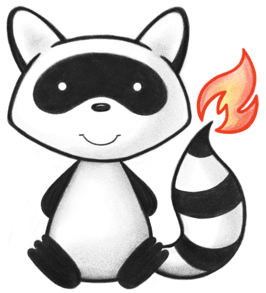
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import ca.uhn.fhir.model.api.annotation.Child; 023import ca.uhn.fhir.model.api.annotation.Description; 024import org.hl7.fhir.instance.model.api.IBase; 025 026import java.lang.reflect.Field; 027import java.util.Collections; 028import java.util.Map; 029import java.util.Set; 030 031public class RuntimeChildResourceBlockDefinition extends BaseRuntimeDeclaredChildDefinition { 032 033 // private RuntimeResourceBlockDefinition myElementDef; 034 private Class<? extends IBase> myResourceBlockType; 035 private FhirContext myContext; 036 037 public RuntimeChildResourceBlockDefinition( 038 FhirContext theContext, 039 Field theField, 040 Child theChildAnnotation, 041 Description theDescriptionAnnotation, 042 String theElementName, 043 Class<? extends IBase> theResourceBlockType) 044 throws ConfigurationException { 045 super(theField, theChildAnnotation, theDescriptionAnnotation, theElementName); 046 myContext = theContext; 047 myResourceBlockType = theResourceBlockType; 048 } 049 050 @Override 051 public BaseRuntimeElementCompositeDefinition getChildByName(String theName) { 052 if (getElementName().equals(theName)) { 053 return getDefinition(); 054 } 055 return null; 056 } 057 058 private BaseRuntimeElementCompositeDefinition getDefinition() { 059 return (BaseRuntimeElementCompositeDefinition) myContext.getElementDefinition(myResourceBlockType); 060 } 061 062 @Override 063 public String getChildNameByDatatype(Class<? extends IBase> theDatatype) { 064 if (myResourceBlockType.equals(theDatatype)) { 065 return getElementName(); 066 } 067 return null; 068 } 069 070 @Override 071 public BaseRuntimeElementDefinition<?> getChildElementDefinitionByDatatype(Class<? extends IBase> theDatatype) { 072 if (myResourceBlockType.equals(theDatatype)) { 073 return getDefinition(); 074 } 075 return null; 076 } 077 078 @Override 079 public Set<String> getValidChildNames() { 080 return Collections.singleton(getElementName()); 081 } 082 083 @Override 084 void sealAndInitialize( 085 FhirContext theContext, 086 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 087 // myElementDef = (RuntimeResourceBlockDefinition) theClassToElementDefinitions.get(myResourceBlockType); 088 } 089}