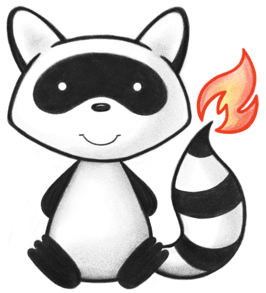
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import com.google.common.collect.Sets; 023import org.apache.commons.lang3.Validate; 024import org.hl7.fhir.instance.model.api.IBase; 025import org.hl7.fhir.instance.model.api.IBaseExtension; 026import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 027 028import java.util.Collections; 029import java.util.HashMap; 030import java.util.List; 031import java.util.Map; 032import java.util.Set; 033 034public class RuntimeChildExt extends BaseRuntimeChildDefinition { 035 036 private Map<String, BaseRuntimeElementDefinition<?>> myNameToChild; 037 private Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> myDatatypeToChild; 038 private Map<Class<? extends IBase>, String> myDatatypeToChildName; 039 040 @Override 041 public IAccessor getAccessor() { 042 return new IAccessor() { 043 @SuppressWarnings({"unchecked", "rawtypes"}) 044 @Override 045 public List<IBase> getValues(IBase theTarget) { 046 List extension = ((IBaseHasExtensions) theTarget).getExtension(); 047 return Collections.unmodifiableList(extension); 048 } 049 }; 050 } 051 052 @Override 053 public BaseRuntimeElementDefinition<?> getChildByName(String theName) { 054 return myNameToChild.get(theName); 055 } 056 057 @Override 058 public BaseRuntimeElementDefinition<?> getChildElementDefinitionByDatatype(Class<? extends IBase> theType) { 059 return myDatatypeToChild.get(theType); 060 } 061 062 @Override 063 public String getChildNameByDatatype(Class<? extends IBase> theDatatype) { 064 return myDatatypeToChildName.get(theDatatype); 065 } 066 067 @Override 068 public String getElementName() { 069 return "extension"; 070 } 071 072 @Override 073 public int getMax() { 074 return -1; 075 } 076 077 @Override 078 public int getMin() { 079 return 0; 080 } 081 082 @Override 083 public IMutator getMutator() { 084 return new IMutator() { 085 @Override 086 public void addValue(IBase theTarget, IBase theValue) { 087 List extensions = ((IBaseHasExtensions) theTarget).getExtension(); 088 IBaseExtension<?, ?> value = (IBaseExtension<?, ?>) theValue; 089 extensions.add(value); 090 } 091 092 @Override 093 public void setValue(IBase theTarget, IBase theValue) { 094 List extensions = ((IBaseHasExtensions) theTarget).getExtension(); 095 extensions.clear(); 096 if (theValue != null) { 097 IBaseExtension<?, ?> value = (IBaseExtension<?, ?>) theValue; 098 extensions.add(value); 099 } 100 } 101 }; 102 } 103 104 @Override 105 public Set<String> getValidChildNames() { 106 return Sets.newHashSet("extension"); 107 } 108 109 @Override 110 public boolean isSummary() { 111 return false; 112 } 113 114 @Override 115 void sealAndInitialize( 116 FhirContext theContext, 117 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 118 myNameToChild = new HashMap<>(); 119 myDatatypeToChild = new HashMap<>(); 120 myDatatypeToChildName = new HashMap<>(); 121 122 for (BaseRuntimeElementDefinition<?> next : theClassToElementDefinitions.values()) { 123 if (next.getName().equals("Extension")) { 124 myNameToChild.put("extension", next); 125 myDatatypeToChild.put(next.getImplementingClass(), next); 126 myDatatypeToChildName.put(next.getImplementingClass(), "extension"); 127 } 128 } 129 130 Validate.isTrue(!myNameToChild.isEmpty()); 131 } 132}