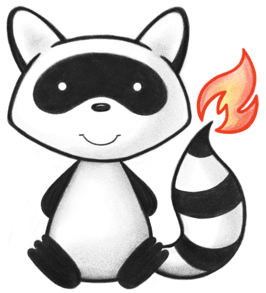
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import ca.uhn.fhir.model.api.annotation.Child; 023import ca.uhn.fhir.model.api.annotation.Description; 024import org.hl7.fhir.instance.model.api.IBase; 025 026import java.lang.reflect.Field; 027import java.util.Collections; 028import java.util.Set; 029 030public class RuntimeChildExtension extends RuntimeChildAny { 031 032 // private RuntimeChildUndeclaredExtensionDefinition myExtensionElement; 033 034 public RuntimeChildExtension( 035 Field theField, String theElementName, Child theChildAnnotation, Description theDescriptionAnnotation) { 036 super(theField, theElementName, theChildAnnotation, theDescriptionAnnotation); 037 } 038 039 @Override 040 public String getChildNameByDatatype(Class<? extends IBase> theDatatype) { 041 return getElementName(); 042 } 043 044 @Override 045 public Set<String> getValidChildNames() { 046 return Collections.singleton(getElementName()); 047 } 048 049 @Override 050 public BaseRuntimeElementDefinition<?> getChildByName(String theName) { 051 if ("extension".equals(theName) || "modifierExtension".equals(theName)) { 052 return super.getChildByName("extensionExtension"); 053 } 054 return super.getChildByName(theName); 055 } 056 057 // @Override 058 // public BaseRuntimeElementDefinition<?> getChildElementDefinitionByDatatype(Class<? extends IBase> theDatatype) { 059 // if (IBaseExtension.class.isAssignableFrom(theDatatype)) { 060 // return myExtensionElement; 061 // } 062 // return super.getChildElementDefinitionByDatatype(theDatatype); 063 // } 064 // 065 // @Override 066 // void sealAndInitialize(FhirContext theContext, Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> 067 // theClassToElementDefinitions) { 068 // super.sealAndInitialize(theContext, theClassToElementDefinitions); 069 // 070 // myExtensionElement = theContext.getRuntimeChildUndeclaredExtensionDefinition(); 071 // } 072 073}