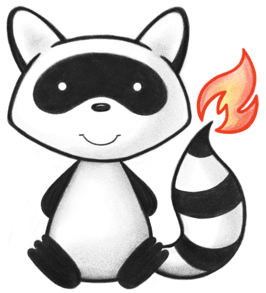
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.context.support; 021 022import org.apache.commons.lang3.Validate; 023 024/** 025 * Options for ValueSet expansion 026 * 027 * @see IValidationSupport 028 */ 029public class ValueSetExpansionOptions { 030 031 private boolean myFailOnMissingCodeSystem = true; 032 private int myCount = 1000; 033 private int myOffset = 0; 034 private boolean myIncludeHierarchy; 035 private String myFilter; 036 037 private String myDisplayLanguage; 038 039 public String getFilter() { 040 return myFilter; 041 } 042 043 public ValueSetExpansionOptions setFilter(String theFilter) { 044 myFilter = theFilter; 045 return this; 046 } 047 048 /** 049 * The number of codes to return. 050 * <p> 051 * Default is 1000 052 * </p> 053 */ 054 public int getCount() { 055 return myCount; 056 } 057 058 /** 059 * The number of codes to return. 060 * <p> 061 * Default is 1000 062 * </p> 063 */ 064 public ValueSetExpansionOptions setCount(int theCount) { 065 Validate.isTrue(theCount >= 0, "theCount must be >= 0"); 066 myCount = theCount; 067 return this; 068 } 069 070 /** 071 * The code index to start at (i.e the individual code index, not the page number) 072 */ 073 public int getOffset() { 074 return myOffset; 075 } 076 077 /** 078 * The code index to start at (i.e the individual code index, not the page number) 079 */ 080 public ValueSetExpansionOptions setOffset(int theOffset) { 081 Validate.isTrue(theOffset >= 0, "theOffset must be >= 0"); 082 myOffset = theOffset; 083 return this; 084 } 085 086 /** 087 * Should the expansion fail if a codesystem is referenced by the valueset, but 088 * it can not be found? 089 * <p> 090 * Default is <code>true</code> 091 * </p> 092 */ 093 public boolean isFailOnMissingCodeSystem() { 094 return myFailOnMissingCodeSystem; 095 } 096 097 /** 098 * Should the expansion fail if a codesystem is referenced by the valueset, but 099 * it can not be found? 100 * <p> 101 * Default is <code>true</code> 102 * </p> 103 */ 104 public ValueSetExpansionOptions setFailOnMissingCodeSystem(boolean theFailOnMissingCodeSystem) { 105 myFailOnMissingCodeSystem = theFailOnMissingCodeSystem; 106 return this; 107 } 108 109 public boolean isIncludeHierarchy() { 110 return myIncludeHierarchy; 111 } 112 113 public void setIncludeHierarchy(boolean theIncludeHierarchy) { 114 myIncludeHierarchy = theIncludeHierarchy; 115 } 116 117 public static ValueSetExpansionOptions forOffsetAndCount(int theOffset, int theCount) { 118 return new ValueSetExpansionOptions().setOffset(theOffset).setCount(theCount); 119 } 120 121 public String getTheDisplayLanguage() { 122 return myDisplayLanguage; 123 } 124 125 public ValueSetExpansionOptions setTheDisplayLanguage(String theDisplayLanguage) { 126 myDisplayLanguage = theDisplayLanguage; 127 return this; 128 } 129}