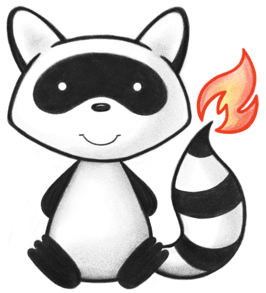
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.primitive.IdDt; 024import ca.uhn.fhir.parser.DataFormatException; 025import ca.uhn.fhir.util.CoverageIgnore; 026 027public abstract class BaseIdentifiableElement extends BaseElement implements IIdentifiableElement { 028 029 private static final long serialVersionUID = -7816838417076777914L; 030 private String myElementSpecificId; 031 032 @Override 033 public String getElementSpecificId() { 034 return myElementSpecificId; 035 } 036 037 /** 038 * @deprecated Use {@link #getElementSpecificId()} instead. This method will be removed because it is easily 039 * confused with other ID methods (such as patient#getIdentifier) 040 */ 041 @CoverageIgnore 042 @Deprecated 043 @Override 044 public IdDt getId() { 045 if (myElementSpecificId == null) { 046 return new LockedId(); 047 } 048 return new LockedId(myElementSpecificId); 049 } 050 051 @Override 052 public void setElementSpecificId(String theElementSpecificId) { 053 myElementSpecificId = theElementSpecificId; 054 } 055 056 /** 057 * @deprecated Use {@link #setElementSpecificId(String)} instead. This method will be removed because it is easily 058 * confused with other ID methods (such as patient#getIdentifier) 059 */ 060 @CoverageIgnore 061 @Deprecated 062 @Override 063 public void setId(IdDt theId) { 064 if (theId == null) { 065 myElementSpecificId = null; 066 } else { 067 myElementSpecificId = theId.getValue(); 068 } 069 } 070 071 /** 072 * @deprecated Use {@link #setElementSpecificId(String)} instead. This method will be removed because it is easily 073 * confused with other ID methods (such as patient#getIdentifier) 074 */ 075 @CoverageIgnore 076 @Override 077 @Deprecated 078 public void setId(String theId) { 079 myElementSpecificId = theId; 080 } 081 082 @CoverageIgnore 083 private static class LockedId extends IdDt { 084 085 @CoverageIgnore 086 public LockedId() {} 087 088 @CoverageIgnore 089 public LockedId(String theElementSpecificId) { 090 super(theElementSpecificId); 091 } 092 093 @Override 094 @CoverageIgnore 095 public IdDt setValue(String theValue) throws DataFormatException { 096 throw new UnsupportedOperationException( 097 Msg.code(1899) + "Use IElement#setElementSpecificId(String) to set the element ID for an element"); 098 } 099 100 @Override 101 @CoverageIgnore 102 public void setValueAsString(String theValue) throws DataFormatException { 103 throw new UnsupportedOperationException( 104 Msg.code(1900) + "Use IElement#setElementSpecificId(String) to set the element ID for an element"); 105 } 106 } 107}