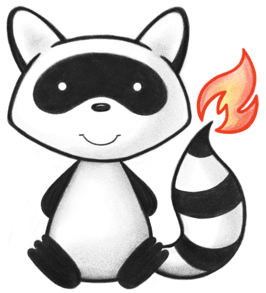
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context; 021 022import ca.uhn.fhir.model.api.annotation.DatatypeDef; 023import org.hl7.fhir.instance.model.api.IBase; 024import org.hl7.fhir.instance.model.api.ICompositeType; 025 026import java.util.ArrayList; 027import java.util.Collections; 028import java.util.List; 029import java.util.Map; 030 031public class RuntimeExtensionDtDefinition extends RuntimeCompositeDatatypeDefinition { 032 033 private List<BaseRuntimeChildDefinition> myChildren; 034 035 public RuntimeExtensionDtDefinition( 036 DatatypeDef theDef, 037 Class<? extends ICompositeType> theImplementingClass, 038 boolean theStandardType, 039 FhirContext theContext, 040 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 041 super(theDef, theImplementingClass, theStandardType, theContext, theClassToElementDefinitions); 042 } 043 044 @Override 045 public List<BaseRuntimeChildDefinition> getChildren() { 046 return myChildren; 047 } 048 049 public List<BaseRuntimeChildDefinition> getChildrenIncludingUrl() { 050 return super.getChildren(); 051 } 052 053 @Override 054 public void sealAndInitialize( 055 FhirContext theContext, 056 Map<Class<? extends IBase>, BaseRuntimeElementDefinition<?>> theClassToElementDefinitions) { 057 super.sealAndInitialize(theContext, theClassToElementDefinitions); 058 059 /* 060 * The "url" child is a weird child because it is not parsed and encoded in the normal way, 061 * so we exclude it here 062 */ 063 064 List<BaseRuntimeChildDefinition> superChildren = super.getChildren(); 065 ArrayList<BaseRuntimeChildDefinition> children = new ArrayList<BaseRuntimeChildDefinition>(); 066 for (BaseRuntimeChildDefinition baseRuntimeChildDefinition : superChildren) { 067 if (baseRuntimeChildDefinition.getValidChildNames().contains("url")) { 068 continue; 069 } 070 children.add(baseRuntimeChildDefinition); 071 } 072 073 myChildren = Collections.unmodifiableList(children); 074 } 075}