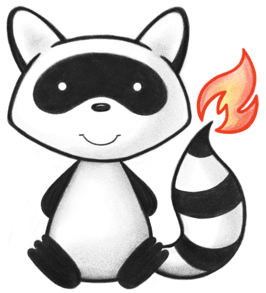
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.annotation.Child; 024import ca.uhn.fhir.model.api.annotation.Description; 025import ca.uhn.fhir.model.base.composite.BaseContainedDt; 026import org.hl7.fhir.instance.model.api.IBase; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028 029import java.lang.reflect.Field; 030import java.util.Collections; 031import java.util.List; 032import java.util.Map; 033import java.util.Set; 034 035public class RuntimeChildContainedResources extends BaseRuntimeDeclaredChildDefinition { 036 037 private BaseRuntimeElementDefinition<?> myElem; 038 039 RuntimeChildContainedResources( 040 Field theField, Child theChildAnnotation, Description theDescriptionAnnotation, String theElementName) 041 throws ConfigurationException { 042 super(theField, theChildAnnotation, theDescriptionAnnotation, theElementName); 043 } 044 045 @Override 046 public int getMax() { 047 return Child.MAX_UNLIMITED; 048 } 049 050 @Override 051 public int getMin() { 052 return 0; 053 } 054 055 @Override 056 public BaseRuntimeElementDefinition<?> getChildByName(String theName) { 057 assert theName.equals(getElementName()); 058 return myElem; 059 } 060 061 @Override 062 public BaseRuntimeElementDefinition<?> getChildElementDefinitionByDatatype(Class<? extends IBase> theType) { 063 return myElem; 064 } 065 066 @Override 067 public String getChildNameByDatatype(Class<? extends IBase> theType) { 068 return getElementName(); 069 } 070 071 @Override 072 public Set<String> getValidChildNames() { 073 return Collections.singleton(getElementName()); 074 } 075 076 @Override 077 void sealAndInitialize( 078 FhirContext theContext, 079 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 080 Class<?> actualType = theContext.getVersion().getContainedType(); 081 if (BaseContainedDt.class.isAssignableFrom(actualType)) { 082 @SuppressWarnings("unchecked") 083 Class<? extends BaseContainedDt> type = (Class<? extends BaseContainedDt>) actualType; 084 myElem = new RuntimeElemContainedResources(type, false); 085 } else if (List.class.isAssignableFrom(actualType)) { 086 myElem = new RuntimeElemContainedResourceList(IBaseResource.class, false); 087 } else { 088 throw new ConfigurationException( 089 Msg.code(1735) + "Fhir Version definition returned invalid contained type: " + actualType); 090 } 091 } 092}