704 "ca.uhn.fhir.rest.api.server.RequestDetails", 1705 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1706 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1707 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1708 1709 /** 1710 * <b>Storage Hook:</b> 1711 * Invoked before a resource will be updated, immediately before the transaction 1712 * is committed (after all validation and other business rules have successfully 1713 * completed, and any other database activity is complete. 1714 * <p> 1715 * Hooks will have access to the contents of the resource being updated 1716 * (both the previous and new contents) but should generally not make any 1717 * changes as storage has already occurred. Changes will not be reflected 1718 * in storage, but may be reflected in the HTTP response. 1719 * </p> 1720 * <p> 1721 * NO-OP note: See {@link #STORAGE_PRESTORAGE_RESOURCE_UPDATED} for a note on 1722 * no-op updates when no changes are detected. 1723 * </p> 1724 * Hooks may accept the following parameters: 1725 * <ul> 1726 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource</li> 1727 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The proposed new contents of the resource</li> 1728 * <li> 1729 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1730 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1731 * pulled out of the servlet request. Note that the bean 1732 * properties are not all guaranteed to be populated, depending on how early during processing the 1733 * exception occurred. 1734 * </li> 1735 * <li> 1736 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1737 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1738 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1739 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1740 * </li> 1741 * <li> 1742 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1743 * </li> 1744 * <li> 1745 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1746 * </li> 1747 * </ul> 1748 * <p> 1749 * Hooks should return <code>void</code>. 1750 * </p> 1751 */ 1752 STORAGE_PRECOMMIT_RESOURCE_UPDATED( 1753 void.class, 1754 "org.hl7.fhir.instance.model.api.IBaseResource", 1755 "org.hl7.fhir.instance.model.api.IBaseResource", 1756 "ca.uhn.fhir.rest.api.server.RequestDetails", 1757 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1758 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1759 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1760 1761 /** 1762 * <b>Storage Hook:</b> 1763 * Invoked before a resource will be deleted 1764 * <p> 1765 * Hooks will have access to the contents of the resource being deleted 1766 * but should not make any changes as storage has already occurred 1767 * </p> 1768 * Hooks may accept the following parameters: 1769 * <ul> 1770 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted</li> 1771 * <li> 1772 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1773 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1774 * pulled out of the servlet request. Note that the bean 1775 * properties are not all guaranteed to be populated, depending on how early during processing the 1776 * exception occurred. 1777 * </li> 1778 * <li> 1779 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1780 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1781 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1782 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1783 * </li> 1784 * <li> 1785 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1786 * </li> 1787 * <li> 1788 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1789 * </li> 1790 * </ul> 1791 * <p> 1792 * Hooks should return <code>void</code>. 1793 * </p> 1794 */ 1795 STORAGE_PRECOMMIT_RESOURCE_DELETED( 1796 void.class, 1797 "org.hl7.fhir.instance.model.api.IBaseResource", 1798 "ca.uhn.fhir.rest.api.server.RequestDetails", 1799 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1800 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1801 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1802 1803 /** 1804 * <b>Storage Hook:</b> 1805 * Invoked when a FHIR transaction bundle is about to begin processing. Hooks may choose to 1806 * modify the bundle, and may affect processing by doing so. 1807 * <p> 1808 * Hooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the 1809 * processing of the transaction bundle 1810 * </p> 1811 * Hooks may accept the following parameters: 1812 * <ul> 1813 * <li>org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle being processed</li> 1814 * <li> 1815 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1816 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1817 * pulled out of the servlet request. 1818 * </li> 1819 * <li> 1820 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1821 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1822 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1823 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1824 * </li> 1825 * </ul> 1826 * <p> 1827 * Hooks should return <code>void</code>. 1828 * </p> 1829 * 1830 * @see #STORAGE_TRANSACTION_PROCESSED 1831 * @since 6.2.0 1832 */ 1833 STORAGE_TRANSACTION_PROCESSING( 1834 void.class, 1835 "org.hl7.fhir.instance.model.api.IBaseBundle", 1836 "ca.uhn.fhir.rest.api.server.RequestDetails", 1837 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1838 1839 /** 1840 * <b>Storage Hook:</b> 1841 * Invoked after all entries in a transaction bundle have been executed 1842 * <p> 1843 * Hooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the 1844 * processing of the transaction bundle 1845 * </p> 1846 * Hooks may accept the following parameters: 1847 * <ul> 1848 * <li>org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle that wsa processed</li> 1849 * <li> 1850 * ca.uhn.fhir.rest.api.server.storage.DeferredInterceptorBroadcasts- A collection of pointcut invocations and their parameters which were deferred. 1851 * </li> 1852 * <li> 1853 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1854 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1855 * pulled out of the servlet request. 1856 * </li> 1857 * <li> 1858 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1859 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1860 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1861 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1862 * </li> 1863 * <li> 1864 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1865 * </li> 1866 * </ul> 1867 * <p> 1868 * Hooks should return <code>void</code>. 1869 * </p> 1870 * 1871 * @see #STORAGE_TRANSACTION_PROCESSING 1872 */ 1873 STORAGE_TRANSACTION_PROCESSED( 1874 void.class, 1875 "org.hl7.fhir.instance.model.api.IBaseBundle", 1876 "ca.uhn.fhir.rest.api.server.storage.DeferredInterceptorBroadcasts", 1877 "ca.uhn.fhir.rest.api.server.RequestDetails", 1878 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1879 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1880 1881 /** 1882 * <b>Storage Hook:</b> 1883 * Invoked during a FHIR transaction, immediately before processing all write operations (i.e. immediately 1884 * before a database transaction will be opened) 1885 * <p> 1886 * Hooks may accept the following parameters: 1887 * </p> 1888 * <ul> 1889 * <li> 1890 * ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start 1891 * </li> 1892 * <li> 1893 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1894 * </li> 1895 * </ul> 1896 * <p> 1897 * Hooks should return <code>void</code>. 1898 * </p> 1899 */ 1900 STORAGE_TRANSACTION_WRITE_OPERATIONS_PRE( 1901 void.class, 1902 "ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails", 1903 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1904 1905 /** 1906 * <b>Storage Hook:</b> 1907 * Invoked during a FHIR transaction, immediately after processing all write operations (i.e. immediately 1908 * after the transaction has been committed or rolled back). This hook will always be called if 1909 * {@link #STORAGE_TRANSACTION_WRITE_OPERATIONS_PRE} has been called, regardless of whether the operation 1910 * succeeded or failed. 1911 * <p> 1912 * Hooks may accept the following parameters: 1913 * </p> 1914 * <ul> 1915 * <li> 1916 * ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start 1917 * </li> 1918 * <li> 1919 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1920 * </li> 1921 * </ul> 1922 * <p> 1923 * Hooks should return <code>void</code>. 1924 * </p> 1925 */ 1926 STORAGE_TRANSACTION_WRITE_OPERATIONS_POST( 1927 void.class, 1928 "ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails", 1929 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1930 1931 /** 1932 * <b>Storage Hook:</b> 1933 * Invoked when a resource delete operation is about to fail due to referential integrity checks. Intended for use with {@literal ca.uhn.fhir.jpa.interceptor.CascadingDeleteInterceptor}. 1934 * <p> 1935 * Hooks will have access to the list of resources that have references to the resource being deleted. 1936 * </p> 1937 * Hooks may accept the following parameters: 1938 * <ul> 1939 * <li>ca.uhn.fhir.jpa.api.model.DeleteConflictList - The list of delete conflicts</li> 1940 * <li> 1941 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1942 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1943 * pulled out of the servlet request. Note that the bean 1944 * properties are not all guaranteed to be populated, depending on how early during processing the 1945 * exception occurred. 1946 * </li> 1947 * <li> 1948 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1949 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1950 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1951 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1952 * </li> 1953 * <li> 1954 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1955 * </li> 1956 * </ul> 1957 * <p> 1958 * Hooks should return <code>ca.uhn.fhir.jpa.delete.DeleteConflictOutcome</code>. 1959 * If the interceptor returns a non-null result, the DeleteConflictOutcome can be 1960 * used to indicate a number of times to retry. 1961 * </p> 1962 */ 1963 STORAGE_PRESTORAGE_DELETE_CONFLICTS( 1964 // Return type 1965 "ca.uhn.fhir.jpa.delete.DeleteConflictOutcome", 1966 // Params 1967 "ca.uhn.fhir.jpa.api.model.DeleteConflictList", 1968 "ca.uhn.fhir.rest.api.server.RequestDetails", 1969 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1970 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1971 1972 /** 1973 * <b>Storage Hook:</b> 1974 * Invoked before a resource is about to be expunged via the <code>$expunge</code> operation. 1975 * <p> 1976 * Hooks will be passed a reference to a counter containing the current number of records that have been deleted. 1977 * If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted. 1978 * </p> 1979 * <p> 1980 * Hooks may accept the following parameters: 1981 * </p> 1982 * <ul> 1983 * <li>java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.</li> 1984 * <li>org.hl7.fhir.instance.model.api.IIdType - The ID of the resource that is about to be deleted</li> 1985 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource that is about to be deleted</li> 1986 * <li> 1987 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1988 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1989 * pulled out of the servlet request. Note that the bean 1990 * properties are not all guaranteed to be populated, depending on how early during processing the 1991 * exception occurred. 1992 * </li> 1993 * <li> 1994 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1995 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1996 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1997 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1998 * </li> 1999 * </ul> 2000 * <p> 2001 * Hooks should return void. 2002 * </p> 2003 */ 2004 STORAGE_PRESTORAGE_EXPUNGE_RESOURCE( 2005 // Return type 2006 void.class, 2007 // Params 2008 "java.util.concurrent.atomic.AtomicInteger", 2009 "org.hl7.fhir.instance.model.api.IIdType", 2010 "org.hl7.fhir.instance.model.api.IBaseResource", 2011 "ca.uhn.fhir.rest.api.server.RequestDetails", 2012 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2013 2014 /** 2015 * <b>Storage Hook:</b> 2016 * Invoked before an <code>$expunge</code> operation on all data (expungeEverything) is called. 2017 * <p> 2018 * Hooks will be passed a reference to a counter containing the current number of records that have been deleted. 2019 * If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted. 2020 * </p> 2021 * Hooks may accept the following parameters: 2022 * <ul> 2023 * <li>java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.</li> 2024 * <li> 2025 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2026 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2027 * pulled out of the servlet request. Note that the bean 2028 * properties are not all guaranteed to be populated, depending on how early during processing the 2029 * exception occurred. 2030 * </li> 2031 * <li> 2032 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2033 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2034 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2035 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2036 * </li> 2037 * </ul> 2038 * <p> 2039 * Hooks should return void. 2040 * </p> 2041 */ 2042 STORAGE_PRESTORAGE_EXPUNGE_EVERYTHING( 2043 // Return type 2044 void.class, 2045 // Params 2046 "java.util.concurrent.atomic.AtomicInteger", 2047 "ca.uhn.fhir.rest.api.server.RequestDetails", 2048 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2049 2050 /** 2051 * <b>Storage Hook:</b> 2052 * Invoked before FHIR <b>create</b> operation to request the identification of the partition ID to be associated 2053 * with the resource being created. This hook will only be called if partitioning is enabled in the JPA 2054 * server. 2055 * <p> 2056 * Hooks may accept the following parameters: 2057 * </p> 2058 * <ul> 2059 * <li> 2060 * org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be created and needs a tenant ID assigned. 2061 * </li> 2062 * <li> 2063 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2064 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2065 * pulled out of the servlet request. Note that the bean 2066 * properties are not all guaranteed to be populated, depending on how early during processing the 2067 * exception occurred. 2068 * </li> 2069 * <li> 2070 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2071 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2072 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2073 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2074 * </li> 2075 * </ul> 2076 * <p> 2077 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2078 * </p> 2079 * 2080 * @see #STORAGE_PARTITION_IDENTIFY_ANY For an alternative that is not read/write specific 2081 */ 2082 STORAGE_PARTITION_IDENTIFY_CREATE( 2083 // Return type 2084 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2085 // Params 2086 "org.hl7.fhir.instance.model.api.IBaseResource", 2087 "ca.uhn.fhir.rest.api.server.RequestDetails", 2088 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2089 2090 /** 2091 * <b>Storage Hook:</b> 2092 * Invoked before any FHIR read/access/extended operation (e.g. <b>read/vread</b>, <b>search</b>, <b>history</b>, 2093 * <b>$reindex</b>, etc.) operation to request the identification of the partition ID to be associated with 2094 * the resource(s) being searched for, read, etc. Essentially any operations in the JPA server that are not 2095 * creating a resource will use this pointcut. Creates will use {@link #STORAGE_PARTITION_IDENTIFY_CREATE}. 2096 * 2097 * <p> 2098 * This hook will only be called if 2099 * partitioning is enabled in the JPA server. 2100 * </p> 2101 * <p> 2102 * Hooks may accept the following parameters: 2103 * </p> 2104 * <ul> 2105 * <li> 2106 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2107 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2108 * pulled out of the servlet request. Note that the bean 2109 * properties are not all guaranteed to be populated, depending on how early during processing the 2110 * exception occurred. 2111 * </li> 2112 * <li> 2113 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2114 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2115 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2116 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2117 * </li> 2118 * <li>ca.uhn.fhir.interceptor.model.ReadPartitionIdRequestDetails - Contains details about what is being read</li> 2119 * </ul> 2120 * <p> 2121 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2122 * </p> 2123 * 2124 * @see #STORAGE_PARTITION_IDENTIFY_ANY For an alternative that is not read/write specific 2125 */ 2126 STORAGE_PARTITION_IDENTIFY_READ( 2127 // Return type 2128 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2129 // Params 2130 "ca.uhn.fhir.rest.api.server.RequestDetails", 2131 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2132 "ca.uhn.fhir.interceptor.model.ReadPartitionIdRequestDetails"), 2133 2134 /** 2135 * <b>Storage Hook:</b> 2136 * Invoked before FHIR operations to request the identification of the partition ID to be associated with the 2137 * request being made. 2138 * <p> 2139 * This hook is an alternative to {@link #STORAGE_PARTITION_IDENTIFY_READ} and {@link #STORAGE_PARTITION_IDENTIFY_CREATE} 2140 * and can be used in cases where a partition interceptor does not need knowledge of the specific resources being 2141 * accessed/read/written in order to determine the appropriate partition. 2142 * If registered, then neither STORAGE_PARTITION_IDENTIFY_READ, nor STORAGE_PARTITION_IDENTIFY_CREATE will be called. 2143 * </p> 2144 * <p> 2145 * This hook will only be called if 2146 * partitioning is enabled in the JPA server. 2147 * </p> 2148 * <p> 2149 * Hooks may accept the following parameters: 2150 * </p> 2151 * <ul> 2152 * <li> 2153 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2154 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2155 * pulled out of the servlet request. Note that the bean 2156 * properties are not all guaranteed to be populated, depending on how early during processing the 2157 * exception occurred. 2158 * </li> 2159 * <li> 2160 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2161 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2162 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2163 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2164 * </li> 2165 * </ul> 2166 * <p> 2167 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2168 * </p> 2169 * 2170 * @see #STORAGE_PARTITION_IDENTIFY_READ 2171 * @see #STORAGE_PARTITION_IDENTIFY_CREATE 2172 */ 2173 STORAGE_PARTITION_IDENTIFY_ANY( 2174 // Return type 2175 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2176 // Params 2177 "ca.uhn.fhir.rest.api.server.RequestDetails", 2178 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2179 2180 /** 2181 * <b>Storage Hook:</b> 2182 * Invoked when a partition has been created, typically meaning the <code>$partition-management-create-partition</code> 2183 * operation has been invoked. 2184 * <p> 2185 * This hook will only be called if 2186 * partitioning is enabled in the JPA server. 2187 * </p> 2188 * <p> 2189 * Hooks may accept the following parameters: 2190 * </p> 2191 * <ul> 2192 * <li> 2193 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected 2194 * </li> 2195 * <li> 2196 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2197 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2198 * pulled out of the servlet request. Note that the bean 2199 * properties are not all guaranteed to be populated, depending on how early during processing the 2200 * exception occurred. 2201 * </li> 2202 * <li> 2203 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2204 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2205 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2206 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2207 * </li> 2208 * </ul> 2209 * <p> 2210 * Hooks must return void. 2211 * </p> 2212 */ 2213 STORAGE_PARTITION_CREATED( 2214 // Return type 2215 void.class, 2216 // Params 2217 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2218 "ca.uhn.fhir.rest.api.server.RequestDetails", 2219 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2220 2221 /** 2222 * <b>Storage Hook:</b> 2223 * Invoked when a partition has been deleted, typically meaning the <code>$partition-management-delete-partition</code> 2224 * operation has been invoked. 2225 * <p> 2226 * This hook will only be called if 2227 * partitioning is enabled in the JPA server. 2228 * </p> 2229 * <p> 2230 * Hooks may accept the following parameters: 2231 * </p> 2232 * <ul> 2233 * <li> 2234 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The ID of the partition that was deleted. 2235 * </li> 2236 * </ul> 2237 * <p> 2238 * Hooks must return void. 2239 * </p> 2240 */ 2241 STORAGE_PARTITION_DELETED( 2242 // Return type 2243 void.class, 2244 // Params 2245 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 2246 2247 /** 2248 * <b>Storage Hook:</b> 2249 * Invoked before any partition aware FHIR operation, when the selected partition has been identified (ie. after the 2250 * {@link #STORAGE_PARTITION_IDENTIFY_CREATE} or {@link #STORAGE_PARTITION_IDENTIFY_READ} hook was called. This allows 2251 * a separate hook to register, and potentially make decisions about whether the request should be allowed to proceed. 2252 * <p> 2253 * This hook will only be called if 2254 * partitioning is enabled in the JPA server. 2255 * </p> 2256 * <p> 2257 * Hooks may accept the following parameters: 2258 * </p> 2259 * <ul> 2260 * <li> 2261 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected 2262 * </li> 2263 * <li> 2264 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2265 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2266 * pulled out of the servlet request. Note that the bean 2267 * properties are not all guaranteed to be populated, depending on how early during processing the 2268 * exception occurred. 2269 * </li> 2270 * <li> 2271 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2272 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2273 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2274 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2275 * </li> 2276 * <li> 2277 * ca.uhn.fhir.context.RuntimeResourceDefinition - The resource type being accessed, or {@literal null} if no specific type is associated with the request. 2278 * </li> 2279 * </ul> 2280 * <p> 2281 * Hooks must return void. 2282 * </p> 2283 */ 2284 STORAGE_PARTITION_SELECTED( 2285 // Return type 2286 void.class, 2287 // Params 2288 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2289 "ca.uhn.fhir.rest.api.server.RequestDetails", 2290 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2291 "ca.uhn.fhir.context.RuntimeResourceDefinition"), 2292 2293 /** 2294 * <b>Storage Hook:</b> 2295 * Invoked when a transaction has been rolled back as a result of a {@link ca.uhn.fhir.rest.server.exceptions.ResourceVersionConflictException}, 2296 * meaning that a database constraint has been violated. This pointcut allows an interceptor to specify a resolution strategy 2297 * other than simply returning the error to the client. This interceptor will be fired after the database transaction rollback 2298 * has been completed. 2299 * <p> 2300 * Hooks may accept the following parameters: 2301 * </p> 2302 * <ul> 2303 * <li> 2304 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2305 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2306 * pulled out of the servlet request. Note that the bean 2307 * properties are not all guaranteed to be populated, depending on how early during processing the 2308 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 2309 * known, such as while processing searches</b> 2310 * </li> 2311 * <li> 2312 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2313 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2314 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2315 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2316 * </li> 2317 * </ul> 2318 * <p> 2319 * Hooks should return <code>ca.uhn.fhir.jpa.api.model.ResourceVersionConflictResolutionStrategy</code>. Hooks should not 2320 * throw any exception. 2321 * </p> 2322 */ 2323 STORAGE_VERSION_CONFLICT( 2324 "ca.uhn.fhir.jpa.api.model.ResourceVersionConflictResolutionStrategy", 2325 "ca.uhn.fhir.rest.api.server.RequestDetails", 2326 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2327 2328 /** 2329 * <b>Validation Hook:</b> 2330 * This hook is called after validation has completed, regardless of whether the validation was successful or failed. 2331 * Typically this is used to modify validation results. 2332 * <p> 2333 * <b>Note on validation Pointcuts:</b> The HAPI FHIR interceptor framework is a part of the client and server frameworks and 2334 * not a part of the core FhirContext. Therefore this Pointcut is invoked by the 2335 * </p> 2336 * <p> 2337 * Hooks may accept the following parameters: 2338 * <ul> 2339 * <li> 2340 * org.hl7.fhir.instance.model.api.IBaseResource - The resource being validated, if a parsed version is available (null otherwise) 2341 * </li> 2342 * <li> 2343 * java.lang.String - The resource being validated, if a raw version is available (null otherwise) 2344 * </li> 2345 * <li> 2346 * ca.uhn.fhir.validation.ValidationResult - The outcome of the validation. Hooks methods should not modify this object, but they can return a new one. 2347 * </li> 2348 * </ul> 2349 * </p> 2350 * Hook methods may return an instance of {@link ca.uhn.fhir.validation.ValidationResult} if they wish to override the validation results, or they may return <code>null</code> or <code>void</code> otherwise. 2351 */ 2352 VALIDATION_COMPLETED( 2353 ValidationResult.class, 2354 "org.hl7.fhir.instance.model.api.IBaseResource", 2355 "java.lang.String", 2356 "ca.uhn.fhir.validation.ValidationResult"), 2357 2358 /** 2359 * <b>MDM(EMPI) Hook:</b> 2360 * Invoked when a persisted resource (a resource that has just been stored in the 2361 * database via a create/update/patch/etc.) enters the MDM module. The purpose of the pointcut is to permit a pseudo 2362 * modification of the resource elements to influence the MDM linking process. Any modifications to the resource are not persisted. 2363 * <p> 2364 * Hooks may accept the following parameters: 2365 * <ul> 2366 * <li>org.hl7.fhir.instance.model.api.IBaseResource - </li> 2367 * </ul> 2368 * </p> 2369 * <p> 2370 * Hooks should return <code>void</code>. 2371 * </p> 2372 */ 2373 MDM_BEFORE_PERSISTED_RESOURCE_CHECKED(void.class, "org.hl7.fhir.instance.model.api.IBaseResource"), 2374 2375 /** 2376 * <b>MDM(EMPI) Hook:</b> 2377 * Invoked whenever a persisted resource (a resource that has just been stored in the 2378 * database via a create/update/patch/etc.) has been matched against related resources and MDM links have been updated. 2379 * <p> 2380 * Hooks may accept the following parameters: 2381 * <ul> 2382 * <li>ca.uhn.fhir.rest.server.messaging.ResourceOperationMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 2383 * <li>ca.uhn.fhir.rest.server.TransactionLogMessages - This parameter is for informational messages provided by the MDM module during MDM processing.</li> 2384 * <li>ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - Contains information about the change event, including target and golden resource IDs and the operation type.</li> 2385 * </ul> 2386 * </p> 2387 * <p> 2388 * Hooks should return <code>void</code>. 2389 * </p> 2390 */ 2391 MDM_AFTER_PERSISTED_RESOURCE_CHECKED( 2392 void.class, 2393 "ca.uhn.fhir.rest.server.messaging.ResourceOperationMessage", 2394 "ca.uhn.fhir.rest.server.TransactionLogMessages", 2395 "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2396 2397 /** 2398 * <b>MDM Create Link</b> 2399 * This hook is invoked after an MDM link is created, 2400 * and changes have been persisted to the database. 2401 * <p> 2402 * Hook may accept the following parameters: 2403 * </p> 2404 * <ul> 2405 * <li> 2406 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2407 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2408 * pulled out of the servlet request. 2409 * </li> 2410 * <li> 2411 * ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type. 2412 * </li> 2413 * </ul> 2414 * <p> 2415 * Hooks should return <code>void</code>. 2416 * </p> 2417 */ 2418 MDM_POST_CREATE_LINK( 2419 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2420 2421 /** 2422 * <b>MDM Update Link</b> 2423 * This hook is invoked after an MDM link is updated, 2424 * and changes have been persisted to the database. 2425 * <p> 2426 * Hook may accept the following parameters: 2427 * </p> 2428 * <ul> 2429 * <li> 2430 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2431 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2432 * pulled out of the servlet request. 2433 * </li> 2434 * <li> 2435 * ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type. 2436 * </li> 2437 * </ul> 2438 * <p> 2439 * Hooks should return <code>void</code>. 2440 * </p> 2441 */ 2442 MDM_POST_UPDATE_LINK( 2443 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2444 2445 /** 2446 * <b>MDM Merge Golden Resources</b> 2447 * This hook is invoked after 2 golden resources have been 2448 * merged together and results persisted. 2449 * <p> 2450 * Hook may accept the following parameters: 2451 * </p> 2452 * <ul> 2453 * <li> 2454 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2455 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2456 * pulled out of the servlet request. 2457 * </li> 2458 * <li> 2459 * ca.uhn.fhir.mdm.model.mdmevents.MdmMergeEvent - Contains information about the from and to resources. 2460 * </li> 2461 * <li> 2462 * ca.uhn.fhir.mdm.model.mdmevents.MdmTransactionContext - Contains information about the Transaction context, e.g. merge or link. 2463 * </li> 2464 * </ul> 2465 * <p> 2466 * Hooks should return <code>void</code>. 2467 * </p> 2468 */ 2469 MDM_POST_MERGE_GOLDEN_RESOURCES( 2470 void.class, 2471 "ca.uhn.fhir.rest.api.server.RequestDetails", 2472 "ca.uhn.fhir.mdm.model.mdmevents.MdmMergeEvent", 2473 "ca.uhn.fhir.mdm.model.MdmTransactionContext"), 2474 2475 /** 2476 * <b>MDM Link History Hook:</b> 2477 * This hook is invoked after link histories are queried, 2478 * but before the results are returned to the caller. 2479 * <p> 2480 * Hook may accept the following parameters: 2481 * </p> 2482 * <ul> 2483 * <li> 2484 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2485 * </li> 2486 * <li> 2487 * ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent - An MDM History Event containing 2488 * information about the requested golden resource ids and/or source ids input, and 2489 * the returned link histories. 2490 * </li> 2491 * </ul> 2492 */ 2493 MDM_POST_LINK_HISTORY( 2494 void.class, 2495 "ca.uhn.fhir.rest.api.server.RequestDetails", 2496 "ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent"), 2497 2498 /** 2499 * <b>MDM Not Duplicate/Unduplicate Hook:</b> 2500 * This hook is invoked after 2 golden resources with an existing link 2501 * of "POSSIBLE_DUPLICATE" get unlinked/unduplicated. 2502 * <p> 2503 * This hook accepts the following parameters: 2504 * </p> 2505 * <ul> 2506 * <li> 2507 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2508 * </li> 2509 * <li> 2510 * ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - the resulting final link 2511 * between the 2 golden resources; now a NO_MATCH link. 2512 * </li> 2513 * </ul> 2514 */ 2515 MDM_POST_NOT_DUPLICATE( 2516 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2517 2518 /** 2519 * <b>MDM Clear Hook:</b> 2520 * This hook is invoked when an mdm clear operation is requested. 2521 * <p> 2522 * This hook accepts the following parameters: 2523 * </p> 2524 * <ul> 2525 * <li> 2526 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2527 * </li> 2528 * <li> 2529 * ca.uhn.fhir.mdm.model.mdmevents.MdmClearEvent - the event containing information on the clear command, 2530 * including the type filter (if any) and the batch size (if any). 2531 * </li> 2532 * </ul> 2533 */ 2534 MDM_CLEAR( 2535 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmClearEvent"), 2536 2537 /** 2538 * <b>MDM Submit Hook:</b> 2539 * This hook is invoked whenever when mdm submit operation is requested. 2540 * MDM submits can be invoked in multiple ways. 2541 * Some of which accept asynchronous calling, and some of which do not. 2542 * <p> 2543 * If the MDM Submit operation is asynchronous 2544 * (typically because the Prefer: respond-async header has been provided) 2545 * this hook will be invoked after the job is submitted, but before it has 2546 * necessarily been executed. 2547 * </p> 2548 * <p> 2549 * If the MDM Submit operation is synchronous, 2550 * this hook will be invoked immediately after the submit operation 2551 * has been executed, but before the call is returned to the caller. 2552 * </p> 2553 * <ul> 2554 * <li> 2555 * On Patient Type. Can be synchronous or asynchronous. 2556 * </li> 2557 * <li> 2558 * On Practitioner Type. Can be synchronous or asynchronous. 2559 * </li> 2560 * <li> 2561 * On specific patient instances. Is always synchronous. 2562 * </li> 2563 * <li> 2564 * On specific practitioner instances. Is always synchronous. 2565 * </li> 2566 * <li> 2567 * On the server (ie, not on any resource) with or without a resource filter. 2568 * Can be synchronous or asynchronous. 2569 * </li> 2570 * </ul> 2571 * <p> 2572 * In all cases, this hook will take the following parameters: 2573 * </p> 2574 * <ul> 2575 * <li> 2576 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2577 * </li> 2578 * <li> 2579 * ca.uhn.fhir.mdm.model.mdmevents.MdmSubmitEvent - An event with the Mdm Submit information 2580 * (urls specifying paths that will be searched for MDM submit, as well as 2581 * if this was an asynchronous request or not). 2582 * </li> 2583 * </ul> 2584 */ 2585 MDM_SUBMIT( 2586 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmSubmitEvent"), 2587 2588 /** 2589 * <b>MDM_SUBMIT_PRE_MESSAGE_DELIVERY Hook:</b> 2590 * Invoked immediately before the delivery of a MESSAGE to the broker. 2591 * <p> 2592 * Hooks can make changes to the delivery payload. 2593 * Furthermore, modification can be made to the outgoing message, 2594 * for example adding headers or changing message key, 2595 * which will be used for the subsequent processing. 2596 * </p> 2597 * Hooks should accept the following parameters: 2598 * <ul> 2599 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage</li> 2600 * </ul> 2601 */ 2602 MDM_SUBMIT_PRE_MESSAGE_DELIVERY(void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage"), 2603 2604 /** 2605 * <b>JPA Hook:</b> 2606 * This hook is invoked when a cross-partition reference is about to be 2607 * stored in the database. 2608 * <p> 2609 * <b>This is an experimental API - It may change in the future, use with caution.</b> 2610 * </p> 2611 * <p> 2612 * Hooks may accept the following parameters: 2613 * </p> 2614 * <ul> 2615 * <li> 2616 * {@literal ca.uhn.fhir.jpa.searchparam.extractor.CrossPartitionReferenceDetails} - Contains details about the 2617 * cross partition reference. 2618 * </li> 2619 * </ul> 2620 * <p> 2621 * Hooks should return <code>void</code>. 2622 * </p> 2623 */ 2624 JPA_RESOLVE_CROSS_PARTITION_REFERENCE( 2625 "ca.uhn.fhir.jpa.model.cross.IResourceLookup", 2626 "ca.uhn.fhir.jpa.searchparam.extractor.CrossPartitionReferenceDetails"), 2627 2628 /** 2629 * <b>Performance Tracing Hook:</b> 2630 * This hook is invoked when any informational messages generated by the 2631 * SearchCoordinator are created. It is typically used to provide logging 2632 * or capture details related to a specific request. 2633 * <p> 2634 * Note that this is a performance tracing hook. Use with caution in production 2635 * systems, since calling it may (or may not) carry a cost. 2636 * </p> 2637 * Hooks may accept the following parameters: 2638 * <ul> 2639 * <li> 2640 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2641 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2642 * pulled out of the servlet request. Note that the bean 2643 * properties are not all guaranteed to be populated, depending on how early during processing the 2644 * exception occurred. 2645 * </li> 2646 * <li> 2647 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2648 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2649 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2650 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2651 * </li> 2652 * <li> 2653 * ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message 2654 * </li> 2655 * </ul> 2656 * <p> 2657 * Hooks should return <code>void</code>. 2658 * </p> 2659 */ 2660 JPA_PERFTRACE_INFO( 2661 void.class, 2662 "ca.uhn.fhir.rest.api.server.RequestDetails", 2663 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2664 "ca.uhn.fhir.jpa.model.search.StorageProcessingMessage"), 2665 2666 /** 2667 * <b>Performance Tracing Hook:</b> 2668 * This hook is invoked when any warning messages generated by the 2669 * SearchCoordinator are created. It is typically used to provide logging 2670 * or capture details related to a specific request. 2671 * <p> 2672 * Note that this is a performance tracing hook. Use with caution in production 2673 * systems, since calling it may (or may not) carry a cost. 2674 * </p> 2675 * Hooks may accept the following parameters: 2676 * <ul> 2677 * <li> 2678 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2679 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2680 * pulled out of the servlet request. Note that the bean 2681 * properties are not all guaranteed to be populated, depending on how early during processing the 2682 * exception occurred. 2683 * </li> 2684 * <li> 2685 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2686 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2687 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2688 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2689 * </li> 2690 * <li> 2691 * ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message 2692 * </li> 2693 * </ul> 2694 * <p> 2695 * Hooks should return <code>void</code>. 2696 * </p> 2697 */ 2698 JPA_PERFTRACE_WARNING( 2699 void.class, 2700 "ca.uhn.fhir.rest.api.server.RequestDetails", 2701 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2702 "ca.uhn.fhir.jpa.model.search.StorageProcessingMessage"), 2703 2704 /** 2705 * <b>Performance Tracing Hook:</b> 2706 * This hook is invoked when a search has returned the very first result 2707 * from the database. The timing on this call can be a good indicator of how 2708 * performant a query is in general. 2709 * <p> 2710 * Note that this is a performance tracing hook. Use with caution in production 2711 * systems, since calling it may (or may not) carry a cost. 2712 * </p> 2713 * Hooks may accept the following parameters: 2714 * <ul> 2715 * <li> 2716 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2717 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2718 * pulled out of the servlet request. Note that the bean 2719 * properties are not all guaranteed to be populated, depending on how early during processing the 2720 * exception occurred. 2721 * </li> 2722 * <li> 2723 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2724 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2725 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2726 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2727 * </li> 2728 * <li> 2729 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2730 * performed. Hooks should not modify this object. 2731 * </li> 2732 * </ul> 2733 * <p> 2734 * Hooks should return <code>void</code>. 2735 * </p> 2736 */ 2737 JPA_PERFTRACE_SEARCH_FIRST_RESULT_LOADED( 2738 void.class, 2739 "ca.uhn.fhir.rest.api.server.RequestDetails", 2740 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2741 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2742 2743 /** 2744 * <b>Performance Tracing Hook:</b> 2745 * This hook is invoked when an individual search query SQL SELECT statement 2746 * has completed and no more results are available from that query. Note that this 2747 * doesn't necessarily mean that no more matching results exist in the database, 2748 * since HAPI FHIR JPA batch loads results in to the query cache in chunks in order 2749 * to provide predicable results without overloading memory or the database. 2750 * <p> 2751 * Note that this is a performance tracing hook. Use with caution in production 2752 * systems, since calling it may (or may not) carry a cost. 2753 * </p> 2754 * Hooks may accept the following parameters: 2755 * <ul> 2756 * <li> 2757 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2758 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2759 * pulled out of the servlet request. Note that the bean 2760 * properties are not all guaranteed to be populated, depending on how early during processing the 2761 * exception occurred. 2762 * </li> 2763 * <li> 2764 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2765 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2766 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2767 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2768 * </li> 2769 * <li> 2770 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2771 * performed. Hooks should not modify this object. 2772 * </li> 2773 * </ul> 2774 * <p> 2775 * Hooks should return <code>void</code>. 2776 * </p> 2777 */ 2778 JPA_PERFTRACE_SEARCH_SELECT_COMPLETE( 2779 void.class, 2780 "ca.uhn.fhir.rest.api.server.RequestDetails", 2781 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2782 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2783 2784 /** 2785 * <b>Performance Tracing Hook:</b> 2786 * This hook is invoked when a search has failed for any reason. When this pointcut 2787 * is invoked, the search has completed unsuccessfully and will not be continued. 2788 * <p> 2789 * Note that this is a performance tracing hook. Use with caution in production 2790 * systems, since calling it may (or may not) carry a cost. 2791 * </p> 2792 * Hooks may accept the following parameters: 2793 * <ul> 2794 * <li> 2795 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2796 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2797 * pulled out of the servlet request. Note that the bean 2798 * properties are not all guaranteed to be populated, depending on how early during processing the 2799 * exception occurred. 2800 * </li> 2801 * <li> 2802 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2803 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2804 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2805 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2806 * </li> 2807 * <li> 2808 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2809 * performed. Hooks should not modify this object. 2810 * </li> 2811 * </ul> 2812 * <p> 2813 * Hooks should return <code>void</code>. 2814 * </p> 2815 */ 2816 JPA_PERFTRACE_SEARCH_FAILED( 2817 void.class, 2818 "ca.uhn.fhir.rest.api.server.RequestDetails", 2819 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2820 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2821 2822 /** 2823 * <b>Performance Tracing Hook:</b> 2824 * This hook is invoked when a search has completed. When this pointcut 2825 * is invoked, a pass in the Search Coordinator has completed successfully, but 2826 * not all possible resources have been loaded yet so a future paging request 2827 * may trigger a new task that will load further resources. 2828 * <p> 2829 * Note that this is a performance tracing hook. Use with caution in production 2830 * systems, since calling it may (or may not) carry a cost. 2831 * </p> 2832 * Hooks may accept the following parameters: 2833 * <ul> 2834 * <li> 2835 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2836 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2837 * pulled out of the servlet request. Note that the bean 2838 * properties are not all guaranteed to be populated, depending on how early during processing the 2839 * exception occurred. 2840 * </li> 2841 * <li> 2842 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2843 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2844 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2845 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2846 * </li> 2847 * <li> 2848 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2849 * performed. Hooks should not modify this object. 2850 * </li> 2851 * </ul> 2852 * <p> 2853 * Hooks should return <code>void</code>. 2854 * </p> 2855 */ 2856 JPA_PERFTRACE_SEARCH_PASS_COMPLETE( 2857 void.class, 2858 "ca.uhn.fhir.rest.api.server.RequestDetails", 2859 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2860 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2861 2862 /** 2863 * <b>Performance Tracing Hook:</b> 2864 * This hook is invoked when a query involving an external index (e.g. Elasticsearch) has completed. When this pointcut 2865 * is invoked, an initial list of resource IDs has been generated which will be used as part of a subsequent database query. 2866 * <p> 2867 * Note that this is a performance tracing hook. Use with caution in production 2868 * systems, since calling it may (or may not) carry a cost. 2869 * </p> 2870 * Hooks may accept the following parameters: 2871 * <ul> 2872 * <li> 2873 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2874 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2875 * pulled out of the servlet request. Note that the bean 2876 * properties are not all guaranteed to be populated, depending on how early during processing the 2877 * exception occurred. 2878 * </li> 2879 * <li> 2880 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2881 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2882 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2883 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2884 * </li> 2885 * <li> 2886 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2887 * performed. Hooks should not modify this object. 2888 * </li> 2889 * </ul> 2890 * <p> 2891 * Hooks should return <code>void</code>. 2892 * </p> 2893 */ 2894 JPA_PERFTRACE_INDEXSEARCH_QUERY_COMPLETE( 2895 void.class, 2896 "ca.uhn.fhir.rest.api.server.RequestDetails", 2897 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2898 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2899 2900 /** 2901 * <b>Performance Tracing Hook:</b> 2902 * Invoked when the storage engine is about to reuse the results of 2903 * a previously cached search. 2904 * <p> 2905 * Note that this is a performance tracing hook. Use with caution in production 2906 * systems, since calling it may (or may not) carry a cost. 2907 * </p> 2908 * <p> 2909 * Hooks may accept the following parameters: 2910 * </p> 2911 * <ul> 2912 * <li> 2913 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked 2914 * </li> 2915 * <li> 2916 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2917 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2918 * pulled out of the servlet request. Note that the bean 2919 * properties are not all guaranteed to be populated, depending on how early during processing the 2920 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 2921 * known, such as while processing searches</b> 2922 * </li> 2923 * <li> 2924 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2925 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2926 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2927 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2928 * </li> 2929 * </ul> 2930 * <p> 2931 * Hooks should return <code>void</code>. 2932 * </p> 2933 */ 2934 JPA_PERFTRACE_SEARCH_REUSING_CACHED( 2935 boolean.class, 2936 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 2937 "ca.uhn.fhir.rest.api.server.RequestDetails", 2938 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2939 2940 /** 2941 * <b>Performance Tracing Hook:</b> 2942 * This hook is invoked when a search has failed for any reason. When this pointcut 2943 * is invoked, a pass in the Search Coordinator has completed successfully, and all 2944 * possible results have been fetched and loaded into the query cache. 2945 * <p> 2946 * Note that this is a performance tracing hook. Use with caution in production 2947 * systems, since calling it may (or may not) carry a cost. 2948 * </p> 2949 * Hooks may accept the following parameters: 2950 * <ul> 2951 * <li> 2952 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2953 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2954 * pulled out of the servlet request. Note that the bean 2955 * properties are not all guaranteed to be populated, depending on how early during processing the 2956 * exception occurred. 2957 * </li> 2958 * <li> 2959 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2960 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2961 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2962 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2963 * </li> 2964 * <li> 2965 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2966 * performed. Hooks should not modify this object. 2967 * </li> 2968 * </ul> 2969 * <p> 2970 * Hooks should return <code>void</code>. 2971 * </p> 2972 */ 2973 JPA_PERFTRACE_SEARCH_COMPLETE( 2974 void.class, 2975 "ca.uhn.fhir.rest.api.server.RequestDetails", 2976 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2977 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2978 2979 /** 2980 * <b>Performance Tracing Hook:</b> 2981 * <p> 2982 * This hook is invoked when a search has found an individual ID. 2983 * </p> 2984 * <p> 2985 * THIS IS AN EXPERIMENTAL HOOK AND MAY BE REMOVED OR CHANGED WITHOUT WARNING. 2986 * </p> 2987 * <p> 2988 * Note that this is a performance tracing hook. Use with caution in production 2989 * systems, since calling it may (or may not) carry a cost. 2990 * </p> 2991 * <p> 2992 * Hooks may accept the following parameters: 2993 * </p> 2994 * <ul> 2995 * <li> 2996 * java.lang.Integer - The query ID 2997 * </li> 2998 * <li> 2999 * java.lang.Object - The ID 3000 * </li> 3001 * </ul> 3002 * <p> 3003 * Hooks should return <code>void</code>. 3004 * </p> 3005 */ 3006 JPA_PERFTRACE_SEARCH_FOUND_ID(void.class, "java.lang.Integer", "java.lang.Object"), 3007 3008 /** 3009 * <b>Performance Tracing Hook:</b> 3010 * This hook is invoked when a query has executed, and includes the raw SQL 3011 * statements that were executed against the database. 3012 * <p> 3013 * Note that this is a performance tracing hook. Use with caution in production 3014 * systems, since calling it may (or may not) carry a cost. 3015 * </p> 3016 * <p> 3017 * Hooks may accept the following parameters: 3018 * </p> 3019 * <ul> 3020 * <li> 3021 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3022 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3023 * pulled out of the servlet request. Note that the bean 3024 * properties are not all guaranteed to be populated, depending on how early during processing the 3025 * exception occurred. 3026 * </li> 3027 * <li> 3028 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3029 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3030 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 3031 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 3032 * </li> 3033 * <li> 3034 * ca.uhn.fhir.jpa.util.SqlQueryList - Contains details about the raw SQL queries. 3035 * </li> 3036 * </ul> 3037 * <p> 3038 * Hooks should return <code>void</code>. 3039 * </p> 3040 */ 3041 JPA_PERFTRACE_RAW_SQL( 3042 void.class, 3043 "ca.uhn.fhir.rest.api.server.RequestDetails", 3044 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 3045 "ca.uhn.fhir.jpa.util.SqlQueryList"), 3046 3047 /** 3048 * <b> Deprecated but still supported. Will eventually be removed. <code>Please use Pointcut.STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX</code> </b> 3049 * <b> Binary Blob Prefix Assigning Hook:</b> 3050 * <p> 3051 * Immediately before a binary blob is stored to its eventual data sink, this hook is called. 3052 * This hook allows implementers to provide a prefix to the binary blob's ID. 3053 * This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used. 3054 * <ul> 3055 * <li> 3056 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3057 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3058 * pulled out of the servlet request. Note that the bean 3059 * properties are not all guaranteed to be populated. 3060 * </li> 3061 * <li> 3062 * org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored. 3063 * </li> 3064 * </ul> 3065 * <p> 3066 * Hooks should return <code>String</code>, which represents the full prefix to be applied to the blob. 3067 * </p> 3068 */ 3069 @Deprecated(since = "7.2.0 - Use STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX instead.") 3070 STORAGE_BINARY_ASSIGN_BLOB_ID_PREFIX( 3071 String.class, 3072 "ca.uhn.fhir.rest.api.server.RequestDetails", 3073 "org.hl7.fhir.instance.model.api.IBaseResource"), 3074 3075 /** 3076 * <b> Binary Content Prefix Assigning Hook:</b> 3077 * <p> 3078 * Immediately before binary content is stored to its eventual data sink, this hook is called. 3079 * This hook allows implementers to provide a prefix to the binary content's ID. 3080 * This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used. 3081 * <ul> 3082 * <li> 3083 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3084 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3085 * pulled out of the servlet request. Note that the bean 3086 * properties are not all guaranteed to be populated. 3087 * </li> 3088 * <li> 3089 * org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored. 3090 * </li> 3091 * </ul> 3092 * <p> 3093 * Hooks should return <code>String</code>, which represents the full prefix to be applied to the blob. 3094 * </p> 3095 */ 3096 STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX( 3097 String.class, 3098 "ca.uhn.fhir.rest.api.server.RequestDetails", 3099 "org.hl7.fhir.instance.model.api.IBaseResource"), 3100 3101 /** 3102 * <b>Storage Hook:</b> 3103 * Invoked before a batch job is persisted to the database. 3104 * <p> 3105 * Hooks will have access to the content of the job being created 3106 * and may choose to make modifications to it. These changes will be 3107 * reflected in permanent storage. 3108 * </p> 3109 * Hooks may accept the following parameters: 3110 * <ul> 3111 * <li> 3112 * ca.uhn.fhir.batch2.model.JobInstance 3113 * </li> 3114 * <li> 3115 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that lead to the creation 3116 * of the jobInstance. 3117 * </li> 3118 * </ul> 3119 * <p> 3120 * Hooks should return <code>void</code>. 3121 * </p> 3122 */ 3123 STORAGE_PRESTORAGE_BATCH_JOB_CREATE( 3124 void.class, "ca.uhn.fhir.batch2.model.JobInstance", "ca.uhn.fhir.rest.api.server.RequestDetails"), 3125 3126 /** 3127 * <b>CDS Hooks Prefetch Hook:</b> 3128 * Invoked before a CDS Hooks prefetch request is made. 3129 * Hooks may accept the following parameters: 3130 * <ul> 3131 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query, template and resolution strategy used for the request. 3132 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3133 * invocations of a prefetch request/response.</li> 3134 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3135 * </ul> 3136 * 3137 * <p> 3138 * Hooks should return <code>void</code>. 3139 * </p> 3140 */ 3141 CDS_HOOK_PREFETCH_REQUEST( 3142 void.class, 3143 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3144 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson"), 3145 3146 /** 3147 * <b>CDS Hooks Prefetch Hook:</b> 3148 * Invoked after CDS Hooks prefetch request is completed successfully. 3149 * Hooks may accept the following parameters: 3150 * <ul> 3151 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request. 3152 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3153 * invocations of a prefetch request/response.</li> 3154 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3155 * <li> "org.hl7.fhir.instance.model.api.IBaseResource" - The resource that is returned by the prefetch 3156 * request</li> 3157 * </ul> 3158 * 3159 * <p> 3160 * Hooks should return <code>void</code>. 3161 * </p> 3162 */ 3163 CDS_HOOK_PREFETCH_RESPONSE( 3164 void.class, 3165 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3166 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson", 3167 "org.hl7.fhir.instance.model.api.IBaseResource"), 3168 3169 /** 3170 * <b>CDS Hooks Prefetch Hook:</b> 3171 * Invoked after a failed CDS Hooks prefetch request. 3172 * Hooks may accept the following parameters: 3173 * <ul> 3174 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request. 3175 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3176 * invocations of a prefetch request/response.</li> 3177 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3178 * <li> "java.lang.Exception" - The exception that caused the failure of the prefetch request</li> 3179 * </ul> 3180 * 3181 * <p> 3182 * Hooks should return <code>void</code>. 3183 * </p> 3184 */ 3185 CDS_HOOK_PREFETCH_FAILED( 3186 void.class, 3187 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3188 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson", 3189 "java.lang.Exception"), 3190 3191 /** 3192 * <b>Batch2 Hook:</b> 3193 * <p>This is a filter hook that can be used around workchunk processing. 3194 * It is expected that implementers return an <code>IInterceptorFilterHook</code> that invokes the supplier 3195 * and includes the logic that should be executed:</p> 3196 * <ol> 3197 * <li>Before a workchunk has been processed</li> 3198 * <li>If an error occurs during processing</li> 3199 * <li>After the workchunk has been processed</li> 3200 * </ol> 3201 * <p>Parameters:</p> 3202 * <ul> 3203 * <li>ca.uhn.fhir.batch2.model.JobInstance - The job instance</li> 3204 * <li>ca.uhn.fhir.batch2.model.WorkChunk - The work chunk</li> 3205 * </ul> 3206 * <p>Hooks should return an {@link ca.uhn.fhir.interceptor.api.IBaseInterceptorBroadcaster.IInterceptorFilterHook}</p> 3207 * <p>For more details see <a href="http://hapifhir.io/hapi-fhir/docs/interceptors/filter_hook_interceptors.html">Filter Hook Interceptors</a></p> 3208 */ 3209 BATCH2_CHUNK_PROCESS_FILTER( 3210 IInterceptorFilterHook.class, "ca.uhn.fhir.batch2.model.JobInstance", "ca.uhn.fhir.batch2.model.WorkChunk"), 3211 /** 3212 * This pointcut is used only for unit tests. Do not use in production code as it may be changed or 3213 * removed at any time. 3214 */ 3215 TEST_RB( 3216 boolean.class, 3217 new ExceptionHandlingSpec().addLogAndSwallow(IllegalStateException.class), 3218 String.class.getName(), 3219 String.class.getName()), 3220 3221 /** 3222 * This pointcut is used only for unit tests. Do not use in production code as it may be changed or 3223 * removed at any time. 3224 */ 3225 TEST_FILTER(IInterceptorFilterHook.class, String.class.getName()), 3226 3227 /** 3228 * This pointcut is used only for unit tests. Do not use in production code as it may be changed or 3229 * removed at any time. 3230 */ 3231 TEST_RO(BaseServerResponseException.class, String.class.getName(), String.class.getName()); 3232 3233 private final List<String> myParameterTypes; 3234 private final Class<?> myReturnType; 3235 private final ExceptionHandlingSpec myExceptionHandlingSpec; 3236 3237 Pointcut(@Nonnull String theReturnType, String... theParameterTypes) { 3238 this(toReturnTypeClass(theReturnType), new ExceptionHandlingSpec(), theParameterTypes); 3239 } 3240 3241 Pointcut( 3242 @Nonnull Class<?> theReturnType, 3243 @Nonnull ExceptionHandlingSpec theExceptionHandlingSpec, 3244 String... theParameterTypes) { 3245 3246 // This enum uses the lowercase-b boolean type to indicate boolean return pointcuts 3247 Validate.isTrue(!theReturnType.equals(Boolean.class), "Return type Boolean not allowed here, must be boolean"); 3248 3249 myReturnType = theReturnType; 3250 myExceptionHandlingSpec = theExceptionHandlingSpec; 3251 myParameterTypes = Collections.unmodifiableList(Arrays.asList(theParameterTypes)); 3252 } 3253 3254 Pointcut(@Nonnull Class<?> theReturnType, String... theParameterTypes) { 3255 this(theReturnType, new ExceptionHandlingSpec(), theParameterTypes); 3256 } 3257 3258 @Override 3259 public boolean isShouldLogAndSwallowException(@Nonnull Throwable theException) { 3260 for (Class<? extends Throwable> next : myExceptionHandlingSpec.myTypesToLogAndSwallow) { 3261 if (next.isAssignableFrom(theException.getClass())) { 3262 return true; 3263 } 3264 } 3265 return false; 3266 } 3267 3268 @Override 3269 @Nonnull 3270 public Class<?> getReturnType() { 3271 return myReturnType; 3272 } 3273 3274 @Override 3275 public Class<?> getBooleanReturnTypeForEnum() { 3276 return boolean.class; 3277 } 3278 3279 @Override 3280 @Nonnull 3281 public List<String> getParameterTypes() { 3282 return myParameterTypes; 3283 } 3284 3285 private static class UnknownType {} 3286 3287 private static class ExceptionHandlingSpec { 3288 3289 private final Set<Class<? extends Throwable>> myTypesToLogAndSwallow = new HashSet<>(); 3290 3291 ExceptionHandlingSpec addLogAndSwallow(@Nonnull Class<? extends Throwable> theType) { 3292 myTypesToLogAndSwallow.add(theType); 3293 return this; 3294 } 3295 } 3296 3297 private static Class<?> toReturnTypeClass(String theReturnType) { 3298 try { 3299 return Class.forName(theReturnType); 3300 } catch (ClassNotFoundException theE) { 3301 return UnknownType.class; 3302 } 3303 } 3304}