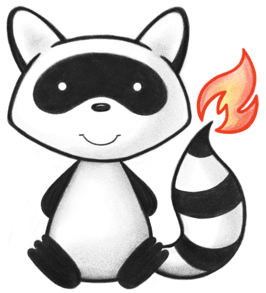
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import ca.uhn.fhir.context.FhirContext; 023 024import java.io.Serializable; 025 026public interface IQueryParameterType extends Serializable { 027 028 /** 029 * This method is generally only called by HAPI itself, and should not need to be called from user code. 030 * 031 * <p> 032 * See FHIR specification <a href="http://www.hl7.org/implement/standards/fhir/search.html#ptypes">2.2.2 Search 033 * SearchParameter Types</a> for information on the <b>token</b> format 034 * </p> 035 * @param theContext TODO 036 * @param theParamName TODO 037 * @param theQualifier 038 * The parameter name qualifier that accompanied this value. For example, if the complete query was 039 * <code>http://foo?name:exact=John</code>, qualifier would be ":exact" 040 * @param theValue 041 * The actual parameter value. For example, if the complete query was 042 * <code>http://foo?name:exact=John</code>, the value would be "John" 043 */ 044 public void setValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue); 045 046 /** 047 * Returns a representation of this parameter's value as it will be represented "over the wire". In other 048 * words, how it will be presented in a URL (although not URL escaped) 049 * 050 * <p> 051 * See FHIR specification <a href="http://www.hl7.org/implement/standards/fhir/search.html#ptypes">2.2.2 Search 052 * SearchParameter Types</a> for information on the <b>token</b> format 053 * </p> 054 * @param theContext TODO 055 * 056 * @return Returns a representation of this parameter's value as it will be represented "over the wire". In other 057 * words, how it will be presented in a URL (although not URL escaped) 058 */ 059 public String getValueAsQueryToken(FhirContext theContext); 060 061 /** 062 * This method will return any qualifier that should be appended to the parameter name (e.g ":exact"). Returns null if none are present. 063 */ 064 public String getQueryParameterQualifier(); 065 066 /** 067 * If set to non-null value, indicates that this parameter has been populated with a "[name]:missing=true" or "[name]:missing=false" vale 068 * instead of a normal value 069 */ 070 Boolean getMissing(); 071 072 /** 073 * If set to non-null value, indicates that this parameter has been populated with a "[name]:missing=true" or "[name]:missing=false" vale 074 * instead of a normal value 075 * 076 * @return Returns a reference to <code>this</code> for easier method chaining 077 */ 078 IQueryParameterType setMissing(Boolean theMissing); 079}