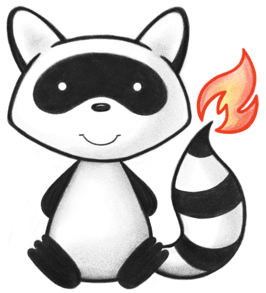
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.interceptor.api; 021 022import ca.uhn.fhir.model.base.resource.BaseOperationOutcome; 023import ca.uhn.fhir.rest.annotation.Read; 024import ca.uhn.fhir.rest.annotation.Search; 025import ca.uhn.fhir.rest.server.exceptions.AuthenticationException; 026import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 027import ca.uhn.fhir.validation.ValidationResult; 028import jakarta.annotation.Nonnull; 029import org.apache.commons.lang3.Validate; 030import org.hl7.fhir.instance.model.api.IBaseConformance; 031 032import java.io.Writer; 033import java.util.Arrays; 034import java.util.Collections; 035import java.util.HashSet; 036import java.util.List; 037import java.util.Set; 038 039/** 040 * Value for {@link Hook#value()} 041 * <p> 042 * Hook pointcuts are divided into several broad categories: 043 * <ul> 044 * <li>INTERCEPTOR_xxx: Hooks on the interceptor infrastructure itself</li> 045 * <li>CLIENT_xxx: Hooks on the HAPI FHIR Client framework</li> 046 * <li>SERVER_xxx: Hooks on the HAPI FHIR Server framework</li> 047 * <li>SUBSCRIPTION_xxx: Hooks on the HAPI FHIR Subscription framework</li> 048 * <li>STORAGE_xxx: Hooks on the storage engine</li> 049 * <li>VALIDATION_xxx: Hooks on the HAPI FHIR Validation framework</li> 050 * <li>JPA_PERFTRACE_xxx: Performance tracing hooks on the JPA server</li> 051 * </ul> 052 * </p> 053 */ 054public enum Pointcut implements IPointcut { 055 056 /** 057 * <b>Interceptor Framework Hook:</b> 058 * This pointcut will be called once when a given interceptor is registered 059 */ 060 INTERCEPTOR_REGISTERED(void.class), 061 062 /** 063 * <b>Client Hook:</b> 064 * This hook is called before an HTTP client request is sent 065 * <p> 066 * Hooks may accept the following parameters: 067 * <ul> 068 * <li> 069 * ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request 070 * </li> 071 * <li> 072 * ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request 073 * </li> 074 * </ul> 075 * </p> 076 * Hook methods must return <code>void</code>. 077 */ 078 CLIENT_REQUEST( 079 void.class, "ca.uhn.fhir.rest.client.api.IHttpRequest", "ca.uhn.fhir.rest.client.api.IRestfulClient"), 080 081 /** 082 * <b>Client Hook:</b> 083 * This hook is called after an HTTP client request has completed, prior to returning 084 * the results to the calling code. Hook methods may modify the response. 085 * <p> 086 * Hooks may accept the following parameters: 087 * <ul> 088 * <li> 089 * ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request 090 * </li> 091 * <li> 092 * ca.uhn.fhir.rest.client.api.IHttpResponse - The details of the response 093 * </li> 094 * <li> 095 * ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request 096 * </li> 097 * <li> 098 * ca.uhn.fhir.rest.client.api.ClientResponseContext - Contains an IHttpRequest, an IHttpResponse, and an IRestfulClient 099 * and also allows the client to mutate the contained IHttpResponse 100 * </li> 101 * </ul> 102 * </p> 103 * Hook methods must return <code>void</code>. 104 */ 105 CLIENT_RESPONSE( 106 void.class, 107 "ca.uhn.fhir.rest.client.api.IHttpRequest", 108 "ca.uhn.fhir.rest.client.api.IHttpResponse", 109 "ca.uhn.fhir.rest.client.api.IRestfulClient", 110 "ca.uhn.fhir.rest.client.api.ClientResponseContext"), 111 112 /** 113 * <b>Server Hook:</b> 114 * This hook is called when a server CapabilityStatement is generated for returning to a client. 115 * <p> 116 * This pointcut will not necessarily be invoked for every client request to the `/metadata` endpoint. 117 * If caching of the generated CapabilityStatement is enabled, a new CapabilityStatement will be 118 * generated periodically and this pointcut will be invoked at that time. 119 * </p> 120 * <p> 121 * Hooks may accept the following parameters: 122 * <ul> 123 * <li> 124 * org.hl7.fhir.instance.model.api.IBaseConformance - The <code>CapabilityStatement</code> resource that will 125 * be returned to the client by the server. Interceptors may make changes to this resource. The parameter 126 * must be of type <code>IBaseConformance</code>, so it is the responsibility of the interceptor hook method 127 * code to cast to the appropriate version. 128 * </li> 129 * <li> 130 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to 131 * be processed 132 * </li> 133 * <li> 134 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that 135 * is about to be processed. This parameter is identical to the RequestDetails parameter above but will only 136 * be populated when operating in a RestfulServer implementation. It is provided as a convenience. 137 * </li> 138 * </ul> 139 * </p> 140 * Hook methods may an instance of a new <code>CapabilityStatement</code> resource which will replace the 141 * one that was supplied to the interceptor, or <code>void</code> to use the original one. If the interceptor 142 * chooses to modify the <code>CapabilityStatement</code> that was supplied to the interceptor, it is fine 143 * for your hook method to return <code>void</code> or <code>null</code>. 144 */ 145 SERVER_CAPABILITY_STATEMENT_GENERATED( 146 IBaseConformance.class, 147 "org.hl7.fhir.instance.model.api.IBaseConformance", 148 "ca.uhn.fhir.rest.api.server.RequestDetails", 149 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 150 151 /** 152 * <b>Server Hook:</b> 153 * This hook is called before any other processing takes place for each incoming request. It may be used to provide 154 * alternate handling for some requests, or to screen requests before they are handled, etc. 155 * <p> 156 * Note that any exceptions thrown by this method will not be trapped by HAPI (they will be passed up to the server) 157 * </p> 158 * <p> 159 * Hooks may accept the following parameters: 160 * <ul> 161 * <li> 162 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 163 * </li> 164 * <li> 165 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 166 * </li> 167 * </ul> 168 * </p> 169 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 170 * This is generally the right thing to do. If your interceptor is providing a response rather than 171 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 172 * no further processing will occur and no further interceptors will be called. 173 */ 174 SERVER_INCOMING_REQUEST_PRE_PROCESSED( 175 boolean.class, "jakarta.servlet.http.HttpServletRequest", "jakarta.servlet.http.HttpServletResponse"), 176 177 /** 178 * <b>Server Hook:</b> 179 * This hook is invoked upon any exception being thrown within the server's request processing code. This includes 180 * any exceptions thrown within resource provider methods (e.g. {@link Search} and {@link Read} methods) as well as 181 * any runtime exceptions thrown by the server itself. This also includes any {@link AuthenticationException} 182 * thrown. 183 * <p> 184 * Hooks may accept the following parameters: 185 * <ul> 186 * <li> 187 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 188 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 189 * pulled out of the servlet request. Note that the bean 190 * properties are not all guaranteed to be populated, depending on how early during processing the 191 * exception occurred. 192 * </li> 193 * <li> 194 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 195 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 196 * pulled out of the servlet request. Note that the bean 197 * properties are not all guaranteed to be populated, depending on how early during processing the 198 * exception occurred. This parameter is identical to the RequestDetails parameter above but will 199 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 200 * </li> 201 * <li> 202 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 203 * </li> 204 * <li> 205 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 206 * </li> 207 * <li> 208 * ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException - The exception that was thrown 209 * </li> 210 * </ul> 211 * </p> 212 * <p> 213 * Implementations of this method may choose to ignore/log/count/etc exceptions, and return <code>true</code> or 214 * <code>void</code>. In 215 * this case, processing will continue, and the server will automatically generate an {@link BaseOperationOutcome 216 * OperationOutcome}. Implementations may also choose to provide their own response to the client. In this case, they 217 * should return <code>false</code>, to indicate that they have handled the request and processing should stop. 218 * </p> 219 */ 220 SERVER_HANDLE_EXCEPTION( 221 boolean.class, 222 "ca.uhn.fhir.rest.api.server.RequestDetails", 223 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 224 "jakarta.servlet.http.HttpServletRequest", 225 "jakarta.servlet.http.HttpServletResponse", 226 "ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException"), 227 228 /** 229 * <b>Server Hook:</b> 230 * This method is immediately before the handling method is selected. Interceptors may make changes 231 * to the request that can influence which handler will ultimately be called. 232 * <p> 233 * Hooks may accept the following parameters: 234 * <ul> 235 * <li> 236 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 237 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 238 * pulled out of the servlet request. 239 * Note that the bean properties are not all guaranteed to be populated at the time this hook is called. 240 * </li> 241 * <li> 242 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 243 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 244 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 245 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 246 * </li> 247 * <li> 248 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 249 * </li> 250 * <li> 251 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 252 * </li> 253 * </ul> 254 * <p> 255 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 256 * This is generally the right thing to do. 257 * If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you 258 * must return <code>false</code>. In this case, no further processing will occur and no further interceptors 259 * will be called. 260 * </p> 261 * <p> 262 * Hook methods may also throw {@link AuthenticationException} if they would like. This exception may be thrown 263 * to indicate that the interceptor has detected an unauthorized access 264 * attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. 265 * 266 * @since 5.4.0 267 */ 268 SERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED( 269 boolean.class, 270 "ca.uhn.fhir.rest.api.server.RequestDetails", 271 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 272 "jakarta.servlet.http.HttpServletRequest", 273 "jakarta.servlet.http.HttpServletResponse"), 274 275 /** 276 * <b>Server Hook:</b> 277 * This method is called just before the actual implementing server method is invoked. 278 * <p> 279 * Hooks may accept the following parameters: 280 * <ul> 281 * <li> 282 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 283 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 284 * pulled out of the servlet request. Note that the bean 285 * properties are not all guaranteed to be populated, depending on how early during processing the 286 * exception occurred. 287 * </li> 288 * <li> 289 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 290 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 291 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 292 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 293 * </li> 294 * <li> 295 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 296 * </li> 297 * <li> 298 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 299 * </li> 300 * </ul> 301 * <p> 302 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 303 * This is generally the right thing to do. 304 * If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you 305 * must return <code>false</code>. In this case, no further processing will occur and no further interceptors 306 * will be called. 307 * </p> 308 * <p> 309 * Hook methods may also throw {@link AuthenticationException} if they would like. This exception may be thrown 310 * to indicate that the interceptor has detected an unauthorized access 311 * attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. 312 */ 313 SERVER_INCOMING_REQUEST_POST_PROCESSED( 314 boolean.class, 315 "ca.uhn.fhir.rest.api.server.RequestDetails", 316 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 317 "jakarta.servlet.http.HttpServletRequest", 318 "jakarta.servlet.http.HttpServletResponse"), 319 320 /** 321 * <b>Server Hook:</b> 322 * This hook is invoked before an incoming request is processed. Note that this method is called 323 * after the server has begun preparing the response to the incoming client request. 324 * As such, it is not able to supply a response to the incoming request in the way that 325 * SERVER_INCOMING_REQUEST_PRE_PROCESSED and {@link #SERVER_INCOMING_REQUEST_POST_PROCESSED} are. 326 * At this point the request has already been passed to the handler so any changes 327 * (e.g. adding parameters) will not be considered. 328 * If you'd like to modify request parameters before they are passed to the handler, 329 * use {@link Pointcut#SERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED} or {@link Pointcut#SERVER_INCOMING_REQUEST_POST_PROCESSED}. 330 * If you are attempting to modify a search before it occurs, use {@link Pointcut#STORAGE_PRESEARCH_REGISTERED}. 331 * <p> 332 * Hooks may accept the following parameters: 333 * <ul> 334 * <li> 335 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 336 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 337 * pulled out of the servlet request. Note that the bean 338 * properties are not all guaranteed to be populated, depending on how early during processing the 339 * exception occurred. 340 * </li> 341 * <li> 342 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 343 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 344 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 345 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 346 * </li> 347 * <li> 348 * ca.uhn.fhir.rest.api.RestOperationTypeEnum - The type of operation that the FHIR server has determined that the client is trying to invoke 349 * </li> 350 * </ul> 351 * </p> 352 * <p> 353 * Hook methods must return <code>void</code> 354 * </p> 355 * <p> 356 * Hook methods method may throw a subclass of {@link BaseServerResponseException}, and processing 357 * will be aborted with an appropriate error returned to the client. 358 * </p> 359 */ 360 SERVER_INCOMING_REQUEST_PRE_HANDLED( 361 void.class, 362 "ca.uhn.fhir.rest.api.server.RequestDetails", 363 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 364 "ca.uhn.fhir.rest.api.RestOperationTypeEnum"), 365 366 /** 367 * <b>Server Hook:</b> 368 * This method is called when a resource provider method is registered and being bound 369 * by the HAPI FHIR Plain Server / RestfulServer. 370 * <p> 371 * Hooks may accept the following parameters: 372 * <ul> 373 * <li> 374 * ca.uhn.fhir.rest.server.method.BaseMethodBinding - The method binding. 375 * </li> 376 * </ul> 377 * <p> 378 * Hook methods may modify the method binding, replace it, or return <code>null</code> to cancel the binding. 379 * </p> 380 */ 381 SERVER_PROVIDER_METHOD_BOUND( 382 "ca.uhn.fhir.rest.server.method.BaseMethodBinding", "ca.uhn.fhir.rest.server.method.BaseMethodBinding"), 383 384 /** 385 * <b>Server Hook:</b> 386 * This method is called upon any exception being thrown within the server's request processing code. This includes 387 * any exceptions thrown within resource provider methods (e.g. {@link Search} and {@link Read} methods) as well as 388 * any runtime exceptions thrown by the server itself. This hook method is invoked for each interceptor (until one of them 389 * returns a non-<code>null</code> response or the end of the list is reached), after which 390 * {@link #SERVER_HANDLE_EXCEPTION} is 391 * called for each interceptor. 392 * <p> 393 * This may be used to add an OperationOutcome to a response, or to convert between exception types for any reason. 394 * </p> 395 * <p> 396 * Implementations of this method may choose to ignore/log/count/etc exceptions, and return <code>null</code>. In 397 * this case, processing will continue, and the server will automatically generate an {@link BaseOperationOutcome 398 * OperationOutcome}. Implementations may also choose to provide their own response to the client. In this case, they 399 * should return a non-<code>null</code>, to indicate that they have handled the request and processing should stop. 400 * </p> 401 * <p> 402 * Hooks may accept the following parameters: 403 * <ul> 404 * <li> 405 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 406 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 407 * pulled out of the servlet request. Note that the bean 408 * properties are not all guaranteed to be populated, depending on how early during processing the 409 * exception occurred. 410 * </li> 411 * <li> 412 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 413 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 414 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 415 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 416 * </li> 417 * <li> 418 * java.lang.Throwable - The exception that was thrown. This will often be an instance of 419 * {@link BaseServerResponseException} but will not necessarily be one (e.g. it could be a 420 * {@link NullPointerException} in the case of a bug being triggered. 421 * </li> 422 * <li> 423 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 424 * </li> 425 * <li> 426 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 427 * </li> 428 * </ul> 429 * <p> 430 * Hook methods may return a new exception to use for processing, or <code>null</code> if this interceptor is not trying to 431 * modify the exception. For example, if this interceptor has nothing to do with exception processing, it 432 * should always return <code>null</code>. If this interceptor adds an OperationOutcome to the exception, it 433 * should return an exception. 434 * </p> 435 */ 436 SERVER_PRE_PROCESS_OUTGOING_EXCEPTION( 437 BaseServerResponseException.class, 438 "ca.uhn.fhir.rest.api.server.RequestDetails", 439 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 440 "java.lang.Throwable", 441 "jakarta.servlet.http.HttpServletRequest", 442 "jakarta.servlet.http.HttpServletResponse"), 443 444 /** 445 * <b>Server Hook:</b> 446 * This method is called after the server implementation method has been called, but before any attempt 447 * to stream the response back to the client. Interceptors may examine or modify the response before it 448 * is returned, or even prevent the response. 449 * <p> 450 * Hooks may accept the following parameters: 451 * <ul> 452 * <li> 453 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 454 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 455 * pulled out of the servlet request. 456 * </li> 457 * <li> 458 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 459 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 460 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 461 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 462 * </li> 463 * <li> 464 * org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be returned. This parameter may be <code>null</code> for some responses. 465 * </li> 466 * <li> 467 * ca.uhn.fhir.rest.api.server.ResponseDetails - This object contains details about the response, including the contents. Hook methods may modify this object to change or replace the response. 468 * </li> 469 * <li> 470 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 471 * </li> 472 * <li> 473 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 474 * </li> 475 * </ul> 476 * </p> 477 * <p> 478 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 479 * This is generally the right thing to do. If your interceptor is providing a response rather than 480 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 481 * no further processing will occur and no further interceptors will be called. 482 * </p> 483 * <p> 484 * Hook methods may also throw {@link AuthenticationException} to indicate that the interceptor 485 * has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 486 * will be returned to the client. 487 */ 488 SERVER_OUTGOING_RESPONSE( 489 boolean.class, 490 "ca.uhn.fhir.rest.api.server.RequestDetails", 491 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 492 "org.hl7.fhir.instance.model.api.IBaseResource", 493 "ca.uhn.fhir.rest.api.server.ResponseDetails", 494 "jakarta.servlet.http.HttpServletRequest", 495 "jakarta.servlet.http.HttpServletResponse"), 496 497 /** 498 * <b>Server Hook:</b> 499 * This method is called when a stream writer is generated that will be used to stream a non-binary response to 500 * a client. Hooks may return a wrapped writer which adds additional functionality as needed. 501 * 502 * <p> 503 * Hooks may accept the following parameters: 504 * <ul> 505 * <li> 506 * java.io.Writer - The response writing Writer. Typically a hook will wrap this writer and layer additional functionality 507 * into the wrapping writer. 508 * </li> 509 * <li> 510 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 511 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 512 * pulled out of the servlet request. 513 * </li> 514 * <li> 515 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 516 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 517 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 518 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 519 * </li> 520 * </ul> 521 * </p> 522 * <p> 523 * Hook methods should return a {@link Writer} instance that will be used to stream the response. Hook methods 524 * should not throw any exception. 525 * </p> 526 * 527 * @since 5.0.0 528 */ 529 SERVER_OUTGOING_WRITER_CREATED( 530 Writer.class, 531 "java.io.Writer", 532 "ca.uhn.fhir.rest.api.server.RequestDetails", 533 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 534 535 /** 536 * <b>Server Hook:</b> 537 * This method is called after the server implementation method has been called, but before any attempt 538 * to stream the response back to the client, specifically for GraphQL requests (as these do not fit 539 * cleanly into the model provided by {@link #SERVER_OUTGOING_RESPONSE}). 540 * <p> 541 * Hooks may accept the following parameters: 542 * <ul> 543 * <li> 544 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 545 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 546 * pulled out of the servlet request. 547 * </li> 548 * <li> 549 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 550 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 551 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 552 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 553 * </li> 554 * <li> 555 * java.lang.String - The GraphQL query 556 * </li> 557 * <li> 558 * java.lang.String - The GraphQL response 559 * </li> 560 * <li> 561 * jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment 562 * </li> 563 * <li> 564 * jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment 565 * </li> 566 * </ul> 567 * </p> 568 * <p> 569 * Hook methods may return <code>true</code> or <code>void</code> if processing should continue normally. 570 * This is generally the right thing to do. If your interceptor is providing a response rather than 571 * letting HAPI handle the response normally, you must return <code>false</code>. In this case, 572 * no further processing will occur and no further interceptors will be called. 573 * </p> 574 * <p> 575 * Hook methods may also throw {@link AuthenticationException} to indicate that the interceptor 576 * has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 577 * will be returned to the client. 578 */ 579 SERVER_OUTGOING_GRAPHQL_RESPONSE( 580 boolean.class, 581 "ca.uhn.fhir.rest.api.server.RequestDetails", 582 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 583 "java.lang.String", 584 "java.lang.String", 585 "jakarta.servlet.http.HttpServletRequest", 586 "jakarta.servlet.http.HttpServletResponse"), 587 588 /** 589 * <b>Server Hook:</b> 590 * This method is called when an OperationOutcome is being returned in response to a failure. 591 * Hook methods may use this hook to modify the OperationOutcome being returned. 592 * <p> 593 * Hooks may accept the following parameters: 594 * <ul> 595 * <li> 596 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 597 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 598 * pulled out of the servlet request. Note that the bean 599 * properties are not all guaranteed to be populated, depending on how early during processing the 600 * exception occurred. 601 * </li> 602 * <li> 603 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 604 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 605 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 606 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 607 * </li> 608 * <li> 609 * org.hl7.fhir.instance.model.api.IBaseOperationOutcome - The OperationOutcome resource that will be 610 * returned. 611 * </ul> 612 * <p> 613 * Hook methods must return <code>void</code> 614 * </p> 615 */ 616 SERVER_OUTGOING_FAILURE_OPERATIONOUTCOME( 617 void.class, 618 "ca.uhn.fhir.rest.api.server.RequestDetails", 619 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 620 "org.hl7.fhir.instance.model.api.IBaseOperationOutcome"), 621 622 /** 623 * <b>Server Hook:</b> 624 * This method is called after all processing is completed for a request, but only if the 625 * request completes normally (i.e. no exception is thrown). 626 * <p> 627 * This pointcut is called after the response has completely finished, meaning that the HTTP response to the client 628 * may or may not have already completely been returned to the client by the time this pointcut is invoked. Use caution 629 * if you have timing-dependent logic, since there is no guarantee about whether the client will have already moved on 630 * by the time your method is invoked. If you need a guarantee that your method is invoked before returning to the 631 * client, consider using {@link #SERVER_OUTGOING_RESPONSE} instead. 632 * </p> 633 * <p> 634 * Hooks may accept the following parameters: 635 * <ul> 636 * <li> 637 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 638 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 639 * pulled out of the servlet request. 640 * </li> 641 * <li> 642 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 643 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 644 * pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment. 645 * </li> 646 * </ul> 647 * </p> 648 * <p> 649 * This method must return <code>void</code> 650 * </p> 651 * <p> 652 * This method should not throw any exceptions. Any exception that is thrown by this 653 * method will be logged, but otherwise not acted upon (i.e. even if a hook method 654 * throws an exception, processing will continue and other interceptors will be 655 * called). Therefore it is considered a bug to throw an exception from hook methods using this 656 * pointcut. 657 * </p> 658 */ 659 SERVER_PROCESSING_COMPLETED_NORMALLY( 660 void.class, 661 new ExceptionHandlingSpec().addLogAndSwallow(Throwable.class), 662 "ca.uhn.fhir.rest.api.server.RequestDetails", 663 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 664 665 /** 666 * <b>Server Hook:</b> 667 * This method is called after all processing is completed for a request, regardless of whether 668 * the request completed successfully or not. It is called after {@link #SERVER_PROCESSING_COMPLETED_NORMALLY} 669 * in the case of successful operations. 670 * <p> 671 * Hooks may accept the following parameters: 672 * <ul> 673 * <li> 674 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 675 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 676 * pulled out of the servlet request. 677 * </li> 678 * <li> 679 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 680 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 681 * pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment. 682 * </li> 683 * </ul> 684 * </p> 685 * <p> 686 * This method must return <code>void</code> 687 * </p> 688 * <p> 689 * This method should not throw any exceptions. Any exception that is thrown by this 690 * method will be logged, but otherwise not acted upon (i.e. even if a hook method 691 * throws an exception, processing will continue and other interceptors will be 692 * called). Therefore it is considered a bug to throw an exception from hook methods using this 693 * pointcut. 694 * </p> 695 */ 696 SERVER_PROCESSING_COMPLETED( 697 void.class, 698 new ExceptionHandlingSpec().addLogAndSwallow(Throwable.class), 699 "ca.uhn.fhir.rest.api.server.RequestDetails", 700 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 701 702 /** 703 * <b>Subscription Hook:</b> 704 * Invoked whenever a persisted resource has been modified and is being submitted to the 705 * subscription processing pipeline. This method is called before the resource is placed 706 * on any queues for processing and executes synchronously during the resource modification 707 * operation itself, so it should return quickly. 708 * <p> 709 * Hooks may accept the following parameters: 710 * <ul> 711 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 712 * </ul> 713 * </p> 714 * <p> 715 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 716 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 717 * returns <code>false</code>, subscription processing will not proceed for the given resource; 718 * </p> 719 */ 720 SUBSCRIPTION_RESOURCE_MODIFIED(boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 721 722 /** 723 * <b>Subscription Hook:</b> 724 * Invoked any time that a resource is matched by an individual subscription, and 725 * is about to be queued for delivery. 726 * <p> 727 * Hooks may make changes to the delivery payload, or make changes to the 728 * canonical subscription such as adding headers, modifying the channel 729 * endpoint, etc. 730 * </p> 731 * Hooks may accept the following parameters: 732 * <ul> 733 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 734 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 735 * <li>ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult</li> 736 * </ul> 737 * <p> 738 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 739 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 740 * returns <code>false</code>, delivery will be aborted. 741 * </p> 742 */ 743 SUBSCRIPTION_RESOURCE_MATCHED( 744 boolean.class, 745 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 746 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", 747 "ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult"), 748 749 /** 750 * <b>Subscription Hook:</b> 751 * Invoked whenever a persisted resource was checked against all active subscriptions, and did not 752 * match any. 753 * <p> 754 * Hooks may accept the following parameters: 755 * <ul> 756 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks should not modify this parameter as changes will not have any effect.</li> 757 * </ul> 758 * </p> 759 * <p> 760 * Hooks should return <code>void</code>. 761 * </p> 762 */ 763 SUBSCRIPTION_RESOURCE_DID_NOT_MATCH_ANY_SUBSCRIPTIONS( 764 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 765 766 /** 767 * <b>Subscription Hook:</b> 768 * Invoked immediately before the delivery of a subscription, and right before any channel-specific 769 * hooks are invoked (e.g. {@link #SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY}. 770 * <p> 771 * Hooks may make changes to the delivery payload, or make changes to the 772 * canonical subscription such as adding headers, modifying the channel 773 * endpoint, etc. 774 * </p> 775 * Hooks may accept the following parameters: 776 * <ul> 777 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 778 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 779 * </ul> 780 * <p> 781 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 782 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 783 * returns <code>false</code>, processing will be aborted. 784 * </p> 785 */ 786 SUBSCRIPTION_BEFORE_DELIVERY( 787 boolean.class, 788 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 789 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 790 791 /** 792 * <b>Subscription Hook:</b> 793 * Invoked immediately after the delivery of a subscription, and right before any channel-specific 794 * hooks are invoked (e.g. {@link #SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY}. 795 * <p> 796 * Hooks may accept the following parameters: 797 * </p> 798 * <ul> 799 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 800 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 801 * </ul> 802 * <p> 803 * Hooks should return <code>void</code>. 804 * </p> 805 */ 806 SUBSCRIPTION_AFTER_DELIVERY( 807 void.class, 808 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 809 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 810 811 /** 812 * <b>Subscription Hook:</b> 813 * Invoked immediately after the attempted delivery of a subscription, if the delivery 814 * failed. 815 * <p> 816 * Hooks may accept the following parameters: 817 * </p> 818 * <ul> 819 * <li>java.lang.Exception - The exception that caused the failure. Note this could be an exception thrown by a SUBSCRIPTION_BEFORE_DELIVERY or SUBSCRIPTION_AFTER_DELIVERY interceptor</li> 820 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage - the message that triggered the exception</li> 821 * <li>java.lang.Exception</li> 822 * </ul> 823 * <p> 824 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 825 * <code>void</code> or <code>true</code>, processing will continue normally, meaning that 826 * an exception will be thrown by the delivery mechanism. This typically means that the 827 * message will be returned to the processing queue. If the method 828 * returns <code>false</code>, processing will be aborted and no further action will be 829 * taken for the delivery. 830 * </p> 831 */ 832 SUBSCRIPTION_AFTER_DELIVERY_FAILED( 833 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", "java.lang.Exception"), 834 835 /** 836 * <b>Subscription Hook:</b> 837 * Invoked immediately after the delivery of a REST HOOK subscription. 838 * <p> 839 * When this hook is called, all processing is complete so this hook should not 840 * make any changes to the parameters. 841 * </p> 842 * Hooks may accept the following parameters: 843 * <ul> 844 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 845 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 846 * </ul> 847 * <p> 848 * Hooks should return <code>void</code>. 849 * </p> 850 */ 851 SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY( 852 void.class, 853 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 854 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 855 856 /** 857 * <b>Subscription Hook:</b> 858 * Invoked immediately before the delivery of a REST HOOK subscription. 859 * <p> 860 * Hooks may make changes to the delivery payload, or make changes to the 861 * canonical subscription such as adding headers, modifying the channel 862 * endpoint, etc. 863 * </p> 864 * Hooks may accept the following parameters: 865 * <ul> 866 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 867 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 868 * </ul> 869 * <p> 870 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 871 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 872 * returns <code>false</code>, processing will be aborted. 873 * </p> 874 */ 875 SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY( 876 boolean.class, 877 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 878 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 879 880 /** 881 * <b>Subscription Hook:</b> 882 * Invoked immediately after the delivery of MESSAGE subscription. 883 * <p> 884 * When this hook is called, all processing is complete so this hook should not 885 * make any changes to the parameters. 886 * </p> 887 * Hooks may accept the following parameters: 888 * <ul> 889 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 890 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 891 * </ul> 892 * <p> 893 * Hooks should return <code>void</code>. 894 * </p> 895 */ 896 SUBSCRIPTION_AFTER_MESSAGE_DELIVERY( 897 void.class, 898 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 899 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage"), 900 901 /** 902 * <b>Subscription Hook:</b> 903 * Invoked immediately before the delivery of a MESSAGE subscription. 904 * <p> 905 * Hooks may make changes to the delivery payload, or make changes to the 906 * canonical subscription such as adding headers, modifying the channel 907 * endpoint, etc. 908 * Furthermore, you may modify the outgoing message wrapper, for example adding headers via ResourceModifiedJsonMessage field. 909 * </p> 910 * Hooks may accept the following parameters: 911 * <ul> 912 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 913 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage</li> 914 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage</li> 915 * 916 * </ul> 917 * <p> 918 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 919 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 920 * returns <code>false</code>, processing will be aborted. 921 * </p> 922 */ 923 SUBSCRIPTION_BEFORE_MESSAGE_DELIVERY( 924 boolean.class, 925 "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription", 926 "ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage", 927 "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage"), 928 929 /** 930 * <b>Subscription Hook:</b> 931 * Invoked whenever a persisted resource (a resource that has just been stored in the 932 * database via a create/update/patch/etc.) is about to be checked for whether any subscriptions 933 * were triggered as a result of the operation. 934 * <p> 935 * Hooks may accept the following parameters: 936 * <ul> 937 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 938 * </ul> 939 * </p> 940 * <p> 941 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 942 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 943 * returns <code>false</code>, processing will be aborted. 944 * </p> 945 */ 946 SUBSCRIPTION_BEFORE_PERSISTED_RESOURCE_CHECKED( 947 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 948 949 /** 950 * <b>Subscription Hook:</b> 951 * Invoked whenever a persisted resource (a resource that has just been stored in the 952 * database via a create/update/patch/etc.) has been checked for whether any subscriptions 953 * were triggered as a result of the operation. 954 * <p> 955 * Hooks may accept the following parameters: 956 * <ul> 957 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 958 * </ul> 959 * </p> 960 * <p> 961 * Hooks should return <code>void</code>. 962 * </p> 963 */ 964 SUBSCRIPTION_AFTER_PERSISTED_RESOURCE_CHECKED( 965 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 966 967 /** 968 * <b>Subscription Hook:</b> 969 * Invoked immediately after an active subscription is "registered". In HAPI FHIR, when 970 * a subscription 971 * <p> 972 * Hooks may make changes to the canonicalized subscription and this will have an effect 973 * on processing across this server. Note however that timing issues may occur, since the 974 * subscription is already technically live by the time this hook is called. 975 * </p> 976 * Hooks may accept the following parameters: 977 * <ul> 978 * <li>ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription</li> 979 * </ul> 980 * <p> 981 * Hooks should return <code>void</code>. 982 * </p> 983 */ 984 SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_REGISTERED( 985 void.class, "ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription"), 986 987 /** 988 * <b>Subscription Hook:</b> 989 * Invoked immediately after an active subscription is "registered". In HAPI FHIR, when 990 * a subscription 991 * <p> 992 * Hooks may make changes to the canonicalized subscription and this will have an effect 993 * on processing across this server. Note however that timing issues may occur, since the 994 * subscription is already technically live by the time this hook is called. 995 * </p> 996 * No parameters are currently supported. 997 * <p> 998 * Hooks should return <code>void</code>. 999 * </p> 1000 */ 1001 SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_UNREGISTERED(void.class), 1002 1003 /** 1004 * <b>Storage Hook:</b> 1005 * Invoked when a resource is being deleted in a cascaded delete. This means that 1006 * some other resource is being deleted, but per use request or other 1007 * policy, the given resource (the one supplied as a parameter to this hook) 1008 * is also being deleted. 1009 * <p> 1010 * Hooks may accept the following parameters: 1011 * </p> 1012 * <ul> 1013 * <li> 1014 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1015 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1016 * pulled out of the servlet request. Note that the bean 1017 * properties are not all guaranteed to be populated, depending on how early during processing the 1018 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1019 * known, such as while processing searches</b> 1020 * </li> 1021 * <li> 1022 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1023 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1024 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1025 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1026 * </li> 1027 * <li> 1028 * ca.uhn.fhir.jpa.util.DeleteConflictList - Contains the details about the delete conflicts that are 1029 * being resolved via deletion. The source resource is the resource that will be deleted, and 1030 * is a cascade because the target resource is already being deleted. 1031 * </li> 1032 * <li> 1033 * org.hl7.fhir.instance.model.api.IBaseResource - The actual resource that is about to be deleted via a cascading delete 1034 * </li> 1035 * </ul> 1036 * <p> 1037 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1038 * which case the delete should be rolled back. 1039 * </p> 1040 */ 1041 STORAGE_CASCADE_DELETE( 1042 void.class, 1043 "ca.uhn.fhir.rest.api.server.RequestDetails", 1044 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1045 "ca.uhn.fhir.jpa.api.model.DeleteConflictList", 1046 "org.hl7.fhir.instance.model.api.IBaseResource"), 1047 1048 /** 1049 * <b>Subscription Topic Hook:</b> 1050 * Invoked whenever a persisted resource (a resource that has just been stored in the 1051 * database via a create/update/patch/etc.) is about to be checked for whether any subscription topics 1052 * were triggered as a result of the operation. 1053 * <p> 1054 * Hooks may accept the following parameters: 1055 * <ul> 1056 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.</li> 1057 * </ul> 1058 * </p> 1059 * <p> 1060 * Hooks may return <code>void</code> or may return a <code>boolean</code>. If the method returns 1061 * <code>void</code> or <code>true</code>, processing will continue normally. If the method 1062 * returns <code>false</code>, processing will be aborted. 1063 * </p> 1064 */ 1065 SUBSCRIPTION_TOPIC_BEFORE_PERSISTED_RESOURCE_CHECKED( 1066 boolean.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 1067 1068 /** 1069 * <b>Subscription Topic Hook:</b> 1070 * Invoked whenever a persisted resource (a resource that has just been stored in the 1071 * database via a create/update/patch/etc.) has been checked for whether any subscription topics 1072 * were triggered as a result of the operation. 1073 * <p> 1074 * Hooks may accept the following parameters: 1075 * <ul> 1076 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 1077 * </ul> 1078 * </p> 1079 * <p> 1080 * Hooks should return <code>void</code>. 1081 * </p> 1082 */ 1083 SUBSCRIPTION_TOPIC_AFTER_PERSISTED_RESOURCE_CHECKED( 1084 void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage"), 1085 1086 /** 1087 * <b>Storage Hook:</b> 1088 * Invoked when a Bulk Export job is being kicked off, but before any permission checks 1089 * have been done. 1090 * This hook can be used to modify or update parameters as need be before 1091 * authorization/permission checks are done. 1092 * <p> 1093 * Hooks may accept the following parameters: 1094 * </p> 1095 * <ul> 1096 * <li> 1097 * ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off 1098 * </li> 1099 * <li> 1100 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1101 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1102 * pulled out of the servlet request. Note that the bean 1103 * properties are not all guaranteed to be populated, depending on how early during processing the 1104 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1105 * known, such as while processing searches</b> 1106 * </li> 1107 * <li> 1108 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1109 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1110 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1111 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1112 * </li> 1113 * </ul> 1114 * <p> 1115 * Hooks should return <code>void</code>, and can throw exceptions. 1116 * </p> 1117 */ 1118 STORAGE_PRE_INITIATE_BULK_EXPORT( 1119 void.class, 1120 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1121 "ca.uhn.fhir.rest.api.server.RequestDetails", 1122 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1123 1124 /** 1125 * <b>Storage Hook:</b> 1126 * Invoked when a Bulk Export job is being kicked off. Hook methods may modify 1127 * the request, or raise an exception to prevent it from being initiated. 1128 * This hook is not guaranteed to be called before permission checks, and so 1129 * anu implementers should be cautious of changing the options in ways that would 1130 * affect permissions. 1131 * <p> 1132 * Hooks may accept the following parameters: 1133 * </p> 1134 * <ul> 1135 * <li> 1136 * ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off 1137 * </li> 1138 * <li> 1139 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1140 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1141 * pulled out of the servlet request. Note that the bean 1142 * properties are not all guaranteed to be populated, depending on how early during processing the 1143 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1144 * known, such as while processing searches</b> 1145 * </li> 1146 * <li> 1147 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1148 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1149 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1150 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1151 * </li> 1152 * </ul> 1153 * <p> 1154 * Hooks should return <code>void</code>, and can throw exceptions. 1155 * </p> 1156 */ 1157 STORAGE_INITIATE_BULK_EXPORT( 1158 void.class, 1159 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1160 "ca.uhn.fhir.rest.api.server.RequestDetails", 1161 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1162 1163 /** 1164 * <b>Storage Hook:</b> 1165 * Invoked when a Bulk Export job is being processed. If any hook method is registered 1166 * for this pointcut, the hook method will be called once for each resource that is 1167 * loaded for inclusion in a bulk export file. Hook methods may modify 1168 * the resource object and this modification will affect the copy that is stored in the 1169 * bulk export data file (but will not affect the original). Hook methods may also 1170 * return <code>false</code> in order to request that the resource be filtered 1171 * from the export. 1172 * <p> 1173 * Hooks may accept the following parameters: 1174 * </p> 1175 * <ul> 1176 * <li> 1177 * ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters - The details of the job being kicked off 1178 * </li> 1179 * <li> 1180 *org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be included in the file 1181 * </li> 1182 * </ul> 1183 * <p> 1184 * Hooks methods may return <code>false</code> to indicate that the resource should be 1185 * filtered out. Otherwise, hook methods should return <code>true</code>. 1186 * </p> 1187 * 1188 * @since 6.8.0 1189 */ 1190 STORAGE_BULK_EXPORT_RESOURCE_INCLUSION( 1191 boolean.class, 1192 "ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters", 1193 "org.hl7.fhir.instance.model.api.IBaseResource"), 1194 1195 /** 1196 * <b>Storage Hook:</b> 1197 * Invoked when a set of resources are about to be deleted and expunged via url like {@code http://localhost/Patient?active=false&_expunge=true}. 1198 * <p> 1199 * Hooks may accept the following parameters: 1200 * </p> 1201 * <ul> 1202 * <li> 1203 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1204 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1205 * pulled out of the servlet request. Note that the bean 1206 * properties are not all guaranteed to be populated, depending on how early during processing the 1207 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1208 * known, such as while processing searches</b> 1209 * </li> 1210 * <li> 1211 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1212 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1213 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1214 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1215 * </li> 1216 * <li> 1217 * java.lang.String - Contains the url used to delete and expunge the resources 1218 * </li> 1219 * </ul> 1220 * <p> 1221 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1222 * which case the delete expunge will not occur. 1223 * </p> 1224 */ 1225 STORAGE_PRE_DELETE_EXPUNGE( 1226 void.class, 1227 "ca.uhn.fhir.rest.api.server.RequestDetails", 1228 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1229 "java.lang.String"), 1230 1231 /** 1232 * <b>Storage Hook:</b> 1233 * Invoked when a batch of resource pids are about to be deleted and expunged via url like {@code http://localhost/Patient?active=false&_expunge=true}. 1234 * <p> 1235 * Hooks may accept the following parameters: 1236 * </p> 1237 * <ul> 1238 * <li> 1239 * java.lang.String - the name of the resource type being deleted 1240 * </li> 1241 * <li> 1242 * java.util.List - the list of Long pids of the resources about to be deleted 1243 * </li> 1244 * <li> 1245 * java.util.concurrent.atomic.AtomicLong - holds a running tally of all entities deleted so far. 1246 * If the pointcut callback deletes any entities, then this parameter should be incremented by the total number 1247 * of additional entities deleted. 1248 * </li> 1249 * <li> 1250 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1251 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1252 * pulled out of the servlet request. Note that the bean 1253 * properties are not all guaranteed to be populated, depending on how early during processing the 1254 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1255 * known, such as while processing searches</b> 1256 * </li> 1257 * <li> 1258 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1259 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1260 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1261 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1262 * </li> 1263 * <li> 1264 * java.lang.String - Contains the url used to delete and expunge the resources 1265 * </li> 1266 * </ul> 1267 * <p> 1268 * Hooks should return <code>void</code>. They may choose to throw an exception however, in 1269 * which case the delete expunge will not occur. 1270 * </p> 1271 */ 1272 STORAGE_PRE_DELETE_EXPUNGE_PID_LIST( 1273 void.class, 1274 "java.lang.String", 1275 "java.util.List", 1276 "java.util.concurrent.atomic.AtomicLong", 1277 "ca.uhn.fhir.rest.api.server.RequestDetails", 1278 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1279 1280 /** 1281 * <b>Storage Hook:</b> 1282 * Invoked when one or more resources may be returned to the user, whether as a part of a READ, 1283 * a SEARCH, or even as the response to a CREATE/UPDATE, etc. 1284 * <p> 1285 * This hook is invoked when a resource has been loaded by the storage engine and 1286 * is being returned to the HTTP stack for response. This is not a guarantee that the 1287 * client will ultimately see it, since filters/headers/etc may affect what 1288 * is returned but if a resource is loaded it is likely to be used. 1289 * Note also that caching may affect whether this pointcut is invoked. 1290 * </p> 1291 * <p> 1292 * Hooks will have access to the contents of the resource being returned 1293 * and may choose to make modifications. These changes will be reflected in 1294 * returned resource but have no effect on storage. 1295 * </p> 1296 * Hooks may accept the following parameters: 1297 * <ul> 1298 * <li> 1299 * ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails - Contains details about the 1300 * specific resources being returned. 1301 * </li> 1302 * <li> 1303 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1304 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1305 * pulled out of the servlet request. Note that the bean 1306 * properties are not all guaranteed to be populated, depending on how early during processing the 1307 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1308 * known, such as while processing searches</b> 1309 * </li> 1310 * <li> 1311 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1312 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1313 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1314 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1315 * </li> 1316 * </ul> 1317 * <p> 1318 * Hooks should return <code>void</code>. 1319 * </p> 1320 */ 1321 STORAGE_PREACCESS_RESOURCES( 1322 void.class, 1323 "ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails", 1324 "ca.uhn.fhir.rest.api.server.RequestDetails", 1325 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1326 1327 /** 1328 * <b>Storage Hook:</b> 1329 * Invoked when the storage engine is about to check for the existence of a pre-cached search 1330 * whose results match the given search parameters. 1331 * <p> 1332 * Hooks may accept the following parameters: 1333 * </p> 1334 * <ul> 1335 * <li> 1336 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked 1337 * </li> 1338 * <li> 1339 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1340 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1341 * pulled out of the servlet request. Note that the bean 1342 * properties are not all guaranteed to be populated, depending on how early during processing the 1343 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1344 * known, such as while processing searches</b> 1345 * </li> 1346 * <li> 1347 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1348 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1349 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1350 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1351 * </li> 1352 * </ul> 1353 * <p> 1354 * Hooks may return <code>boolean</code>. If the hook method returns 1355 * <code>false</code>, the server will not attempt to check for a cached 1356 * search no matter what. 1357 * </p> 1358 */ 1359 STORAGE_PRECHECK_FOR_CACHED_SEARCH( 1360 boolean.class, 1361 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 1362 "ca.uhn.fhir.rest.api.server.RequestDetails", 1363 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1364 1365 /** 1366 * <b>Storage Hook:</b> 1367 * Invoked when a search is starting, prior to creating a record for the search. 1368 * <p> 1369 * Hooks may accept the following parameters: 1370 * </p> 1371 * <ul> 1372 * <li> 1373 * ca.uhn.fhir.rest.server.util.ICachedSearchDetails - Contains the details of the search that 1374 * is being created and initialized. Interceptors may use this parameter to modify aspects of the search 1375 * before it is stored and executed. 1376 * </li> 1377 * <li> 1378 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1379 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1380 * pulled out of the servlet request. Note that the bean 1381 * properties are not all guaranteed to be populated, depending on how early during processing the 1382 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1383 * known, such as while processing searches</b> 1384 * </li> 1385 * <li> 1386 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1387 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1388 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1389 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1390 * </li> 1391 * <li> 1392 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked. This can be modified. 1393 * </li> 1394 * <li> 1395 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition associated with the request (or {@literal null} if the server is not partitioned) 1396 * </li> 1397 * </ul> 1398 * <p> 1399 * Hooks should return <code>void</code>. 1400 * </p> 1401 */ 1402 STORAGE_PRESEARCH_REGISTERED( 1403 void.class, 1404 "ca.uhn.fhir.rest.server.util.ICachedSearchDetails", 1405 "ca.uhn.fhir.rest.api.server.RequestDetails", 1406 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1407 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 1408 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 1409 1410 /** 1411 * <b>Storage Hook:</b> 1412 * Invoked when one or more resources may be returned to the user, whether as a part of a READ, 1413 * a SEARCH, or even as the response to a CREATE/UPDATE, etc. 1414 * <p> 1415 * This hook is invoked when a resource has been loaded by the storage engine and 1416 * is being returned to the HTTP stack for response. 1417 * This is not a guarantee that the 1418 * client will ultimately see it, since filters/headers/etc may affect what 1419 * is returned but if a resource is loaded it is likely to be used. 1420 * Note also that caching may affect whether this pointcut is invoked. 1421 * </p> 1422 * <p> 1423 * Hooks will have access to the contents of the resource being returned 1424 * and may choose to make modifications. These changes will be reflected in 1425 * returned resource but have no effect on storage. 1426 * </p> 1427 * Hooks may accept the following parameters: 1428 * <ul> 1429 * <li> 1430 * ca.uhn.fhir.rest.api.server.IPreResourceShowDetails - Contains the resources that 1431 * will be shown to the user. This object may be manipulated in order to modify 1432 * the actual resources being shown to the user (e.g. for masking) 1433 * </li> 1434 * <li> 1435 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1436 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1437 * pulled out of the servlet request. Note that the bean 1438 * properties are not all guaranteed to be populated, depending on how early during processing the 1439 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 1440 * known, such as while processing searches</b> 1441 * </li> 1442 * <li> 1443 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1444 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1445 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1446 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1447 * </li> 1448 * </ul> 1449 * <p> 1450 * Hooks should return <code>void</code>. 1451 * </p> 1452 */ 1453 STORAGE_PRESHOW_RESOURCES( 1454 void.class, 1455 "ca.uhn.fhir.rest.api.server.IPreResourceShowDetails", 1456 "ca.uhn.fhir.rest.api.server.RequestDetails", 1457 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1458 1459 /** 1460 * <b>Storage Hook:</b> 1461 * Invoked before a resource will be created, immediately before the resource 1462 * is persisted to the database. 1463 * <p> 1464 * Hooks will have access to the contents of the resource being created 1465 * and may choose to make modifications to it. These changes will be 1466 * reflected in permanent storage. 1467 * </p> 1468 * Hooks may accept the following parameters: 1469 * <ul> 1470 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1471 * <li> 1472 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1473 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1474 * pulled out of the servlet request. Note that the bean 1475 * properties are not all guaranteed to be populated, depending on how early during processing the 1476 * exception occurred. 1477 * </li> 1478 * <li> 1479 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1480 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1481 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1482 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1483 * </li> 1484 * <li> 1485 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1486 * </li> 1487 * </ul> 1488 * <p> 1489 * Hooks should return <code>void</code>. 1490 * </p> 1491 */ 1492 STORAGE_PRESTORAGE_RESOURCE_CREATED( 1493 void.class, 1494 "org.hl7.fhir.instance.model.api.IBaseResource", 1495 "ca.uhn.fhir.rest.api.server.RequestDetails", 1496 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1497 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1498 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 1499 1500 /** 1501 * <b>Storage Hook:</b> 1502 * Invoked before client-assigned id is created. 1503 * <p> 1504 * Hooks will have access to the contents of the resource being created 1505 * so that client-assigned ids can be allowed/denied. These changes will 1506 * be reflected in permanent storage. 1507 * </p> 1508 * Hooks may accept the following parameters: 1509 * <ul> 1510 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1511 * <li> 1512 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1513 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1514 * pulled out of the servlet request. Note that the bean 1515 * properties are not all guaranteed to be populated, depending on how early during processing the 1516 * exception occurred. 1517 * </li> 1518 * </ul> 1519 * <p> 1520 * Hooks should return <code>void</code>. 1521 * </p> 1522 */ 1523 STORAGE_PRESTORAGE_CLIENT_ASSIGNED_ID( 1524 void.class, "org.hl7.fhir.instance.model.api.IBaseResource", "ca.uhn.fhir.rest.api.server.RequestDetails"), 1525 1526 /** 1527 * <b>Storage Hook:</b> 1528 * Invoked before a resource will be updated, immediately before the resource 1529 * is persisted to the database. 1530 * <p> 1531 * Hooks will have access to the contents of the resource being updated 1532 * (both the previous and new contents) and may choose to make modifications 1533 * to the new contents of the resource. These changes will be reflected in 1534 * permanent storage. 1535 * </p> 1536 * <p> 1537 * <b>NO-OPS:</b> If the client has submitted an update that does not actually make any changes 1538 * (i.e. the resource they include in the PUT body is identical to the content that 1539 * was already stored) the server may choose to ignore the update and perform 1540 * a "NO-OP". In this case, this pointcut is still invoked, but {@link #STORAGE_PRECOMMIT_RESOURCE_UPDATED} 1541 * will not be. Hook methods for this pointcut may make changes to the new contents of the 1542 * resource being updated, and in this case the NO-OP will be cancelled and 1543 * {@link #STORAGE_PRECOMMIT_RESOURCE_UPDATED} will also be invoked. 1544 * </p> 1545 * Hooks may accept the following parameters: 1546 * <ul> 1547 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource being updated</li> 1548 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The new contents of the resource being updated</li> 1549 * <li> 1550 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1551 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1552 * pulled out of the servlet request. Note that the bean 1553 * properties are not all guaranteed to be populated, depending on how early during processing the 1554 * exception occurred. 1555 * </li> 1556 * <li> 1557 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1558 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1559 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1560 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1561 * </li> 1562 * <li> 1563 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1564 * </li> 1565 * </ul> 1566 * <p> 1567 * Hooks should return <code>void</code>. 1568 * </p> 1569 */ 1570 STORAGE_PRESTORAGE_RESOURCE_UPDATED( 1571 void.class, 1572 "org.hl7.fhir.instance.model.api.IBaseResource", 1573 "org.hl7.fhir.instance.model.api.IBaseResource", 1574 "ca.uhn.fhir.rest.api.server.RequestDetails", 1575 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1576 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1577 1578 /** 1579 * <b>Storage Hook:</b> 1580 * Invoked before a resource will be deleted, immediately before the resource 1581 * is removed from the database. 1582 * <p> 1583 * Hooks will have access to the contents of the resource being deleted 1584 * and may choose to make modifications related to it. These changes will be 1585 * reflected in permanent storage. 1586 * </p> 1587 * Hooks may accept the following parameters: 1588 * <ul> 1589 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted</li> 1590 * <li> 1591 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1592 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1593 * pulled out of the servlet request. Note that the bean 1594 * properties are not all guaranteed to be populated, depending on how early during processing the 1595 * exception occurred. 1596 * </li> 1597 * <li> 1598 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1599 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1600 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1601 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1602 * </li> 1603 * <li> 1604 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1605 * </li> 1606 * </ul> 1607 * <p> 1608 * Hooks should return <code>void</code>. 1609 * </p> 1610 */ 1611 STORAGE_PRESTORAGE_RESOURCE_DELETED( 1612 void.class, 1613 "org.hl7.fhir.instance.model.api.IBaseResource", 1614 "ca.uhn.fhir.rest.api.server.RequestDetails", 1615 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1616 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1617 1618 /** 1619 * <b>Storage Hook:</b> 1620 * Invoked before a resource will be created, immediately before the transaction 1621 * is committed (after all validation and other business rules have successfully 1622 * completed, and any other database activity is complete. 1623 * <p> 1624 * Hooks will have access to the contents of the resource being created 1625 * but should generally not make any 1626 * changes as storage has already occurred. Changes will not be reflected 1627 * in storage, but may be reflected in the HTTP response. 1628 * </p> 1629 * Hooks may accept the following parameters: 1630 * <ul> 1631 * <li>org.hl7.fhir.instance.model.api.IBaseResource</li> 1632 * <li> 1633 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1634 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1635 * pulled out of the servlet request. Note that the bean 1636 * properties are not all guaranteed to be populated, depending on how early during processing the 1637 * exception occurred. 1638 * </li> 1639 * <li> 1640 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1641 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1642 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1643 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1644 * </li> 1645 * <li> 1646 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1647 * </li> 1648 * <li> 1649 * Boolean - Whether this pointcut invocation was deferred or not(since 5.4.0) 1650 * </li> 1651 * <li> 1652 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1653 * </li> 1654 * </ul> 1655 * <p> 1656 * Hooks should return <code>void</code>. 1657 * </p> 1658 */ 1659 STORAGE_PRECOMMIT_RESOURCE_CREATED( 1660 void.class, 1661 "org.hl7.fhir.instance.model.api.IBaseResource", 1662 "ca.uhn.fhir.rest.api.server.RequestDetails", 1663 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1664 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1665 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1666 1667 /** 1668 * <b>Storage Hook:</b> 1669 * Invoked before a resource will be updated, immediately before the transaction 1670 * is committed (after all validation and other business rules have successfully 1671 * completed, and any other database activity is complete. 1672 * <p> 1673 * Hooks will have access to the contents of the resource being updated 1674 * (both the previous and new contents) but should generally not make any 1675 * changes as storage has already occurred. Changes will not be reflected 1676 * in storage, but may be reflected in the HTTP response. 1677 * </p> 1678 * <p> 1679 * NO-OP note: See {@link #STORAGE_PRESTORAGE_RESOURCE_UPDATED} for a note on 1680 * no-op updates when no changes are detected. 1681 * </p> 1682 * Hooks may accept the following parameters: 1683 * <ul> 1684 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource</li> 1685 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The proposed new contents of the resource</li> 1686 * <li> 1687 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1688 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1689 * pulled out of the servlet request. Note that the bean 1690 * properties are not all guaranteed to be populated, depending on how early during processing the 1691 * exception occurred. 1692 * </li> 1693 * <li> 1694 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1695 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1696 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1697 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1698 * </li> 1699 * <li> 1700 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1701 * </li> 1702 * <li> 1703 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1704 * </li> 1705 * </ul> 1706 * <p> 1707 * Hooks should return <code>void</code>. 1708 * </p> 1709 */ 1710 STORAGE_PRECOMMIT_RESOURCE_UPDATED( 1711 void.class, 1712 "org.hl7.fhir.instance.model.api.IBaseResource", 1713 "org.hl7.fhir.instance.model.api.IBaseResource", 1714 "ca.uhn.fhir.rest.api.server.RequestDetails", 1715 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1716 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1717 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1718 1719 /** 1720 * <b>Storage Hook:</b> 1721 * Invoked before a resource will be deleted 1722 * <p> 1723 * Hooks will have access to the contents of the resource being deleted 1724 * but should not make any changes as storage has already occurred 1725 * </p> 1726 * Hooks may accept the following parameters: 1727 * <ul> 1728 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted</li> 1729 * <li> 1730 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1731 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1732 * pulled out of the servlet request. Note that the bean 1733 * properties are not all guaranteed to be populated, depending on how early during processing the 1734 * exception occurred. 1735 * </li> 1736 * <li> 1737 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1738 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1739 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1740 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1741 * </li> 1742 * <li> 1743 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1744 * </li> 1745 * <li> 1746 * ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED. 1747 * </li> 1748 * </ul> 1749 * <p> 1750 * Hooks should return <code>void</code>. 1751 * </p> 1752 */ 1753 STORAGE_PRECOMMIT_RESOURCE_DELETED( 1754 void.class, 1755 "org.hl7.fhir.instance.model.api.IBaseResource", 1756 "ca.uhn.fhir.rest.api.server.RequestDetails", 1757 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1758 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails", 1759 "ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum"), 1760 1761 /** 1762 * <b>Storage Hook:</b> 1763 * Invoked when a FHIR transaction bundle is about to begin processing. Hooks may choose to 1764 * modify the bundle, and may affect processing by doing so. 1765 * <p> 1766 * Hooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the 1767 * processing of the transaction bundle 1768 * </p> 1769 * Hooks may accept the following parameters: 1770 * <ul> 1771 * <li>org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle being processed</li> 1772 * <li> 1773 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1774 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1775 * pulled out of the servlet request. 1776 * </li> 1777 * <li> 1778 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1779 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1780 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1781 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1782 * </li> 1783 * </ul> 1784 * <p> 1785 * Hooks should return <code>void</code>. 1786 * </p> 1787 * 1788 * @see #STORAGE_TRANSACTION_PROCESSED 1789 * @since 6.2.0 1790 */ 1791 STORAGE_TRANSACTION_PROCESSING( 1792 void.class, 1793 "org.hl7.fhir.instance.model.api.IBaseBundle", 1794 "ca.uhn.fhir.rest.api.server.RequestDetails", 1795 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1796 1797 /** 1798 * <b>Storage Hook:</b> 1799 * Invoked after all entries in a transaction bundle have been executed 1800 * <p> 1801 * Hooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the 1802 * processing of the transaction bundle 1803 * </p> 1804 * Hooks may accept the following parameters: 1805 * <ul> 1806 * <li>org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle that wsa processed</li> 1807 * <li> 1808 * ca.uhn.fhir.rest.api.server.storage.DeferredInterceptorBroadcasts- A collection of pointcut invocations and their parameters which were deferred. 1809 * </li> 1810 * <li> 1811 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1812 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1813 * pulled out of the servlet request. 1814 * </li> 1815 * <li> 1816 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1817 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1818 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1819 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1820 * </li> 1821 * <li> 1822 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1823 * </li> 1824 * </ul> 1825 * <p> 1826 * Hooks should return <code>void</code>. 1827 * </p> 1828 * 1829 * @see #STORAGE_TRANSACTION_PROCESSING 1830 */ 1831 STORAGE_TRANSACTION_PROCESSED( 1832 void.class, 1833 "org.hl7.fhir.instance.model.api.IBaseBundle", 1834 "ca.uhn.fhir.rest.api.server.storage.DeferredInterceptorBroadcasts", 1835 "ca.uhn.fhir.rest.api.server.RequestDetails", 1836 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1837 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1838 1839 /** 1840 * <b>Storage Hook:</b> 1841 * Invoked during a FHIR transaction, immediately before processing all write operations (i.e. immediately 1842 * before a database transaction will be opened) 1843 * <p> 1844 * Hooks may accept the following parameters: 1845 * </p> 1846 * <ul> 1847 * <li> 1848 * ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start 1849 * </li> 1850 * <li> 1851 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1852 * </li> 1853 * </ul> 1854 * <p> 1855 * Hooks should return <code>void</code>. 1856 * </p> 1857 */ 1858 STORAGE_TRANSACTION_WRITE_OPERATIONS_PRE( 1859 void.class, 1860 "ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails", 1861 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1862 1863 /** 1864 * <b>Storage Hook:</b> 1865 * Invoked during a FHIR transaction, immediately after processing all write operations (i.e. immediately 1866 * after the transaction has been committed or rolled back). This hook will always be called if 1867 * {@link #STORAGE_TRANSACTION_WRITE_OPERATIONS_PRE} has been called, regardless of whether the operation 1868 * succeeded or failed. 1869 * <p> 1870 * Hooks may accept the following parameters: 1871 * </p> 1872 * <ul> 1873 * <li> 1874 * ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start 1875 * </li> 1876 * <li> 1877 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1878 * </li> 1879 * </ul> 1880 * <p> 1881 * Hooks should return <code>void</code>. 1882 * </p> 1883 */ 1884 STORAGE_TRANSACTION_WRITE_OPERATIONS_POST( 1885 void.class, 1886 "ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails", 1887 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1888 1889 /** 1890 * <b>Storage Hook:</b> 1891 * Invoked when a resource delete operation is about to fail due to referential integrity checks. Intended for use with {@literal ca.uhn.fhir.jpa.interceptor.CascadingDeleteInterceptor}. 1892 * <p> 1893 * Hooks will have access to the list of resources that have references to the resource being deleted. 1894 * </p> 1895 * Hooks may accept the following parameters: 1896 * <ul> 1897 * <li>ca.uhn.fhir.jpa.api.model.DeleteConflictList - The list of delete conflicts</li> 1898 * <li> 1899 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1900 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1901 * pulled out of the servlet request. Note that the bean 1902 * properties are not all guaranteed to be populated, depending on how early during processing the 1903 * exception occurred. 1904 * </li> 1905 * <li> 1906 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1907 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1908 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1909 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1910 * </li> 1911 * <li> 1912 * ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0) 1913 * </li> 1914 * </ul> 1915 * <p> 1916 * Hooks should return <code>ca.uhn.fhir.jpa.delete.DeleteConflictOutcome</code>. 1917 * If the interceptor returns a non-null result, the DeleteConflictOutcome can be 1918 * used to indicate a number of times to retry. 1919 * </p> 1920 */ 1921 STORAGE_PRESTORAGE_DELETE_CONFLICTS( 1922 // Return type 1923 "ca.uhn.fhir.jpa.delete.DeleteConflictOutcome", 1924 // Params 1925 "ca.uhn.fhir.jpa.api.model.DeleteConflictList", 1926 "ca.uhn.fhir.rest.api.server.RequestDetails", 1927 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 1928 "ca.uhn.fhir.rest.api.server.storage.TransactionDetails"), 1929 1930 /** 1931 * <b>Storage Hook:</b> 1932 * Invoked before a resource is about to be expunged via the <code>$expunge</code> operation. 1933 * <p> 1934 * Hooks will be passed a reference to a counter containing the current number of records that have been deleted. 1935 * If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted. 1936 * </p> 1937 * <p> 1938 * Hooks may accept the following parameters: 1939 * </p> 1940 * <ul> 1941 * <li>java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.</li> 1942 * <li>org.hl7.fhir.instance.model.api.IIdType - The ID of the resource that is about to be deleted</li> 1943 * <li>org.hl7.fhir.instance.model.api.IBaseResource - The resource that is about to be deleted</li> 1944 * <li> 1945 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1946 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1947 * pulled out of the servlet request. Note that the bean 1948 * properties are not all guaranteed to be populated, depending on how early during processing the 1949 * exception occurred. 1950 * </li> 1951 * <li> 1952 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1953 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1954 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1955 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1956 * </li> 1957 * </ul> 1958 * <p> 1959 * Hooks should return void. 1960 * </p> 1961 */ 1962 STORAGE_PRESTORAGE_EXPUNGE_RESOURCE( 1963 // Return type 1964 void.class, 1965 // Params 1966 "java.util.concurrent.atomic.AtomicInteger", 1967 "org.hl7.fhir.instance.model.api.IIdType", 1968 "org.hl7.fhir.instance.model.api.IBaseResource", 1969 "ca.uhn.fhir.rest.api.server.RequestDetails", 1970 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 1971 1972 /** 1973 * <b>Storage Hook:</b> 1974 * Invoked before an <code>$expunge</code> operation on all data (expungeEverything) is called. 1975 * <p> 1976 * Hooks will be passed a reference to a counter containing the current number of records that have been deleted. 1977 * If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted. 1978 * </p> 1979 * Hooks may accept the following parameters: 1980 * <ul> 1981 * <li>java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.</li> 1982 * <li> 1983 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1984 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1985 * pulled out of the servlet request. Note that the bean 1986 * properties are not all guaranteed to be populated, depending on how early during processing the 1987 * exception occurred. 1988 * </li> 1989 * <li> 1990 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 1991 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 1992 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 1993 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 1994 * </li> 1995 * </ul> 1996 * <p> 1997 * Hooks should return void. 1998 * </p> 1999 */ 2000 STORAGE_PRESTORAGE_EXPUNGE_EVERYTHING( 2001 // Return type 2002 void.class, 2003 // Params 2004 "java.util.concurrent.atomic.AtomicInteger", 2005 "ca.uhn.fhir.rest.api.server.RequestDetails", 2006 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2007 2008 /** 2009 * <b>Storage Hook:</b> 2010 * Invoked before FHIR <b>create</b> operation to request the identification of the partition ID to be associated 2011 * with the resource being created. This hook will only be called if partitioning is enabled in the JPA 2012 * server. 2013 * <p> 2014 * Hooks may accept the following parameters: 2015 * </p> 2016 * <ul> 2017 * <li> 2018 * org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be created and needs a tenant ID assigned. 2019 * </li> 2020 * <li> 2021 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2022 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2023 * pulled out of the servlet request. Note that the bean 2024 * properties are not all guaranteed to be populated, depending on how early during processing the 2025 * exception occurred. 2026 * </li> 2027 * <li> 2028 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2029 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2030 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2031 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2032 * </li> 2033 * </ul> 2034 * <p> 2035 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2036 * </p> 2037 * 2038 * @see #STORAGE_PARTITION_IDENTIFY_ANY For an alternative that is not read/write specific 2039 */ 2040 STORAGE_PARTITION_IDENTIFY_CREATE( 2041 // Return type 2042 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2043 // Params 2044 "org.hl7.fhir.instance.model.api.IBaseResource", 2045 "ca.uhn.fhir.rest.api.server.RequestDetails", 2046 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2047 2048 /** 2049 * <b>Storage Hook:</b> 2050 * Invoked before any FHIR read/access/extended operation (e.g. <b>read/vread</b>, <b>search</b>, <b>history</b>, 2051 * <b>$reindex</b>, etc.) operation to request the identification of the partition ID to be associated with 2052 * the resource(s) being searched for, read, etc. Essentially any operations in the JPA server that are not 2053 * creating a resource will use this pointcut. Creates will use {@link #STORAGE_PARTITION_IDENTIFY_CREATE}. 2054 * 2055 * <p> 2056 * This hook will only be called if 2057 * partitioning is enabled in the JPA server. 2058 * </p> 2059 * <p> 2060 * Hooks may accept the following parameters: 2061 * </p> 2062 * <ul> 2063 * <li> 2064 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2065 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2066 * pulled out of the servlet request. Note that the bean 2067 * properties are not all guaranteed to be populated, depending on how early during processing the 2068 * exception occurred. 2069 * </li> 2070 * <li> 2071 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2072 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2073 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2074 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2075 * </li> 2076 * <li>ca.uhn.fhir.interceptor.model.ReadPartitionIdRequestDetails - Contains details about what is being read</li> 2077 * </ul> 2078 * <p> 2079 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2080 * </p> 2081 * 2082 * @see #STORAGE_PARTITION_IDENTIFY_ANY For an alternative that is not read/write specific 2083 */ 2084 STORAGE_PARTITION_IDENTIFY_READ( 2085 // Return type 2086 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2087 // Params 2088 "ca.uhn.fhir.rest.api.server.RequestDetails", 2089 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2090 "ca.uhn.fhir.interceptor.model.ReadPartitionIdRequestDetails"), 2091 2092 /** 2093 * <b>Storage Hook:</b> 2094 * Invoked before FHIR operations to request the identification of the partition ID to be associated with the 2095 * request being made. 2096 * <p> 2097 * This hook is an alternative to {@link #STORAGE_PARTITION_IDENTIFY_READ} and {@link #STORAGE_PARTITION_IDENTIFY_CREATE} 2098 * and can be used in cases where a partition interceptor does not need knowledge of the specific resources being 2099 * accessed/read/written in order to determine the appropriate partition. 2100 * If registered, then neither STORAGE_PARTITION_IDENTIFY_READ, nor STORAGE_PARTITION_IDENTIFY_CREATE will be called. 2101 * </p> 2102 * <p> 2103 * This hook will only be called if 2104 * partitioning is enabled in the JPA server. 2105 * </p> 2106 * <p> 2107 * Hooks may accept the following parameters: 2108 * </p> 2109 * <ul> 2110 * <li> 2111 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2112 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2113 * pulled out of the servlet request. Note that the bean 2114 * properties are not all guaranteed to be populated, depending on how early during processing the 2115 * exception occurred. 2116 * </li> 2117 * <li> 2118 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2119 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2120 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2121 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2122 * </li> 2123 * </ul> 2124 * <p> 2125 * Hooks must return an instance of <code>ca.uhn.fhir.interceptor.model.RequestPartitionId</code>. 2126 * </p> 2127 * 2128 * @see #STORAGE_PARTITION_IDENTIFY_READ 2129 * @see #STORAGE_PARTITION_IDENTIFY_CREATE 2130 */ 2131 STORAGE_PARTITION_IDENTIFY_ANY( 2132 // Return type 2133 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2134 // Params 2135 "ca.uhn.fhir.rest.api.server.RequestDetails", 2136 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2137 2138 /** 2139 * <b>Storage Hook:</b> 2140 * Invoked when a partition has been created, typically meaning the <code>$partition-management-create-partition</code> 2141 * operation has been invoked. 2142 * <p> 2143 * This hook will only be called if 2144 * partitioning is enabled in the JPA server. 2145 * </p> 2146 * <p> 2147 * Hooks may accept the following parameters: 2148 * </p> 2149 * <ul> 2150 * <li> 2151 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected 2152 * </li> 2153 * <li> 2154 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2155 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2156 * pulled out of the servlet request. Note that the bean 2157 * properties are not all guaranteed to be populated, depending on how early during processing the 2158 * exception occurred. 2159 * </li> 2160 * <li> 2161 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2162 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2163 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2164 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2165 * </li> 2166 * </ul> 2167 * <p> 2168 * Hooks must return void. 2169 * </p> 2170 */ 2171 STORAGE_PARTITION_CREATED( 2172 // Return type 2173 void.class, 2174 // Params 2175 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2176 "ca.uhn.fhir.rest.api.server.RequestDetails", 2177 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2178 2179 /** 2180 * <b>Storage Hook:</b> 2181 * Invoked when a partition has been deleted, typically meaning the <code>$partition-management-delete-partition</code> 2182 * operation has been invoked. 2183 * <p> 2184 * This hook will only be called if 2185 * partitioning is enabled in the JPA server. 2186 * </p> 2187 * <p> 2188 * Hooks may accept the following parameters: 2189 * </p> 2190 * <ul> 2191 * <li> 2192 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The ID of the partition that was deleted. 2193 * </li> 2194 * </ul> 2195 * <p> 2196 * Hooks must return void. 2197 * </p> 2198 */ 2199 STORAGE_PARTITION_DELETED( 2200 // Return type 2201 void.class, 2202 // Params 2203 "ca.uhn.fhir.interceptor.model.RequestPartitionId"), 2204 2205 /** 2206 * <b>Storage Hook:</b> 2207 * Invoked before any partition aware FHIR operation, when the selected partition has been identified (ie. after the 2208 * {@link #STORAGE_PARTITION_IDENTIFY_CREATE} or {@link #STORAGE_PARTITION_IDENTIFY_READ} hook was called. This allows 2209 * a separate hook to register, and potentially make decisions about whether the request should be allowed to proceed. 2210 * <p> 2211 * This hook will only be called if 2212 * partitioning is enabled in the JPA server. 2213 * </p> 2214 * <p> 2215 * Hooks may accept the following parameters: 2216 * </p> 2217 * <ul> 2218 * <li> 2219 * ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected 2220 * </li> 2221 * <li> 2222 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2223 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2224 * pulled out of the servlet request. Note that the bean 2225 * properties are not all guaranteed to be populated, depending on how early during processing the 2226 * exception occurred. 2227 * </li> 2228 * <li> 2229 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2230 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2231 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2232 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2233 * </li> 2234 * <li> 2235 * ca.uhn.fhir.context.RuntimeResourceDefinition - The resource type being accessed, or {@literal null} if no specific type is associated with the request. 2236 * </li> 2237 * </ul> 2238 * <p> 2239 * Hooks must return void. 2240 * </p> 2241 */ 2242 STORAGE_PARTITION_SELECTED( 2243 // Return type 2244 void.class, 2245 // Params 2246 "ca.uhn.fhir.interceptor.model.RequestPartitionId", 2247 "ca.uhn.fhir.rest.api.server.RequestDetails", 2248 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2249 "ca.uhn.fhir.context.RuntimeResourceDefinition"), 2250 2251 /** 2252 * <b>Storage Hook:</b> 2253 * Invoked when a transaction has been rolled back as a result of a {@link ca.uhn.fhir.rest.server.exceptions.ResourceVersionConflictException}, 2254 * meaning that a database constraint has been violated. This pointcut allows an interceptor to specify a resolution strategy 2255 * other than simply returning the error to the client. This interceptor will be fired after the database transaction rollback 2256 * has been completed. 2257 * <p> 2258 * Hooks may accept the following parameters: 2259 * </p> 2260 * <ul> 2261 * <li> 2262 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2263 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2264 * pulled out of the servlet request. Note that the bean 2265 * properties are not all guaranteed to be populated, depending on how early during processing the 2266 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 2267 * known, such as while processing searches</b> 2268 * </li> 2269 * <li> 2270 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2271 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2272 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2273 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2274 * </li> 2275 * </ul> 2276 * <p> 2277 * Hooks should return <code>ca.uhn.fhir.jpa.api.model.ResourceVersionConflictResolutionStrategy</code>. Hooks should not 2278 * throw any exception. 2279 * </p> 2280 */ 2281 STORAGE_VERSION_CONFLICT( 2282 "ca.uhn.fhir.jpa.api.model.ResourceVersionConflictResolutionStrategy", 2283 "ca.uhn.fhir.rest.api.server.RequestDetails", 2284 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2285 2286 /** 2287 * <b>Validation Hook:</b> 2288 * This hook is called after validation has completed, regardless of whether the validation was successful or failed. 2289 * Typically this is used to modify validation results. 2290 * <p> 2291 * <b>Note on validation Pointcuts:</b> The HAPI FHIR interceptor framework is a part of the client and server frameworks and 2292 * not a part of the core FhirContext. Therefore this Pointcut is invoked by the 2293 * </p> 2294 * <p> 2295 * Hooks may accept the following parameters: 2296 * <ul> 2297 * <li> 2298 * org.hl7.fhir.instance.model.api.IBaseResource - The resource being validated, if a parsed version is available (null otherwise) 2299 * </li> 2300 * <li> 2301 * java.lang.String - The resource being validated, if a raw version is available (null otherwise) 2302 * </li> 2303 * <li> 2304 * ca.uhn.fhir.validation.ValidationResult - The outcome of the validation. Hooks methods should not modify this object, but they can return a new one. 2305 * </li> 2306 * </ul> 2307 * </p> 2308 * Hook methods may return an instance of {@link ca.uhn.fhir.validation.ValidationResult} if they wish to override the validation results, or they may return <code>null</code> or <code>void</code> otherwise. 2309 */ 2310 VALIDATION_COMPLETED( 2311 ValidationResult.class, 2312 "org.hl7.fhir.instance.model.api.IBaseResource", 2313 "java.lang.String", 2314 "ca.uhn.fhir.validation.ValidationResult"), 2315 2316 /** 2317 * <b>MDM(EMPI) Hook:</b> 2318 * Invoked when a persisted resource (a resource that has just been stored in the 2319 * database via a create/update/patch/etc.) enters the MDM module. The purpose of the pointcut is to permit a pseudo 2320 * modification of the resource elements to influence the MDM linking process. Any modifications to the resource are not persisted. 2321 * <p> 2322 * Hooks may accept the following parameters: 2323 * <ul> 2324 * <li>org.hl7.fhir.instance.model.api.IBaseResource - </li> 2325 * </ul> 2326 * </p> 2327 * <p> 2328 * Hooks should return <code>void</code>. 2329 * </p> 2330 */ 2331 MDM_BEFORE_PERSISTED_RESOURCE_CHECKED(void.class, "org.hl7.fhir.instance.model.api.IBaseResource"), 2332 2333 /** 2334 * <b>MDM(EMPI) Hook:</b> 2335 * Invoked whenever a persisted resource (a resource that has just been stored in the 2336 * database via a create/update/patch/etc.) has been matched against related resources and MDM links have been updated. 2337 * <p> 2338 * Hooks may accept the following parameters: 2339 * <ul> 2340 * <li>ca.uhn.fhir.rest.server.messaging.ResourceOperationMessage - This parameter should not be modified as processing is complete when this hook is invoked.</li> 2341 * <li>ca.uhn.fhir.rest.server.TransactionLogMessages - This parameter is for informational messages provided by the MDM module during MDM processing.</li> 2342 * <li>ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - Contains information about the change event, including target and golden resource IDs and the operation type.</li> 2343 * </ul> 2344 * </p> 2345 * <p> 2346 * Hooks should return <code>void</code>. 2347 * </p> 2348 */ 2349 MDM_AFTER_PERSISTED_RESOURCE_CHECKED( 2350 void.class, 2351 "ca.uhn.fhir.rest.server.messaging.ResourceOperationMessage", 2352 "ca.uhn.fhir.rest.server.TransactionLogMessages", 2353 "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2354 2355 /** 2356 * <b>MDM Create Link</b> 2357 * This hook is invoked after an MDM link is created, 2358 * and changes have been persisted to the database. 2359 * <p> 2360 * Hook may accept the following parameters: 2361 * </p> 2362 * <ul> 2363 * <li> 2364 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2365 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2366 * pulled out of the servlet request. 2367 * </li> 2368 * <li> 2369 * ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type. 2370 * </li> 2371 * </ul> 2372 * <p> 2373 * Hooks should return <code>void</code>. 2374 * </p> 2375 */ 2376 MDM_POST_CREATE_LINK( 2377 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2378 2379 /** 2380 * <b>MDM Update Link</b> 2381 * This hook is invoked after an MDM link is updated, 2382 * and changes have been persisted to the database. 2383 * <p> 2384 * Hook may accept the following parameters: 2385 * </p> 2386 * <ul> 2387 * <li> 2388 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2389 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2390 * pulled out of the servlet request. 2391 * </li> 2392 * <li> 2393 * ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type. 2394 * </li> 2395 * </ul> 2396 * <p> 2397 * Hooks should return <code>void</code>. 2398 * </p> 2399 */ 2400 MDM_POST_UPDATE_LINK( 2401 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2402 2403 /** 2404 * <b>MDM Merge Golden Resources</b> 2405 * This hook is invoked after 2 golden resources have been 2406 * merged together and results persisted. 2407 * <p> 2408 * Hook may accept the following parameters: 2409 * </p> 2410 * <ul> 2411 * <li> 2412 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the 2413 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2414 * pulled out of the servlet request. 2415 * </li> 2416 * <li> 2417 * ca.uhn.fhir.mdm.model.mdmevents.MdmMergeEvent - Contains information about the from and to resources. 2418 * </li> 2419 * <li> 2420 * ca.uhn.fhir.mdm.model.mdmevents.MdmTransactionContext - Contains information about the Transaction context, e.g. merge or link. 2421 * </li> 2422 * </ul> 2423 * <p> 2424 * Hooks should return <code>void</code>. 2425 * </p> 2426 */ 2427 MDM_POST_MERGE_GOLDEN_RESOURCES( 2428 void.class, 2429 "ca.uhn.fhir.rest.api.server.RequestDetails", 2430 "ca.uhn.fhir.mdm.model.mdmevents.MdmMergeEvent", 2431 "ca.uhn.fhir.mdm.model.MdmTransactionContext"), 2432 2433 /** 2434 * <b>MDM Link History Hook:</b> 2435 * This hook is invoked after link histories are queried, 2436 * but before the results are returned to the caller. 2437 * <p> 2438 * Hook may accept the following parameters: 2439 * </p> 2440 * <ul> 2441 * <li> 2442 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2443 * </li> 2444 * <li> 2445 * ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent - An MDM History Event containing 2446 * information about the requested golden resource ids and/or source ids input, and 2447 * the returned link histories. 2448 * </li> 2449 * </ul> 2450 */ 2451 MDM_POST_LINK_HISTORY( 2452 void.class, 2453 "ca.uhn.fhir.rest.api.server.RequestDetails", 2454 "ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent"), 2455 2456 /** 2457 * <b>MDM Not Duplicate/Unduplicate Hook:</b> 2458 * This hook is invoked after 2 golden resources with an existing link 2459 * of "POSSIBLE_DUPLICATE" get unlinked/unduplicated. 2460 * <p> 2461 * This hook accepts the following parameters: 2462 * </p> 2463 * <ul> 2464 * <li> 2465 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2466 * </li> 2467 * <li> 2468 * ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - the resulting final link 2469 * between the 2 golden resources; now a NO_MATCH link. 2470 * </li> 2471 * </ul> 2472 */ 2473 MDM_POST_NOT_DUPLICATE( 2474 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent"), 2475 2476 /** 2477 * <b>MDM Clear Hook:</b> 2478 * This hook is invoked when an mdm clear operation is requested. 2479 * <p> 2480 * This hook accepts the following parameters: 2481 * </p> 2482 * <ul> 2483 * <li> 2484 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2485 * </li> 2486 * <li> 2487 * ca.uhn.fhir.mdm.model.mdmevents.MdmClearEvent - the event containing information on the clear command, 2488 * including the type filter (if any) and the batch size (if any). 2489 * </li> 2490 * </ul> 2491 */ 2492 MDM_CLEAR( 2493 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmClearEvent"), 2494 2495 /** 2496 * <b>MDM Submit Hook:</b> 2497 * This hook is invoked whenever when mdm submit operation is requested. 2498 * MDM submits can be invoked in multiple ways. 2499 * Some of which accept asynchronous calling, and some of which do not. 2500 * <p> 2501 * If the MDM Submit operation is asynchronous 2502 * (typically because the Prefer: respond-async header has been provided) 2503 * this hook will be invoked after the job is submitted, but before it has 2504 * necessarily been executed. 2505 * </p> 2506 * <p> 2507 * If the MDM Submit operation is synchronous, 2508 * this hook will be invoked immediately after the submit operation 2509 * has been executed, but before the call is returned to the caller. 2510 * </p> 2511 * <ul> 2512 * <li> 2513 * On Patient Type. Can be synchronous or asynchronous. 2514 * </li> 2515 * <li> 2516 * On Practitioner Type. Can be synchronous or asynchronous. 2517 * </li> 2518 * <li> 2519 * On specific patient instances. Is always synchronous. 2520 * </li> 2521 * <li> 2522 * On specific practitioner instances. Is always synchronous. 2523 * </li> 2524 * <li> 2525 * On the server (ie, not on any resource) with or without a resource filter. 2526 * Can be synchronous or asynchronous. 2527 * </li> 2528 * </ul> 2529 * <p> 2530 * In all cases, this hook will take the following parameters: 2531 * </p> 2532 * <ul> 2533 * <li> 2534 * ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed. 2535 * </li> 2536 * <li> 2537 * ca.uhn.fhir.mdm.model.mdmevents.MdmSubmitEvent - An event with the Mdm Submit information 2538 * (urls specifying paths that will be searched for MDM submit, as well as 2539 * if this was an asynchronous request or not). 2540 * </li> 2541 * </ul> 2542 */ 2543 MDM_SUBMIT( 2544 void.class, "ca.uhn.fhir.rest.api.server.RequestDetails", "ca.uhn.fhir.mdm.model.mdmevents.MdmSubmitEvent"), 2545 2546 /** 2547 * <b>MDM_SUBMIT_PRE_MESSAGE_DELIVERY Hook:</b> 2548 * Invoked immediately before the delivery of a MESSAGE to the broker. 2549 * <p> 2550 * Hooks can make changes to the delivery payload. 2551 * Furthermore, modification can be made to the outgoing message, 2552 * for example adding headers or changing message key, 2553 * which will be used for the subsequent processing. 2554 * </p> 2555 * Hooks should accept the following parameters: 2556 * <ul> 2557 * <li>ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage</li> 2558 * </ul> 2559 */ 2560 MDM_SUBMIT_PRE_MESSAGE_DELIVERY(void.class, "ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage"), 2561 2562 /** 2563 * <b>JPA Hook:</b> 2564 * This hook is invoked when a cross-partition reference is about to be 2565 * stored in the database. 2566 * <p> 2567 * <b>This is an experimental API - It may change in the future, use with caution.</b> 2568 * </p> 2569 * <p> 2570 * Hooks may accept the following parameters: 2571 * </p> 2572 * <ul> 2573 * <li> 2574 * {@literal ca.uhn.fhir.jpa.searchparam.extractor.CrossPartitionReferenceDetails} - Contains details about the 2575 * cross partition reference. 2576 * </li> 2577 * </ul> 2578 * <p> 2579 * Hooks should return <code>void</code>. 2580 * </p> 2581 */ 2582 JPA_RESOLVE_CROSS_PARTITION_REFERENCE( 2583 "ca.uhn.fhir.jpa.model.cross.IResourceLookup", 2584 "ca.uhn.fhir.jpa.searchparam.extractor.CrossPartitionReferenceDetails"), 2585 2586 /** 2587 * <b>Performance Tracing Hook:</b> 2588 * This hook is invoked when any informational messages generated by the 2589 * SearchCoordinator are created. It is typically used to provide logging 2590 * or capture details related to a specific request. 2591 * <p> 2592 * Note that this is a performance tracing hook. Use with caution in production 2593 * systems, since calling it may (or may not) carry a cost. 2594 * </p> 2595 * Hooks may accept the following parameters: 2596 * <ul> 2597 * <li> 2598 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2599 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2600 * pulled out of the servlet request. Note that the bean 2601 * properties are not all guaranteed to be populated, depending on how early during processing the 2602 * exception occurred. 2603 * </li> 2604 * <li> 2605 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2606 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2607 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2608 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2609 * </li> 2610 * <li> 2611 * ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message 2612 * </li> 2613 * </ul> 2614 * <p> 2615 * Hooks should return <code>void</code>. 2616 * </p> 2617 */ 2618 JPA_PERFTRACE_INFO( 2619 void.class, 2620 "ca.uhn.fhir.rest.api.server.RequestDetails", 2621 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2622 "ca.uhn.fhir.jpa.model.search.StorageProcessingMessage"), 2623 2624 /** 2625 * <b>Performance Tracing Hook:</b> 2626 * This hook is invoked when any warning messages generated by the 2627 * SearchCoordinator are created. It is typically used to provide logging 2628 * or capture details related to a specific request. 2629 * <p> 2630 * Note that this is a performance tracing hook. Use with caution in production 2631 * systems, since calling it may (or may not) carry a cost. 2632 * </p> 2633 * Hooks may accept the following parameters: 2634 * <ul> 2635 * <li> 2636 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2637 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2638 * pulled out of the servlet request. Note that the bean 2639 * properties are not all guaranteed to be populated, depending on how early during processing the 2640 * exception occurred. 2641 * </li> 2642 * <li> 2643 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2644 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2645 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2646 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2647 * </li> 2648 * <li> 2649 * ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message 2650 * </li> 2651 * </ul> 2652 * <p> 2653 * Hooks should return <code>void</code>. 2654 * </p> 2655 */ 2656 JPA_PERFTRACE_WARNING( 2657 void.class, 2658 "ca.uhn.fhir.rest.api.server.RequestDetails", 2659 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2660 "ca.uhn.fhir.jpa.model.search.StorageProcessingMessage"), 2661 2662 /** 2663 * <b>Performance Tracing Hook:</b> 2664 * This hook is invoked when a search has returned the very first result 2665 * from the database. The timing on this call can be a good indicator of how 2666 * performant a query is in general. 2667 * <p> 2668 * Note that this is a performance tracing hook. Use with caution in production 2669 * systems, since calling it may (or may not) carry a cost. 2670 * </p> 2671 * Hooks may accept the following parameters: 2672 * <ul> 2673 * <li> 2674 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2675 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2676 * pulled out of the servlet request. Note that the bean 2677 * properties are not all guaranteed to be populated, depending on how early during processing the 2678 * exception occurred. 2679 * </li> 2680 * <li> 2681 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2682 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2683 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2684 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2685 * </li> 2686 * <li> 2687 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2688 * performed. Hooks should not modify this object. 2689 * </li> 2690 * </ul> 2691 * <p> 2692 * Hooks should return <code>void</code>. 2693 * </p> 2694 */ 2695 JPA_PERFTRACE_SEARCH_FIRST_RESULT_LOADED( 2696 void.class, 2697 "ca.uhn.fhir.rest.api.server.RequestDetails", 2698 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2699 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2700 2701 /** 2702 * <b>Performance Tracing Hook:</b> 2703 * This hook is invoked when an individual search query SQL SELECT statement 2704 * has completed and no more results are available from that query. Note that this 2705 * doesn't necessarily mean that no more matching results exist in the database, 2706 * since HAPI FHIR JPA batch loads results in to the query cache in chunks in order 2707 * to provide predicable results without overloading memory or the database. 2708 * <p> 2709 * Note that this is a performance tracing hook. Use with caution in production 2710 * systems, since calling it may (or may not) carry a cost. 2711 * </p> 2712 * Hooks may accept the following parameters: 2713 * <ul> 2714 * <li> 2715 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2716 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2717 * pulled out of the servlet request. Note that the bean 2718 * properties are not all guaranteed to be populated, depending on how early during processing the 2719 * exception occurred. 2720 * </li> 2721 * <li> 2722 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2723 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2724 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2725 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2726 * </li> 2727 * <li> 2728 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2729 * performed. Hooks should not modify this object. 2730 * </li> 2731 * </ul> 2732 * <p> 2733 * Hooks should return <code>void</code>. 2734 * </p> 2735 */ 2736 JPA_PERFTRACE_SEARCH_SELECT_COMPLETE( 2737 void.class, 2738 "ca.uhn.fhir.rest.api.server.RequestDetails", 2739 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2740 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2741 2742 /** 2743 * <b>Performance Tracing Hook:</b> 2744 * This hook is invoked when a search has failed for any reason. When this pointcut 2745 * is invoked, the search has completed unsuccessfully and will not be continued. 2746 * <p> 2747 * Note that this is a performance tracing hook. Use with caution in production 2748 * systems, since calling it may (or may not) carry a cost. 2749 * </p> 2750 * Hooks may accept the following parameters: 2751 * <ul> 2752 * <li> 2753 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2754 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2755 * pulled out of the servlet request. Note that the bean 2756 * properties are not all guaranteed to be populated, depending on how early during processing the 2757 * exception occurred. 2758 * </li> 2759 * <li> 2760 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2761 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2762 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2763 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2764 * </li> 2765 * <li> 2766 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2767 * performed. Hooks should not modify this object. 2768 * </li> 2769 * </ul> 2770 * <p> 2771 * Hooks should return <code>void</code>. 2772 * </p> 2773 */ 2774 JPA_PERFTRACE_SEARCH_FAILED( 2775 void.class, 2776 "ca.uhn.fhir.rest.api.server.RequestDetails", 2777 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2778 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2779 2780 /** 2781 * <b>Performance Tracing Hook:</b> 2782 * This hook is invoked when a search has completed. When this pointcut 2783 * is invoked, a pass in the Search Coordinator has completed successfully, but 2784 * not all possible resources have been loaded yet so a future paging request 2785 * may trigger a new task that will load further resources. 2786 * <p> 2787 * Note that this is a performance tracing hook. Use with caution in production 2788 * systems, since calling it may (or may not) carry a cost. 2789 * </p> 2790 * Hooks may accept the following parameters: 2791 * <ul> 2792 * <li> 2793 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2794 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2795 * pulled out of the servlet request. Note that the bean 2796 * properties are not all guaranteed to be populated, depending on how early during processing the 2797 * exception occurred. 2798 * </li> 2799 * <li> 2800 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2801 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2802 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2803 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2804 * </li> 2805 * <li> 2806 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2807 * performed. Hooks should not modify this object. 2808 * </li> 2809 * </ul> 2810 * <p> 2811 * Hooks should return <code>void</code>. 2812 * </p> 2813 */ 2814 JPA_PERFTRACE_SEARCH_PASS_COMPLETE( 2815 void.class, 2816 "ca.uhn.fhir.rest.api.server.RequestDetails", 2817 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2818 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2819 2820 /** 2821 * <b>Performance Tracing Hook:</b> 2822 * This hook is invoked when a query involving an external index (e.g. Elasticsearch) has completed. When this pointcut 2823 * is invoked, an initial list of resource IDs has been generated which will be used as part of a subsequent database query. 2824 * <p> 2825 * Note that this is a performance tracing hook. Use with caution in production 2826 * systems, since calling it may (or may not) carry a cost. 2827 * </p> 2828 * Hooks may accept the following parameters: 2829 * <ul> 2830 * <li> 2831 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2832 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2833 * pulled out of the servlet request. Note that the bean 2834 * properties are not all guaranteed to be populated, depending on how early during processing the 2835 * exception occurred. 2836 * </li> 2837 * <li> 2838 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2839 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2840 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2841 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2842 * </li> 2843 * <li> 2844 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2845 * performed. Hooks should not modify this object. 2846 * </li> 2847 * </ul> 2848 * <p> 2849 * Hooks should return <code>void</code>. 2850 * </p> 2851 */ 2852 JPA_PERFTRACE_INDEXSEARCH_QUERY_COMPLETE( 2853 void.class, 2854 "ca.uhn.fhir.rest.api.server.RequestDetails", 2855 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2856 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2857 2858 /** 2859 * <b>Performance Tracing Hook:</b> 2860 * Invoked when the storage engine is about to reuse the results of 2861 * a previously cached search. 2862 * <p> 2863 * Note that this is a performance tracing hook. Use with caution in production 2864 * systems, since calling it may (or may not) carry a cost. 2865 * </p> 2866 * <p> 2867 * Hooks may accept the following parameters: 2868 * </p> 2869 * <ul> 2870 * <li> 2871 * ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked 2872 * </li> 2873 * <li> 2874 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2875 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2876 * pulled out of the servlet request. Note that the bean 2877 * properties are not all guaranteed to be populated, depending on how early during processing the 2878 * exception occurred. <b>Note that this parameter may be null in contexts where the request is not 2879 * known, such as while processing searches</b> 2880 * </li> 2881 * <li> 2882 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2883 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2884 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2885 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2886 * </li> 2887 * </ul> 2888 * <p> 2889 * Hooks should return <code>void</code>. 2890 * </p> 2891 */ 2892 JPA_PERFTRACE_SEARCH_REUSING_CACHED( 2893 boolean.class, 2894 "ca.uhn.fhir.jpa.searchparam.SearchParameterMap", 2895 "ca.uhn.fhir.rest.api.server.RequestDetails", 2896 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails"), 2897 2898 /** 2899 * <b>Performance Tracing Hook:</b> 2900 * This hook is invoked when a search has failed for any reason. When this pointcut 2901 * is invoked, a pass in the Search Coordinator has completed successfully, and all 2902 * possible results have been fetched and loaded into the query cache. 2903 * <p> 2904 * Note that this is a performance tracing hook. Use with caution in production 2905 * systems, since calling it may (or may not) carry a cost. 2906 * </p> 2907 * Hooks may accept the following parameters: 2908 * <ul> 2909 * <li> 2910 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2911 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2912 * pulled out of the servlet request. Note that the bean 2913 * properties are not all guaranteed to be populated, depending on how early during processing the 2914 * exception occurred. 2915 * </li> 2916 * <li> 2917 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2918 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2919 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2920 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2921 * </li> 2922 * <li> 2923 * ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being 2924 * performed. Hooks should not modify this object. 2925 * </li> 2926 * </ul> 2927 * <p> 2928 * Hooks should return <code>void</code>. 2929 * </p> 2930 */ 2931 JPA_PERFTRACE_SEARCH_COMPLETE( 2932 void.class, 2933 "ca.uhn.fhir.rest.api.server.RequestDetails", 2934 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 2935 "ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails"), 2936 2937 /** 2938 * <b>Performance Tracing Hook:</b> 2939 * <p> 2940 * This hook is invoked when a search has found an individual ID. 2941 * </p> 2942 * <p> 2943 * THIS IS AN EXPERIMENTAL HOOK AND MAY BE REMOVED OR CHANGED WITHOUT WARNING. 2944 * </p> 2945 * <p> 2946 * Note that this is a performance tracing hook. Use with caution in production 2947 * systems, since calling it may (or may not) carry a cost. 2948 * </p> 2949 * <p> 2950 * Hooks may accept the following parameters: 2951 * </p> 2952 * <ul> 2953 * <li> 2954 * java.lang.Integer - The query ID 2955 * </li> 2956 * <li> 2957 * java.lang.Object - The ID 2958 * </li> 2959 * </ul> 2960 * <p> 2961 * Hooks should return <code>void</code>. 2962 * </p> 2963 */ 2964 JPA_PERFTRACE_SEARCH_FOUND_ID(void.class, "java.lang.Integer", "java.lang.Object"), 2965 2966 /** 2967 * <b>Performance Tracing Hook:</b> 2968 * This hook is invoked when a query has executed, and includes the raw SQL 2969 * statements that were executed against the database. 2970 * <p> 2971 * Note that this is a performance tracing hook. Use with caution in production 2972 * systems, since calling it may (or may not) carry a cost. 2973 * </p> 2974 * <p> 2975 * Hooks may accept the following parameters: 2976 * </p> 2977 * <ul> 2978 * <li> 2979 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2980 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2981 * pulled out of the servlet request. Note that the bean 2982 * properties are not all guaranteed to be populated, depending on how early during processing the 2983 * exception occurred. 2984 * </li> 2985 * <li> 2986 * ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the 2987 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 2988 * pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will 2989 * only be populated when operating in a RestfulServer implementation. It is provided as a convenience. 2990 * </li> 2991 * <li> 2992 * ca.uhn.fhir.jpa.util.SqlQueryList - Contains details about the raw SQL queries. 2993 * </li> 2994 * </ul> 2995 * <p> 2996 * Hooks should return <code>void</code>. 2997 * </p> 2998 */ 2999 JPA_PERFTRACE_RAW_SQL( 3000 void.class, 3001 "ca.uhn.fhir.rest.api.server.RequestDetails", 3002 "ca.uhn.fhir.rest.server.servlet.ServletRequestDetails", 3003 "ca.uhn.fhir.jpa.util.SqlQueryList"), 3004 3005 /** 3006 * <b> Deprecated but still supported. Will eventually be removed. <code>Please use Pointcut.STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX</code> </b> 3007 * <b> Binary Blob Prefix Assigning Hook:</b> 3008 * <p> 3009 * Immediately before a binary blob is stored to its eventual data sink, this hook is called. 3010 * This hook allows implementers to provide a prefix to the binary blob's ID. 3011 * This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used. 3012 * <ul> 3013 * <li> 3014 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3015 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3016 * pulled out of the servlet request. Note that the bean 3017 * properties are not all guaranteed to be populated. 3018 * </li> 3019 * <li> 3020 * org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored. 3021 * </li> 3022 * </ul> 3023 * <p> 3024 * Hooks should return <code>String</code>, which represents the full prefix to be applied to the blob. 3025 * </p> 3026 */ 3027 @Deprecated(since = "7.2.0 - Use STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX instead.") 3028 STORAGE_BINARY_ASSIGN_BLOB_ID_PREFIX( 3029 String.class, 3030 "ca.uhn.fhir.rest.api.server.RequestDetails", 3031 "org.hl7.fhir.instance.model.api.IBaseResource"), 3032 3033 /** 3034 * <b> Binary Content Prefix Assigning Hook:</b> 3035 * <p> 3036 * Immediately before binary content is stored to its eventual data sink, this hook is called. 3037 * This hook allows implementers to provide a prefix to the binary content's ID. 3038 * This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used. 3039 * <ul> 3040 * <li> 3041 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the 3042 * resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been 3043 * pulled out of the servlet request. Note that the bean 3044 * properties are not all guaranteed to be populated. 3045 * </li> 3046 * <li> 3047 * org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored. 3048 * </li> 3049 * </ul> 3050 * <p> 3051 * Hooks should return <code>String</code>, which represents the full prefix to be applied to the blob. 3052 * </p> 3053 */ 3054 STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX( 3055 String.class, 3056 "ca.uhn.fhir.rest.api.server.RequestDetails", 3057 "org.hl7.fhir.instance.model.api.IBaseResource"), 3058 3059 /** 3060 * <b>Storage Hook:</b> 3061 * Invoked before a batch job is persisted to the database. 3062 * <p> 3063 * Hooks will have access to the content of the job being created 3064 * and may choose to make modifications to it. These changes will be 3065 * reflected in permanent storage. 3066 * </p> 3067 * Hooks may accept the following parameters: 3068 * <ul> 3069 * <li> 3070 * ca.uhn.fhir.batch2.model.JobInstance 3071 * </li> 3072 * <li> 3073 * ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that lead to the creation 3074 * of the jobInstance. 3075 * </li> 3076 * </ul> 3077 * <p> 3078 * Hooks should return <code>void</code>. 3079 * </p> 3080 */ 3081 STORAGE_PRESTORAGE_BATCH_JOB_CREATE( 3082 void.class, "ca.uhn.fhir.batch2.model.JobInstance", "ca.uhn.fhir.rest.api.server.RequestDetails"), 3083 3084 /** 3085 * <b>CDS Hooks Prefetch Hook:</b> 3086 * Invoked before a CDS Hooks prefetch request is made. 3087 * Hooks may accept the following parameters: 3088 * <ul> 3089 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query, template and resolution strategy used for the request. 3090 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3091 * invocations of a prefetch request/response.</li> 3092 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3093 * </ul> 3094 * 3095 * <p> 3096 * Hooks should return <code>void</code>. 3097 * </p> 3098 */ 3099 CDS_HOOK_PREFETCH_REQUEST( 3100 void.class, 3101 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3102 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson"), 3103 3104 /** 3105 * <b>CDS Hooks Prefetch Hook:</b> 3106 * Invoked after CDS Hooks prefetch request is completed successfully. 3107 * Hooks may accept the following parameters: 3108 * <ul> 3109 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request. 3110 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3111 * invocations of a prefetch request/response.</li> 3112 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3113 * <li> "org.hl7.fhir.instance.model.api.IBaseResource" - The resource that is returned by the prefetch 3114 * request</li> 3115 * </ul> 3116 * 3117 * <p> 3118 * Hooks should return <code>void</code>. 3119 * </p> 3120 */ 3121 CDS_HOOK_PREFETCH_RESPONSE( 3122 void.class, 3123 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3124 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson", 3125 "org.hl7.fhir.instance.model.api.IBaseResource"), 3126 3127 /** 3128 * <b>CDS Hooks Prefetch Hook:</b> 3129 * Invoked after a failed CDS Hooks prefetch request. 3130 * Hooks may accept the following parameters: 3131 * <ul> 3132 * <li> "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request. 3133 * This parameter also contains a user data map <code>(String, Object)</code>, that allows data to be store between pointcut 3134 * invocations of a prefetch request/response.</li> 3135 * <li> "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for</li> 3136 * <li> "java.lang.Exception" - The exception that caused the failure of the prefetch request</li> 3137 * </ul> 3138 * 3139 * <p> 3140 * Hooks should return <code>void</code>. 3141 * </p> 3142 */ 3143 CDS_HOOK_PREFETCH_FAILED( 3144 void.class, 3145 "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson", 3146 "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson", 3147 "java.lang.Exception"), 3148 3149 /** 3150 * This pointcut is used only for unit tests. Do not use in production code as it may be changed or 3151 * removed at any time. 3152 */ 3153 TEST_RB( 3154 boolean.class, 3155 new ExceptionHandlingSpec().addLogAndSwallow(IllegalStateException.class), 3156 String.class.getName(), 3157 String.class.getName()), 3158 3159 /** 3160 * This pointcut is used only for unit tests. Do not use in production code as it may be changed or 3161 * removed at any time. 3162 */ 3163 TEST_RO(BaseServerResponseException.class, String.class.getName(), String.class.getName()); 3164 3165 private final List<String> myParameterTypes; 3166 private final Class<?> myReturnType; 3167 private final ExceptionHandlingSpec myExceptionHandlingSpec; 3168 3169 Pointcut(@Nonnull String theReturnType, String... theParameterTypes) { 3170 this(toReturnTypeClass(theReturnType), new ExceptionHandlingSpec(), theParameterTypes); 3171 } 3172 3173 Pointcut( 3174 @Nonnull Class<?> theReturnType, 3175 @Nonnull ExceptionHandlingSpec theExceptionHandlingSpec, 3176 String... theParameterTypes) { 3177 3178 // This enum uses the lowercase-b boolean type to indicate boolean return pointcuts 3179 Validate.isTrue(!theReturnType.equals(Boolean.class), "Return type Boolean not allowed here, must be boolean"); 3180 3181 myReturnType = theReturnType; 3182 myExceptionHandlingSpec = theExceptionHandlingSpec; 3183 myParameterTypes = Collections.unmodifiableList(Arrays.asList(theParameterTypes)); 3184 } 3185 3186 Pointcut(@Nonnull Class<?> theReturnType, String... theParameterTypes) { 3187 this(theReturnType, new ExceptionHandlingSpec(), theParameterTypes); 3188 } 3189 3190 @Override 3191 public boolean isShouldLogAndSwallowException(@Nonnull Throwable theException) { 3192 for (Class<? extends Throwable> next : myExceptionHandlingSpec.myTypesToLogAndSwallow) { 3193 if (next.isAssignableFrom(theException.getClass())) { 3194 return true; 3195 } 3196 } 3197 return false; 3198 } 3199 3200 @Override 3201 @Nonnull 3202 public Class<?> getReturnType() { 3203 return myReturnType; 3204 } 3205 3206 @Override 3207 public Class<?> getBooleanReturnTypeForEnum() { 3208 return boolean.class; 3209 } 3210 3211 @Override 3212 @Nonnull 3213 public List<String> getParameterTypes() { 3214 return myParameterTypes; 3215 } 3216 3217 private static class UnknownType {} 3218 3219 private static class ExceptionHandlingSpec { 3220 3221 private final Set<Class<? extends Throwable>> myTypesToLogAndSwallow = new HashSet<>(); 3222 3223 ExceptionHandlingSpec addLogAndSwallow(@Nonnull Class<? extends Throwable> theType) { 3224 myTypesToLogAndSwallow.add(theType); 3225 return this; 3226 } 3227 } 3228 3229 private static Class<?> toReturnTypeClass(String theReturnType) { 3230 try { 3231 return Class.forName(theReturnType); 3232 } catch (ClassNotFoundException theE) { 3233 return UnknownType.class; 3234 } 3235 } 3236}