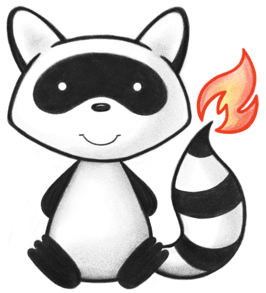
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context.support; 021 022import java.util.Collection; 023import java.util.Collections; 024import java.util.Objects; 025 026/** 027 * Represents parameters which can be passed to the $lookup operation for codes. 028 * @since 7.0.0 029 */ 030public class LookupCodeRequest { 031 private final String mySystem; 032 private final String myCode; 033 private String myDisplayLanguage; 034 private Collection<String> myPropertyNames; 035 036 /** 037 * @param theSystem The CodeSystem URL 038 * @param theCode The code 039 */ 040 public LookupCodeRequest(String theSystem, String theCode) { 041 mySystem = theSystem; 042 myCode = theCode; 043 } 044 045 /** 046 * @param theSystem The CodeSystem URL 047 * @param theCode The code 048 * @param theDisplayLanguage Used to filter out the designation by the display language. To return all designation, set this value to <code>null</code>. 049 * @param thePropertyNames The collection of properties to be returned in the output. If no properties are specified, the implementor chooses what to return. 050 */ 051 public LookupCodeRequest( 052 String theSystem, String theCode, String theDisplayLanguage, Collection<String> thePropertyNames) { 053 this(theSystem, theCode); 054 myDisplayLanguage = theDisplayLanguage; 055 myPropertyNames = thePropertyNames; 056 } 057 058 public String getSystem() { 059 return mySystem; 060 } 061 062 public String getCode() { 063 return myCode; 064 } 065 066 public String getDisplayLanguage() { 067 return myDisplayLanguage; 068 } 069 070 public Collection<String> getPropertyNames() { 071 if (myPropertyNames == null) { 072 return Collections.emptyList(); 073 } 074 return myPropertyNames; 075 } 076 077 @Override 078 public boolean equals(Object theO) { 079 if (this == theO) return true; 080 if (!(theO instanceof LookupCodeRequest)) return false; 081 LookupCodeRequest that = (LookupCodeRequest) theO; 082 return Objects.equals(mySystem, that.mySystem) 083 && Objects.equals(myCode, that.myCode) 084 && Objects.equals(myDisplayLanguage, that.myDisplayLanguage) 085 && Objects.equals(myPropertyNames, that.myPropertyNames); 086 } 087 088 @Override 089 public int hashCode() { 090 return Objects.hash(mySystem, myCode, myDisplayLanguage, myPropertyNames); 091 } 092}