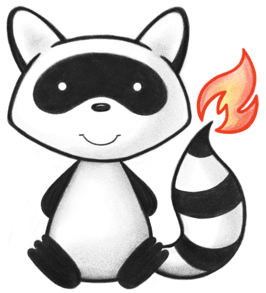
Package ca.uhn.fhir.util
Class BundleUtil
java.lang.Object
ca.uhn.fhir.util.BundleUtil
Fetch resources from a bundle
-
Field Summary
Fields -
Method Summary
Modifier and TypeMethodDescriptionstatic <T extends IBaseBundle>
TconvertBundleIntoTransaction
(FhirContext theContext, T theBundle, String thePrefixIdsOrNull) Converts a Bundle containing resources into a FHIR transaction which creates/updates the resources.static IBase
createNewBundleEntryWithSingleField
(FhirContext theContext, String theFieldName, IBase... theValues) create a new bundle entry and set a value for a single fieldstatic List
<org.apache.commons.lang3.tuple.Pair<String, IBaseResource>> getBundleEntryFullUrlsAndResources
(FhirContext theContext, IBaseBundle theBundle) Returns a collection of Pairs, one for each entry in the bundle.static List
<org.apache.commons.lang3.tuple.Pair<String, IBaseResource>> getBundleEntryUrlsAndResources
(FhirContext theContext, IBaseBundle theBundle) static String
getBundleType
(FhirContext theContext, IBaseBundle theBundle) static BundleTypeEnum
getBundleTypeEnum
(FhirContext theContext, IBaseBundle theBundle) static String
getLinkUrlOfType
(FhirContext theContext, IBaseBundle theBundle, String theLinkRelation) static IBase
getReferenceInBundle
(FhirContext theFhirContext, String theUrl, Object theAppContext) static IBaseResource
getResourceByReferenceAndResourceType
(FhirContext theContext, IBaseBundle theBundle, IBaseReference theReference) Get resource from bundle by resource type and referencestatic List
<SearchBundleEntryParts> getSearchBundleEntryParts
(FhirContext theContext, IBaseBundle theBundle) static Integer
getTotal
(FhirContext theContext, IBaseBundle theBundle) static boolean
isDstu3TransactionPatch
(FhirContext theContext, IBaseResource thePayloadResource) DSTU3 did not allow the PATCH verb for transaction bundles- so instead we infer that a bundle is a patch if the payload is a binary resource containing a patch.static void
processEntries
(FhirContext theContext, IBaseBundle theBundle, Consumer<ModifiableBundleEntry> theProcessor) Given a bundle, and a consumer, apply the consumer to each entry in the bundle.static void
setBundleType
(FhirContext theContext, IBaseBundle theBundle, String theType) static void
setTotal
(FhirContext theContext, IBaseBundle theBundle, Integer theTotal) static void
sortEntriesIntoProcessingOrder
(FhirContext theContext, IBaseBundle theBundle) Function which will do an in-place sort of a bundles' entries, to the correct processing order, which is: 1.static List
<BundleEntryParts> toListOfEntries
(FhirContext theContext, IBaseBundle theBundle) Extract all of the resources from a given bundletoListOfResourceIds
(FhirContext theContext, IBaseBundle theBundle) Extract all of ids of all the resources from a given bundlestatic List
<IBaseResource> toListOfResources
(FhirContext theContext, IBaseBundle theBundle) Extract all of the resources from a given bundlestatic <T extends IBaseResource>
List<T> toListOfResourcesOfType
(FhirContext theContext, IBaseBundle theBundle, Class<T> theTypeToInclude) Extract all of the resources of a given type from a given bundle
-
Field Details
-
DIFFERENT_LINK_ERROR_MSG
- See Also:
-
-
Method Details
-
getLinkUrlOfType
public static String getLinkUrlOfType(FhirContext theContext, IBaseBundle theBundle, String theLinkRelation) - Returns:
- Returns
null
if the link isn't found or has no value
-
getBundleEntryFullUrlsAndResources
public static List<org.apache.commons.lang3.tuple.Pair<String,IBaseResource>> getBundleEntryFullUrlsAndResources(FhirContext theContext, IBaseBundle theBundle) Returns a collection of Pairs, one for each entry in the bundle. Each pair will contain the values of Bundle.entry.fullUrl, and Bundle.entry.resource respectively. Nulls are possible in either or both values in the Pair.- Since:
- 7.0.0
-
getBundleEntryUrlsAndResources
public static List<org.apache.commons.lang3.tuple.Pair<String,IBaseResource>> getBundleEntryUrlsAndResources(FhirContext theContext, IBaseBundle theBundle) -
getBundleType
-
getBundleTypeEnum
-
setBundleType
-
getTotal
-
setTotal
-
toListOfEntries
Extract all of the resources from a given bundle -
sortEntriesIntoProcessingOrder
public static void sortEntriesIntoProcessingOrder(FhirContext theContext, IBaseBundle theBundle) throws IllegalStateException Function which will do an in-place sort of a bundles' entries, to the correct processing order, which is: 1. Deletes 2. Creates 3. UpdatesFurthermore, within these operation types, the entries will be sorted based on the order in which they should be processed e.g. if you have 2 CREATEs, one for a Patient, and one for an Observation which has this Patient as its Subject, the patient will come first, then the observation.
In cases of there being a cyclic dependency (e.g. Organization/1 is partOf Organization/2 and Organization/2 is partOf Organization/1) this function will throw an IllegalStateException.
- Parameters:
theContext
- The FhirContext.theBundle
- TheIBaseBundle
which contains the entries you would like sorted into processing order.- Throws:
IllegalStateException
-
convertBundleIntoTransaction
public static <T extends IBaseBundle> T convertBundleIntoTransaction(@Nonnull FhirContext theContext, @Nonnull T theBundle, @Nullable String thePrefixIdsOrNull) Converts a Bundle containing resources into a FHIR transaction which creates/updates the resources. This method does not modify the original bundle, but returns a new copy.This method is mostly intended for test scenarios where you have a Bundle containing search results or other sourced resources, and want to upload these resources to a server using a single FHIR transaction.
The Bundle is converted using the following logic:
- Bundle.type is changed to
transaction
- Bundle.request.method is changed to
PUT
- Bundle.request.url is changed to
[resourceType]/[id]
- Bundle.fullUrl is changed to
[resourceType]/[id]
- Parameters:
theContext
- The FhirContext to use with the bundletheBundle
- The Bundle to modify. All resources in the Bundle should have an ID.thePrefixIdsOrNull
- If notnull
, all resource IDs and all references in the Bundle will be modified to such that their IDs contain the given prefix. For example, for a value of "A", the resource "Patient/123" will be changed to be "Patient/A123". If set tonull
, resource IDs are unchanged.- Since:
- 7.4.0
- Bundle.type is changed to
-
getSearchBundleEntryParts
public static List<SearchBundleEntryParts> getSearchBundleEntryParts(FhirContext theContext, IBaseBundle theBundle) -
processEntries
public static void processEntries(FhirContext theContext, IBaseBundle theBundle, Consumer<ModifiableBundleEntry> theProcessor) Given a bundle, and a consumer, apply the consumer to each entry in the bundle.- Parameters:
theContext
- The FHIR ContexttheBundle
- The bundle to have its entries processed.theProcessor
- aConsumer
which will operate on all the entries of a bundle.
-
toListOfResources
Extract all of the resources from a given bundle -
toListOfResourceIds
Extract all of ids of all the resources from a given bundle -
toListOfResourcesOfType
public static <T extends IBaseResource> List<T> toListOfResourcesOfType(FhirContext theContext, IBaseBundle theBundle, Class<T> theTypeToInclude) Extract all of the resources of a given type from a given bundle -
getReferenceInBundle
public static IBase getReferenceInBundle(@Nonnull FhirContext theFhirContext, @Nonnull String theUrl, @Nullable Object theAppContext) -
isDstu3TransactionPatch
public static boolean isDstu3TransactionPatch(FhirContext theContext, IBaseResource thePayloadResource) DSTU3 did not allow the PATCH verb for transaction bundles- so instead we infer that a bundle is a patch if the payload is a binary resource containing a patch. This method tests whether a resource (which should have come fromBundle.entry.resource
is a Binary resource with a patch payload type. -
createNewBundleEntryWithSingleField
public static IBase createNewBundleEntryWithSingleField(FhirContext theContext, String theFieldName, IBase... theValues) create a new bundle entry and set a value for a single field- Parameters:
theContext
- Context holding resource definitiontheFieldName
- Child field name of the bundle entry to settheValues
- The values to set on the bundle entry child field name- Returns:
- the new bundle entry
-
getResourceByReferenceAndResourceType
@Nonnull public static IBaseResource getResourceByReferenceAndResourceType(@Nonnull FhirContext theContext, @Nonnull IBaseBundle theBundle, @Nonnull IBaseReference theReference) Get resource from bundle by resource type and reference- Parameters:
theContext
- FhirContexttheBundle
- IBaseBundletheReference
- IBaseReference- Returns:
- IBaseResource if found and null if not found.
-