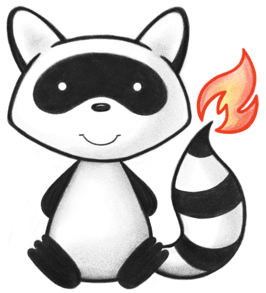
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context.support; 021 022import org.apache.commons.lang3.builder.EqualsBuilder; 023import org.apache.commons.lang3.builder.HashCodeBuilder; 024 025import java.util.ArrayList; 026import java.util.List; 027 028public class TranslateConceptResults { 029 private List<TranslateConceptResult> myResults; 030 private String myMessage; 031 private boolean myResult; 032 033 public TranslateConceptResults() { 034 super(); 035 myResults = new ArrayList<>(); 036 } 037 038 public List<TranslateConceptResult> getResults() { 039 return myResults; 040 } 041 042 public void setResults(List<TranslateConceptResult> theResults) { 043 myResults = theResults; 044 } 045 046 public String getMessage() { 047 return myMessage; 048 } 049 050 public void setMessage(String theMessage) { 051 myMessage = theMessage; 052 } 053 054 public boolean getResult() { 055 return myResult; 056 } 057 058 public void setResult(boolean theMatched) { 059 myResult = theMatched; 060 } 061 062 public int size() { 063 return getResults().size(); 064 } 065 066 public boolean isEmpty() { 067 return getResults().isEmpty(); 068 } 069 070 @Override 071 public boolean equals(Object theO) { 072 if (this == theO) { 073 return true; 074 } 075 076 if (theO == null || getClass() != theO.getClass()) { 077 return false; 078 } 079 080 TranslateConceptResults that = (TranslateConceptResults) theO; 081 082 EqualsBuilder b = new EqualsBuilder(); 083 b.append(myResults, that.myResults); 084 return b.isEquals(); 085 } 086 087 @Override 088 public int hashCode() { 089 HashCodeBuilder b = new HashCodeBuilder(17, 37); 090 b.append(myResults); 091 return b.toHashCode(); 092 } 093}