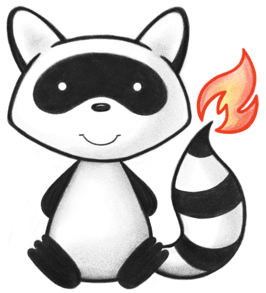
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import org.apache.commons.lang3.StringUtils; 023import org.apache.commons.lang3.builder.ToStringBuilder; 024import org.apache.commons.lang3.builder.ToStringStyle; 025import org.hl7.fhir.instance.model.api.IBaseCoding; 026 027import java.net.URI; 028import java.util.Objects; 029 030/** 031 * A single tag 032 * <p> 033 * Note on equality- When computing hashCode or equals values for this class, only the 034 * {@link #getScheme() scheme} and 035 * </p> 036 */ 037public class Tag extends BaseElement implements IElement, IBaseCoding { 038 039 private static final long serialVersionUID = 1L; 040 041 public static final String ATTR_LABEL = "label"; 042 public static final String ATTR_SCHEME = "scheme"; 043 public static final String ATTR_TERM = "term"; 044 045 /** 046 * Convenience constant containing the "http://hl7.org/fhir/tag" scheme value 047 */ 048 public static final String HL7_ORG_FHIR_TAG = "http://hl7.org/fhir/tag"; 049 /** 050 * Convenience constant containing the "http://hl7.org/fhir/tag/profile" scheme value 051 */ 052 public static final String HL7_ORG_PROFILE_TAG = "http://hl7.org/fhir/tag/profile"; 053 /** 054 * Convenience constant containing the "http://hl7.org/fhir/tag/security" scheme value 055 */ 056 public static final String HL7_ORG_SECURITY_TAG = "http://hl7.org/fhir/tag/security"; 057 058 private String myLabel; 059 private String myScheme; 060 private String myTerm; 061 private String myVersion; 062 private Boolean myUserSelected; 063 064 public Tag() {} 065 066 /** 067 * @deprecated There is no reason to create a tag with a term and not a scheme, so this constructor will be removed 068 */ 069 @Deprecated 070 public Tag(String theTerm) { 071 this((String) null, theTerm, null); 072 } 073 074 public Tag(String theScheme, String theTerm) { 075 myScheme = theScheme; 076 myTerm = theTerm; 077 } 078 079 public Tag(String theScheme, String theTerm, String theLabel) { 080 myTerm = theTerm; 081 myLabel = theLabel; 082 myScheme = theScheme; 083 } 084 085 public Tag(URI theScheme, URI theTerm, String theLabel) { 086 if (theScheme != null) { 087 myScheme = theScheme.toASCIIString(); 088 } 089 if (theTerm != null) { 090 myTerm = theTerm.toASCIIString(); 091 } 092 myLabel = theLabel; 093 } 094 095 public String getLabel() { 096 return myLabel; 097 } 098 099 public String getScheme() { 100 return myScheme; 101 } 102 103 public String getTerm() { 104 return myTerm; 105 } 106 107 @Override 108 public boolean equals(Object obj) { 109 if (this == obj) return true; 110 if (obj == null) return false; 111 if (getClass() != obj.getClass()) return false; 112 Tag other = (Tag) obj; 113 114 return Objects.equals(myScheme, other.myScheme) 115 && Objects.equals(myTerm, other.myTerm) 116 && Objects.equals(myVersion, other.myVersion) 117 && Objects.equals(myUserSelected, other.myUserSelected); 118 } 119 120 @Override 121 public int hashCode() { 122 final int prime = 31; 123 int result = 1; 124 result = prime * result + Objects.hashCode(myScheme); 125 result = prime * result + Objects.hashCode(myTerm); 126 result = prime * result + Objects.hashCode(myVersion); 127 result = prime * result + Objects.hashCode(myUserSelected); 128 return result; 129 } 130 131 /** 132 * Returns <code>true</code> if either scheme or term is populated. 133 */ 134 @Override 135 public boolean isEmpty() { 136 return StringUtils.isBlank(myScheme) && StringUtils.isBlank(myTerm); 137 } 138 139 /** 140 * Sets the label and returns a reference to this tag 141 */ 142 public Tag setLabel(String theLabel) { 143 myLabel = theLabel; 144 return this; 145 } 146 147 /** 148 * Sets the scheme and returns a reference to this tag 149 */ 150 public Tag setScheme(String theScheme) { 151 myScheme = theScheme; 152 return this; 153 } 154 155 /** 156 * Sets the term and returns a reference to this tag 157 */ 158 public Tag setTerm(String theTerm) { 159 myTerm = theTerm; 160 return this; 161 } 162 163 @Override 164 public String toString() { 165 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 166 b.append("Scheme", myScheme); 167 b.append("Term", myTerm); 168 b.append("Label", myLabel); 169 b.append("Version", myVersion); 170 b.append("UserSelected", myUserSelected); 171 return b.toString(); 172 } 173 174 @Override 175 public String getCode() { 176 return getTerm(); 177 } 178 179 @Override 180 public String getDisplay() { 181 return getLabel(); 182 } 183 184 @Override 185 public String getSystem() { 186 return getScheme(); 187 } 188 189 @Override 190 public IBaseCoding setCode(String theTerm) { 191 setTerm(theTerm); 192 return this; 193 } 194 195 @Override 196 public IBaseCoding setDisplay(String theLabel) { 197 setLabel(theLabel); 198 return this; 199 } 200 201 @Override 202 public IBaseCoding setSystem(String theScheme) { 203 setScheme(theScheme); 204 return this; 205 } 206 207 @Override 208 public String getVersion() { 209 return myVersion; 210 } 211 212 @Override 213 public IBaseCoding setVersion(String theVersion) { 214 myVersion = theVersion; 215 return this; 216 } 217 218 @Override 219 public boolean getUserSelected() { 220 return myUserSelected != null && myUserSelected; 221 } 222 223 public Boolean getUserSelectedBoolean() { 224 return myUserSelected; 225 } 226 227 @Override 228 public IBaseCoding setUserSelected(boolean theUserSelected) { 229 myUserSelected = theUserSelected; 230 return this; 231 } 232 233 public void setUserSelectedBoolean(Boolean theUserSelected) { 234 myUserSelected = theUserSelected; 235 } 236}