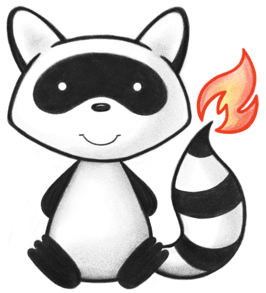
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.fhirpath; 021 022import jakarta.annotation.Nonnull; 023import org.hl7.fhir.instance.model.api.IBase; 024 025import java.util.List; 026import java.util.Optional; 027 028public interface IFhirPath { 029 030 /** 031 * Apply the given FhirPath expression against the given input and return 032 * all results in a list 033 * 034 * @param theInput The input object (generally a resource or datatype) 035 * @param thePath The fluent path expression 036 * @param theReturnType The type to return (in order to avoid casting) 037 */ 038 <T extends IBase> List<T> evaluate(IBase theInput, String thePath, Class<T> theReturnType); 039 040 /** 041 * Apply the given FhirPath expression against the given input and return 042 * all results in a list. Unlike the {@link #evaluate(IBase, String, Class)} method which 043 * uses a String containing a FHIRPath expression, this method takes a parsed FHIRPath 044 * expression returned by the {@link #parse(String)} method. This has the advantage 045 * of avoiding re-parsing expressions if the same expression will be evaluated 046 * repeatedly. 047 * 048 * @param theInput The input object (generally a resource or datatype) 049 * @param theParsedExpression A parsed FHIRPath expression returned by {@link #parse(String)} 050 * @param theReturnType The type to return (in order to avoid casting) 051 * @since 6.8.0 052 */ 053 <T extends IBase> List<T> evaluate(IBase theInput, IParsedExpression theParsedExpression, Class<T> theReturnType); 054 055 /** 056 * Apply the given FhirPath expression against the given input and return 057 * the first match (if any) 058 * 059 * @param theInput The input object (generally a resource or datatype) 060 * @param thePath The fluent path expression 061 * @param theReturnType The type to return (in order to avoid casting) 062 */ 063 <T extends IBase> Optional<T> evaluateFirst(IBase theInput, String thePath, Class<T> theReturnType); 064 065 /** 066 * Apply the given FhirPath expression against the given input and return 067 * the first match (if any). Unlike the {@link #evaluateFirst(IBase, String, Class)} method which 068 * uses a String containing a FHIRPath expression, this method takes a parsed FHIRPath 069 * expression returned by the {@link #parse(String)} method. This has the advantage 070 * of avoiding re-parsing expressions if the same expression will be evaluated 071 * repeatedly. 072 * 073 * @param theInput The input object (generally a resource or datatype) 074 * @param theParsedExpression A parsed FHIRPath expression returned by {@link #parse(String)} 075 * @param theReturnType The type to return (in order to avoid casting) 076 * @since 6.8.0 077 */ 078 <T extends IBase> Optional<T> evaluateFirst( 079 IBase theInput, IParsedExpression theParsedExpression, Class<T> theReturnType); 080 081 /** 082 * Parses the expression and throws an exception if it can not parse correctly. 083 * Note that the return type from this method is intended to be a "black box". It can 084 * be passed back into the {@link #evaluate(IBase, IParsedExpression, Class)} 085 * method on any FHIRPath instance that comes from the same {@link ca.uhn.fhir.context.FhirContext} 086 * instance. Any other use will produce unspecified results. 087 */ 088 IParsedExpression parse(String theExpression) throws Exception; 089 090 /** 091 * This method can be used optionally to supply an evaluation context for the 092 * FHIRPath evaluator instance. The context can be used to supply data needed by 093 * specific functions, e.g. allowing the <code>resolve()</code> function to 094 * fetch referenced resources. 095 * 096 * @since 6.4.0 097 */ 098 void setEvaluationContext(@Nonnull IFhirPathEvaluationContext theEvaluationContext); 099 100 /** 101 * This interface is a marker interface representing a parsed FHIRPath expression. 102 * Instances of this class will be returned by {@link #parse(String)} and can be 103 * passed to {@link #evaluate(IBase, IParsedExpression, Class)} and 104 * {@link #evaluateFirst(IBase, IParsedExpression, Class)}. Using a pre-parsed 105 * FHIRPath expression can perform much faster in some situations where an 106 * identical expression will be evaluated many times against different targets, 107 * since the parsing step doesn't need to be repeated. 108 * <p> 109 * Instances of this interface should be treated as a "black box". There are no 110 * methods that can be used to manipulate parsed FHIRPath expressions. 111 * </p> 112 * 113 * @since 6.8.0 114 */ 115 interface IParsedExpression { 116 // no methods 117 } 118}