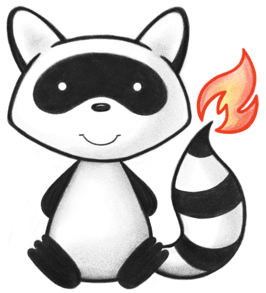
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api; 021 022import org.apache.commons.lang3.time.DateUtils; 023 024import java.util.Calendar; 025import java.util.Date; 026 027public enum TemporalPrecisionEnum { 028 YEAR(Calendar.YEAR) { 029 @Override 030 public Date add(Date theInput, int theAmount) { 031 return DateUtils.addYears(theInput, theAmount); 032 } 033 }, 034 035 MONTH(Calendar.MONTH) { 036 @Override 037 public Date add(Date theInput, int theAmount) { 038 return DateUtils.addMonths(theInput, theAmount); 039 } 040 }, 041 DAY(Calendar.DATE) { 042 @Override 043 public Date add(Date theInput, int theAmount) { 044 return DateUtils.addDays(theInput, theAmount); 045 } 046 }, 047 MINUTE(Calendar.MINUTE) { 048 @Override 049 public Date add(Date theInput, int theAmount) { 050 return DateUtils.addMinutes(theInput, theAmount); 051 } 052 }, 053 SECOND(Calendar.SECOND) { 054 @Override 055 public Date add(Date theInput, int theAmount) { 056 return DateUtils.addSeconds(theInput, theAmount); 057 } 058 }, 059 060 MILLI(Calendar.MILLISECOND) { 061 @Override 062 public Date add(Date theInput, int theAmount) { 063 return DateUtils.addMilliseconds(theInput, theAmount); 064 } 065 }, 066 ; 067 068 private int myCalendarConstant; 069 070 TemporalPrecisionEnum(int theCalendarConstant) { 071 myCalendarConstant = theCalendarConstant; 072 } 073 074 public abstract Date add(Date theInput, int theAmount); 075 076 public int getCalendarConstant() { 077 return myCalendarConstant; 078 } 079 080 /** 081 * Given the standard string representation - YYYY-DD-MMTHH:NN:SS.SSS - how long is the string for the stated precision? 082 */ 083 public int stringLength() { 084 switch (this) { 085 case YEAR: 086 return 4; 087 case MONTH: 088 return 7; 089 case DAY: 090 return 10; 091 case MINUTE: 092 return 16; 093 case SECOND: 094 return 19; 095 case MILLI: 096 return 23; 097 } 098 return 0; // ?? 099 } 100}