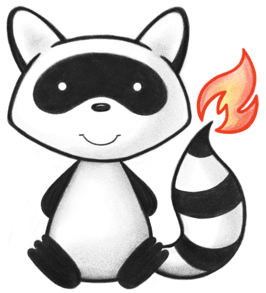
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.interceptor.api; 021 022import java.util.List; 023import java.util.function.Supplier; 024 025public interface IBaseInterceptorBroadcaster<POINTCUT extends IPointcut> { 026 027 /** 028 * Invoke registered interceptor hook methods for the given Pointcut. 029 * 030 * @return Returns <code>false</code> if any of the invoked hook methods returned 031 * <code>false</code>, and returns <code>true</code> otherwise. 032 */ 033 boolean callHooks(POINTCUT thePointcut, HookParams theParams); 034 035 /** 036 * A supplier-based callHooks() for lazy construction of the HookParameters. 037 * @return false if any hook methods return false, return true otherwise. 038 */ 039 default boolean ifHasCallHooks(POINTCUT thePointcut, Supplier<HookParams> theParamsSupplier) { 040 if (hasHooks(thePointcut)) { 041 HookParams params = theParamsSupplier.get(); 042 return callHooks(thePointcut, params); 043 } 044 return true; // callHooks returns true when none present 045 } 046 047 /** 048 * Invoke registered interceptor hook methods for the given Pointcut. This method 049 * should only be called for pointcuts that return a type other than 050 * <code>void</code> or <code>boolean</code> 051 * 052 * @return Returns the object returned by the first hook method that did not return <code>null</code> 053 */ 054 Object callHooksAndReturnObject(POINTCUT thePointcut, HookParams theParams); 055 056 /** 057 * A supplier-based version of callHooksAndReturnObject for lazy construction of the params. 058 * 059 * @return Returns the object returned by the first hook method that did not return <code>null</code> or <code>null</code> 060 */ 061 default Object ifHasCallHooksAndReturnObject(POINTCUT thePointcut, Supplier<HookParams> theParams) { 062 if (hasHooks(thePointcut)) { 063 HookParams params = theParams.get(); 064 return callHooksAndReturnObject(thePointcut, params); 065 } 066 return null; 067 } 068 069 /** 070 * Does this broadcaster have any hooks for the given pointcut? 071 * 072 * @param thePointcut The poointcut 073 * @return Does this broadcaster have any hooks for the given pointcut? 074 * @since 4.0.0 075 */ 076 boolean hasHooks(POINTCUT thePointcut); 077 078 List<IInvoker> getInvokersForPointcut(POINTCUT thePointcut); 079 080 interface IInvoker extends Comparable<IInvoker> { 081 082 Object invoke(HookParams theParams); 083 084 int getOrder(); 085 086 Object getInterceptor(); 087 088 default String getHookDescription() { 089 return toString(); 090 } 091 } 092}