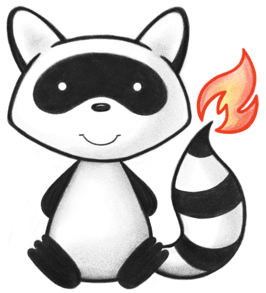
Package ca.uhn.fhir.util
Class ExtensionUtil
java.lang.Object
ca.uhn.fhir.util.ExtensionUtil
Utility for modifying with extensions in a FHIR version-independent approach.
-
Method Summary
Modifier and TypeMethodDescriptionstatic void
addExtension
(FhirContext theFhirContext, IBase theBase, String theUrl, String theValueType, Object theValue) Adds an extension with the specified valuestatic IBaseExtension
<?, ?> addExtension
(IBase theBase) Returns an new empty extension.static IBaseExtension
<?, ?> addExtension
(IBase theBase, String theUrl) Returns an extension with the specified URLstatic List
<IBaseExtension<?, ?>> clearAllExtensions
(IBase theBase) Removes all extensions.static List
<IBaseExtension<?, ?>> clearExtensionsByUrl
(IBase theBase, String theUrl) Removes all extensions by URL.static boolean
equals
(IBaseExtension<?, ?> theLeftExtension, IBaseExtension<?, ?> theRightExtension) Compares two extensions, returns true if they have the same value and urlstatic <D,
T extends IBaseExtension<T, D>>
StringextractChildPrimitiveExtensionValue
(IBaseExtension<T, D> theExtension, String theChildExtensionUrl) Given an extension, looks for the first child extension with the given URL of theChildExtensionUrl and a primitive datatype value, and returns the String version of that value.static IBaseExtension
<?, ?> getExtensionByUrl
(IBase theBase, String theExtensionUrl) Gets the first extension with the specified URLgetExtensionPrimitiveValues
(IBase theBase, String theExtensionUrl) Given a resource or other structure that can have direct extensions, pulls out any extensions that have the given theExtensionUrl and a primitive value type, and returns a list of the string version of the extension values.static List
<IBaseExtension<?, ?>> getExtensionsByUrl
(IBaseHasExtensions theBase, String theExtensionUrl) Gets all extensions with the specified URLstatic List
<IBaseExtension<?, ?>> getExtensionsMatchingPredicate
(IBase theBase, Predicate<? super IBaseExtension<?, ?>> theFilter) Gets all extensions that match the specified filter predicatestatic IBaseExtension
<?, ?> getOrCreateExtension
(IBase theBase, String theUrl) Returns an extension with the specified URL creating one if it doesn't exist.static boolean
hasExtension
(IBase theBase, String theExtensionUrl) Checks if the specified instance has an extension with the specified URLstatic boolean
hasExtension
(IBase theBase, String theExtensionUrl, String theExtensionValue) Checks if the specified instance has an extension with the specified URLstatic void
setExtension
(FhirContext theFhirContext, IBaseExtension<?, ?> theExtension, String theValue) Sets value of the extension as a stringstatic void
setExtension
(FhirContext theFhirContext, IBaseExtension<?, ?> theExtension, String theExtensionType, Object theValue) Sets value of the extensionstatic void
setExtension
(FhirContext theFhirContext, IBase theBase, String theUrl, String theValueType, Object theValue) Sets or replaces existing extension with the specified valuestatic void
setExtensionAsString
(FhirContext theFhirContext, IBase theBase, String theUrl, String theValue) Sets or replaces existing extension with the specified value as a string
-
Method Details
-
getOrCreateExtension
Returns an extension with the specified URL creating one if it doesn't exist.- Parameters:
theBase
- Base resource to get extension fromtheUrl
- URL for the extension- Returns:
- Returns a extension with the specified URL.
- Throws:
IllegalArgumentException
- IllegalArgumentException is thrown in case resource doesn't support extensions
-
addExtension
Returns an new empty extension.- Parameters:
theBase
- Base resource to add the extension to- Returns:
- Returns a new extension
- Throws:
IllegalArgumentException
- IllegalArgumentException is thrown in case resource doesn't support extensions
-
addExtension
Returns an extension with the specified URL- Parameters:
theBase
- Base resource to add the extension totheUrl
- URL for the extension- Returns:
- Returns a new extension with the specified URL.
- Throws:
IllegalArgumentException
- IllegalArgumentException is thrown in case resource doesn't support extensions
-
addExtension
public static void addExtension(FhirContext theFhirContext, IBase theBase, String theUrl, String theValueType, Object theValue) Adds an extension with the specified value- Parameters:
theFhirContext
- The context containing FHIR resource definitionstheBase
- The resource to update extension ontheUrl
- Extension URLtheValueType
- Type of the value to set in the extensiontheValue
- Extension value
-
hasExtension
Checks if the specified instance has an extension with the specified URL- Parameters:
theBase
- The base resource to check extensions ontheExtensionUrl
- URL of the extension- Returns:
- Returns true if extension is exists and false otherwise
-
hasExtension
Checks if the specified instance has an extension with the specified URL- Parameters:
theBase
- The base resource to check extensions ontheExtensionUrl
- URL of the extension- Returns:
- Returns true if extension is exists and false otherwise
-
getExtensionByUrl
Gets the first extension with the specified URL- Parameters:
theBase
- The resource to get the extension fortheExtensionUrl
- URL of the extension to get. Must be non-null- Returns:
- Returns the first available extension with the specified URL, or null if such extension doesn't exist
-
getExtensionPrimitiveValues
Given a resource or other structure that can have direct extensions, pulls out any extensions that have the given theExtensionUrl and a primitive value type, and returns a list of the string version of the extension values. -
getExtensionsMatchingPredicate
public static List<IBaseExtension<?,?>> getExtensionsMatchingPredicate(IBase theBase, Predicate<? super IBaseExtension<?, ?>> theFilter) Gets all extensions that match the specified filter predicate- Parameters:
theBase
- The resource to get the extension fortheFilter
- Predicate to match the extension against- Returns:
- Returns all extension with the specified URL, or an empty list if such extensions do not exist
-
clearAllExtensions
Removes all extensions.- Parameters:
theBase
- The resource to clear the extension for- Returns:
- Returns all extension that were removed
-
clearExtensionsByUrl
Removes all extensions by URL.- Parameters:
theBase
- The resource to clear the extension fortheUrl
- The url to clear extensions for- Returns:
- Returns all extension that were removed
-
getExtensionsByUrl
public static List<IBaseExtension<?,?>> getExtensionsByUrl(IBaseHasExtensions theBase, String theExtensionUrl) Gets all extensions with the specified URL- Parameters:
theBase
- The resource to get the extension fortheExtensionUrl
- URL of the extension to get. Must be non-null- Returns:
- Returns all extension with the specified URL, or an empty list if such extensions do not exist
-
setExtension
public static void setExtension(FhirContext theFhirContext, IBaseExtension<?, ?> theExtension, String theValue) Sets value of the extension as a string- Parameters:
theFhirContext
- The context containing FHIR resource definitionstheExtension
- The extension to set the value ontheValue
- The value to set
-
setExtension
public static void setExtension(FhirContext theFhirContext, IBaseExtension<?, ?> theExtension, String theExtensionType, Object theValue) Sets value of the extension- Parameters:
theFhirContext
- The context containing FHIR resource definitionstheExtension
- The extension to set the value ontheExtensionType
- Element type of the extensiontheValue
- The value to set
-
setExtensionAsString
public static void setExtensionAsString(FhirContext theFhirContext, IBase theBase, String theUrl, String theValue) Sets or replaces existing extension with the specified value as a string- Parameters:
theFhirContext
- The context containing FHIR resource definitionstheBase
- The resource to update extension ontheUrl
- Extension URLtheValue
- Extension value
-
setExtension
public static void setExtension(FhirContext theFhirContext, IBase theBase, String theUrl, String theValueType, Object theValue) Sets or replaces existing extension with the specified value- Parameters:
theFhirContext
- The context containing FHIR resource definitionstheBase
- The resource to update extension ontheUrl
- Extension URLtheValueType
- Type of the value to set in the extensiontheValue
- Extension value
-
equals
public static boolean equals(IBaseExtension<?, ?> theLeftExtension, IBaseExtension<?, ?> theRightExtension) Compares two extensions, returns true if they have the same value and url- Parameters:
theLeftExtension
- : Extension to be evaluated #1theRightExtension
- : Extension to be evaluated #2- Returns:
- Result of the comparison
-
extractChildPrimitiveExtensionValue
public static <D,T extends IBaseExtension<T, String extractChildPrimitiveExtensionValueD>> (@Nonnull IBaseExtension<T, D> theExtension, @Nonnull String theChildExtensionUrl) Given an extension, looks for the first child extension with the given URL of theChildExtensionUrl and a primitive datatype value, and returns the String version of that value. E.g. if the value is a FHIR boolean, it would return the string "true" or "false. If the extension has no value, or the value is not a primitive datatype, or the URL is not found, the method will return null.- Parameters:
theExtension
- The parent extension. Must not be null.theChildExtensionUrl
- The child extension URL. Must not be null or blank.- Since:
- 6.6.0
-