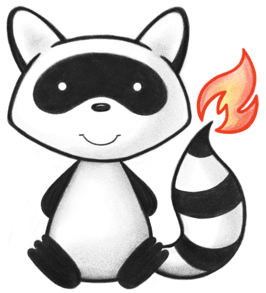
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022/** 023 * This enum contains the allowable codes in the HAPI FHIR defined 024 * codesystem: https://hapifhir.io/fhir/CodeSystem/hapi-fhir-storage-response-code 025 * 026 * This is used in CRUD response OperationOutcome resources. 027 */ 028public enum StorageResponseCodeEnum implements ICodingEnum { 029 SUCCESSFUL_CREATE("Create succeeded."), 030 SUCCESSFUL_CREATE_NO_CONDITIONAL_MATCH( 031 "Conditional create succeeded: no existing resource matched the conditional URL."), 032 SUCCESSFUL_CREATE_WITH_CONDITIONAL_MATCH( 033 "Conditional create succeeded: an existing resource matched the conditional URL so no action was taken."), 034 SUCCESSFUL_UPDATE("Update succeeded."), 035 SUCCESSFUL_UPDATE_AS_CREATE("Update as create succeeded."), 036 SUCCESSFUL_UPDATE_NO_CHANGE("Update succeeded: No changes were detected so no action was taken."), 037 SUCCESSFUL_UPDATE_NO_CONDITIONAL_MATCH( 038 "Conditional update succeeded: no existing resource matched the conditional URL so a new resource was created."), 039 SUCCESSFUL_UPDATE_WITH_CONDITIONAL_MATCH( 040 "Conditional update succeeded: an existing resource matched the conditional URL and was updated."), 041 SUCCESSFUL_UPDATE_WITH_CONDITIONAL_MATCH_NO_CHANGE( 042 "Conditional update succeeded: an existing resource matched the conditional URL and was updated, but no changes were detected so no action was taken."), 043 SUCCESSFUL_DELETE("Delete succeeded."), 044 SUCCESSFUL_DELETE_ALREADY_DELETED("Delete succeeded: Resource was already deleted so no action was taken."), 045 SUCCESSFUL_DELETE_NOT_FOUND("Delete succeeded: No existing resource was found so no action was taken."), 046 047 SUCCESSFUL_PATCH("Patch succeeded."), 048 049 SUCCESSFUL_PATCH_NO_CHANGE("Patch succeeded: No changes were detected so no action was taken."), 050 SUCCESSFUL_CONDITIONAL_PATCH("Conditional patch succeeded."), 051 SUCCESSFUL_CONDITIONAL_PATCH_NO_CHANGE( 052 "Conditional patch succeeded: No changes were detected so no action was taken."), 053 AUTOMATICALLY_CREATED_PLACEHOLDER_RESOURCE("Automatically created placeholder resource."), 054 FAILURE("Failed to process resource."), 055 ; 056 057 public static final String SYSTEM = "https://hapifhir.io/fhir/CodeSystem/hapi-fhir-storage-response-code"; 058 059 private final String myDisplay; 060 061 StorageResponseCodeEnum(String theDisplay) { 062 myDisplay = theDisplay; 063 } 064 065 @Override 066 public String getCode() { 067 return name(); 068 } 069 070 @Override 071 public String getSystem() { 072 return SYSTEM; 073 } 074 075 @Override 076 public String getDisplay() { 077 return myDisplay; 078 } 079}