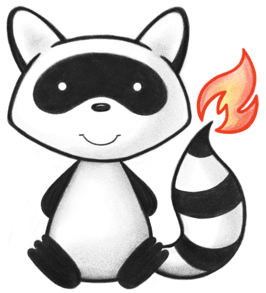
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import org.hl7.fhir.instance.model.api.IBaseDatatype; 023 024import java.util.List; 025 026public interface ISupportsUndeclaredExtensions extends IElement { 027 028 /** 029 * Returns a list containing all undeclared non-modifier extensions. The returned list 030 * is mutable, so it may be modified (e.g. to add or remove an extension). 031 */ 032 List<ExtensionDt> getUndeclaredExtensions(); 033 034 /** 035 * Returns an <b>immutable</b> list containing all undeclared extensions (modifier and non-modifier) by extension URL 036 * 037 * @see #getUndeclaredExtensions() To return a mutable list which may be used to remove extensions 038 */ 039 List<ExtensionDt> getUndeclaredExtensionsByUrl(String theUrl); 040 041 /** 042 * Returns an <b>immutable</b> list containing all extensions (modifier and non-modifier). 043 * 044 * @see #getUndeclaredExtensions() To return a mutable list which may be used to remove undeclared non-modifier extensions 045 * @see #getUndeclaredModifierExtensions() To return a mutable list which may be used to remove undeclared modifier extensions 046 */ 047 List<ExtensionDt> getAllUndeclaredExtensions(); 048 049 /** 050 * Returns a list containing all undeclared modifier extensions. The returned list 051 * is mutable, so it may be modified (e.g. to add or remove an extension). 052 */ 053 List<ExtensionDt> getUndeclaredModifierExtensions(); 054 055 /** 056 * Adds an extension to this object. This extension should have the 057 * following properties set: 058 * <ul> 059 * <li>{@link ExtensionDt#setModifier(boolean) Is Modifier}</li> 060 * <li>{@link ExtensionDt#setUrl(String) URL}</li> 061 * <li>And one of: 062 * <ul> 063 * <li>{@link ExtensionDt#setValue(IBaseDatatype) A datatype value}</li> 064 * <li>{@link #addUndeclaredExtension(ExtensionDt) Further sub-extensions}</li> 065 * </ul> 066 * </ul> 067 * 068 * @param theExtension The extension to add. Can not be null. 069 */ 070 void addUndeclaredExtension(ExtensionDt theExtension); 071 072 /** 073 * Adds an extension to this object 074 * 075 * @see #getUndeclaredExtensions() To return a mutable list which may be used to remove extensions 076 */ 077 ExtensionDt addUndeclaredExtension(boolean theIsModifier, String theUrl, IBaseDatatype theValue); 078 079 /** 080 * Adds an extension to this object. This method is intended for use when 081 * an extension is being added which will contain child extensions, as opposed to 082 * a datatype. 083 * 084 * @see #getUndeclaredExtensions() To return a mutable list which may be used to remove extensions 085 */ 086 ExtensionDt addUndeclaredExtension(boolean theIsModifier, String theUrl); 087}