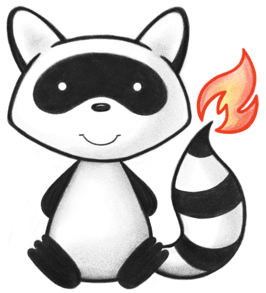
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context.support; 021 022import org.apache.commons.lang3.builder.EqualsBuilder; 023import org.apache.commons.lang3.builder.HashCodeBuilder; 024import org.apache.commons.lang3.builder.ToStringBuilder; 025import org.apache.commons.lang3.builder.ToStringStyle; 026 027public class TranslateConceptResult { 028 private String mySystem; 029 private String myCode; 030 private String myDisplay; 031 private String myEquivalence; 032 private String myConceptMapUrl; 033 private String myValueSet; 034 private String mySystemVersion; 035 036 /** 037 * Constructor 038 */ 039 public TranslateConceptResult() { 040 super(); 041 } 042 043 public String getSystem() { 044 return mySystem; 045 } 046 047 public TranslateConceptResult setSystem(String theSystem) { 048 mySystem = theSystem; 049 return this; 050 } 051 052 @Override 053 public String toString() { 054 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 055 .append("system", mySystem) 056 .append("code", myCode) 057 .append("display", myDisplay) 058 .append("equivalence", myEquivalence) 059 .append("conceptMapUrl", myConceptMapUrl) 060 .append("valueSet", myValueSet) 061 .append("systemVersion", mySystemVersion) 062 .toString(); 063 } 064 065 public String getCode() { 066 return myCode; 067 } 068 069 public TranslateConceptResult setCode(String theCode) { 070 myCode = theCode; 071 return this; 072 } 073 074 public String getDisplay() { 075 return myDisplay; 076 } 077 078 public TranslateConceptResult setDisplay(String theDisplay) { 079 myDisplay = theDisplay; 080 return this; 081 } 082 083 public String getEquivalence() { 084 return myEquivalence; 085 } 086 087 public TranslateConceptResult setEquivalence(String theEquivalence) { 088 myEquivalence = theEquivalence; 089 return this; 090 } 091 092 public String getSystemVersion() { 093 return mySystemVersion; 094 } 095 096 public void setSystemVersion(String theSystemVersion) { 097 mySystemVersion = theSystemVersion; 098 } 099 100 public String getValueSet() { 101 return myValueSet; 102 } 103 104 public TranslateConceptResult setValueSet(String theValueSet) { 105 myValueSet = theValueSet; 106 return this; 107 } 108 109 public String getConceptMapUrl() { 110 return myConceptMapUrl; 111 } 112 113 public TranslateConceptResult setConceptMapUrl(String theConceptMapUrl) { 114 myConceptMapUrl = theConceptMapUrl; 115 return this; 116 } 117 118 @Override 119 public boolean equals(Object theO) { 120 if (this == theO) { 121 return true; 122 } 123 124 if (theO == null || getClass() != theO.getClass()) { 125 return false; 126 } 127 128 TranslateConceptResult that = (TranslateConceptResult) theO; 129 130 EqualsBuilder b = new EqualsBuilder(); 131 b.append(mySystem, that.mySystem); 132 b.append(myCode, that.myCode); 133 b.append(myDisplay, that.myDisplay); 134 b.append(myEquivalence, that.myEquivalence); 135 b.append(myConceptMapUrl, that.myConceptMapUrl); 136 b.append(myValueSet, that.myValueSet); 137 b.append(mySystemVersion, that.mySystemVersion); 138 return b.isEquals(); 139 } 140 141 @Override 142 public int hashCode() { 143 HashCodeBuilder b = new HashCodeBuilder(17, 37); 144 b.append(mySystem); 145 b.append(myCode); 146 b.append(myDisplay); 147 b.append(myEquivalence); 148 b.append(myConceptMapUrl); 149 b.append(myValueSet); 150 b.append(mySystemVersion); 151 return b.toHashCode(); 152 } 153}