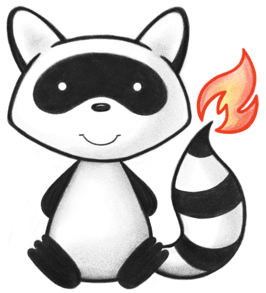
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import ca.uhn.fhir.model.api.annotation.Child; 023import ca.uhn.fhir.model.api.annotation.Description; 024import org.hl7.fhir.instance.model.api.IBase; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027import java.lang.reflect.Field; 028import java.util.Collections; 029import java.util.Map; 030import java.util.Set; 031 032public class RuntimeChildDirectResource extends BaseRuntimeDeclaredChildDefinition { 033 034 // private RuntimeElemContainedResources myElem; 035 private FhirContext myContext; 036 037 RuntimeChildDirectResource( 038 Field theField, Child theChildAnnotation, Description theDescriptionAnnotation, String theElementName) 039 throws ConfigurationException { 040 super(theField, theChildAnnotation, theDescriptionAnnotation, theElementName); 041 } 042 043 @Override 044 public BaseRuntimeElementDefinition<?> getChildByName(String theName) { 045 return new RuntimeElementDirectResource(false); 046 } 047 048 @SuppressWarnings("unchecked") 049 @Override 050 public BaseRuntimeElementDefinition<?> getChildElementDefinitionByDatatype(Class<? extends IBase> theType) { 051 return myContext.getResourceDefinition((Class<? extends IBaseResource>) theType); 052 } 053 054 @Override 055 public String getChildNameByDatatype(Class<? extends IBase> theDatatype) { 056 return getElementName(); 057 } 058 059 @Override 060 public Set<String> getValidChildNames() { 061 return Collections.singleton(getElementName()); 062 } 063 064 @Override 065 void sealAndInitialize( 066 FhirContext theContext, 067 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 068 myContext = theContext; 069 } 070}