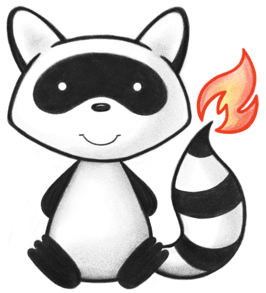
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import ca.uhn.fhir.model.api.annotation.ResourceDef; 023import ca.uhn.fhir.model.base.composite.BaseContainedDt; 024import ca.uhn.fhir.model.base.composite.BaseNarrativeDt; 025import ca.uhn.fhir.model.base.resource.ResourceMetadataMap; 026import ca.uhn.fhir.model.primitive.CodeDt; 027import ca.uhn.fhir.model.primitive.IdDt; 028import org.hl7.fhir.instance.model.api.IBaseMetaType; 029 030/** 031 * This interface is the parent interface for all FHIR Resource definition classes. Classes implementing this interface should be annotated with the {@link ResourceDef @ResourceDef} annotation. 032 * 033 * <p> 034 * Note that this class is a part of HAPI's model API, used to define structure classes. Users will often interact with this interface, but should not need to implement it directly. 035 * </p> 036 */ 037public interface IResource extends ICompositeElement, org.hl7.fhir.instance.model.api.IBaseResource { 038 039 /** 040 * Returns the contained resource list for this resource. 041 * <p> 042 * Usage note: HAPI will generally populate and use the resources from this list automatically (placing inline resources in the contained list when encoding, and copying contained resources from 043 * this list to their appropriate references when parsing) so it is generally not neccesary to interact with this list directly. Instead, in a server you can place resource instances in reference 044 * fields (such as <code>Patient#setManagingOrganization(ResourceReferenceDt)</code> ) and the resource will be automatically contained. In a client, contained resources will be automatically 045 * populated into their appropriate fields by the HAPI parser. 046 * </p> 047 * TODO: document contained resources and link there 048 */ 049 BaseContainedDt getContained(); 050 051 /** 052 * Returns the ID of this resource. Note that this identifier is the URL (or a portion of the URL) used to access this resource, and is not the same thing as any business identifiers stored within 053 * the resource. For example, a Patient resource might have any number of medical record numbers but these are not stored here. 054 * <p> 055 * This ID is specified as the "Logical ID" and "Version ID" in the FHIR specification, see <a href="http://www.hl7.org/implement/standards/fhir/resources.html#metadata">here</a> 056 * </p> 057 */ 058 IdDt getId(); 059 060 /** 061 * Gets the language of the resource itself - <b>NOTE that this language attribute applies to the resource itself, it is not (for example) the language spoken by a practitioner or patient</b> 062 */ 063 CodeDt getLanguage(); 064 065 /** 066 * Returns a view of the {@link #getResourceMetadata() resource metadata} map. 067 * Note that getters from this map return immutable objects, but the <code>addFoo()</code> 068 * and <code>setFoo()</code> methods may be used to modify metadata. 069 * 070 * @since 1.5 071 */ 072 @Override 073 IBaseMetaType getMeta(); 074 075 /** 076 * Returns the metadata map for this object, creating it if neccesary. Metadata entries are used to get/set feed bundle entries, such as the resource version, or the last updated timestamp. 077 * <p> 078 * Keys in this map are enumerated in the {@link ResourceMetadataKeyEnum}, and each key has a specific value type that it must use. 079 * </p> 080 * 081 * @see ResourceMetadataKeyEnum for a list of allowable keys and the object types that values of a given key must use. 082 */ 083 ResourceMetadataMap getResourceMetadata(); 084 085 /** 086 * Returns a String representing the name of this Resource. This return value is not used for anything by HAPI itself, but is provided as a convenience to developers using the API. 087 * 088 * @return the name of this resource, e.g. "Patient", or "Observation" 089 */ 090 String getResourceName(); 091 092 /** 093 * Returns the FHIR version represented by this structure 094 */ 095 @Override 096 public ca.uhn.fhir.context.FhirVersionEnum getStructureFhirVersionEnum(); 097 098 /** 099 * Returns the narrative block for this resource 100 */ 101 BaseNarrativeDt<?> getText(); 102 103 /** 104 * Sets the ID of this resource. Note that this identifier is the URL (or a portion of the URL) used to access this resource, and is not the same thing as any business identifiers stored within the 105 * resource. For example, a Patient resource might have any number of medical record numbers but these are not stored here. 106 * <p> 107 * This ID is specified as the "Logical ID" and "Version ID" in the FHIR specification, see <a href="http://www.hl7.org/implement/standards/fhir/resources.html#metadata">here</a> 108 * </p> 109 */ 110 void setId(IdDt theId); 111 112 /** 113 * Sets the language of the resource itself - <b>NOTE that this language attribute applies to the resource itself, it is not (for example) the language spoken by a practitioner or patient</b> 114 */ 115 void setLanguage(CodeDt theLanguage); 116 117 /** 118 * Sets the metadata map for this object. Metadata entries are used to get/set feed bundle entries, such as the resource version, or the last updated timestamp. 119 * 120 * @throws NullPointerException 121 * The map must not be null 122 */ 123 void setResourceMetadata(ResourceMetadataMap theMap); 124}