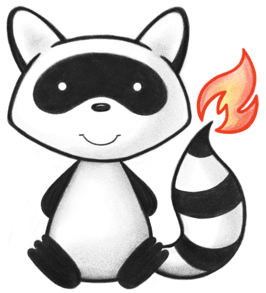
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.api.annotation; 021 022import java.lang.annotation.ElementType; 023import java.lang.annotation.Inherited; 024import java.lang.annotation.Retention; 025import java.lang.annotation.RetentionPolicy; 026import java.lang.annotation.Target; 027 028/** 029 * Class annotation which indicates a resource definition class 030 */ 031@Inherited 032@Retention(RetentionPolicy.RUNTIME) 033@Target(value = {ElementType.TYPE}) 034public @interface ResourceDef { 035 036 /** 037 * The name of the resource (e.g. "Patient" or "DiagnosticReport"). If you are defining your 038 * own custom extension to a built-in FHIR resource definition type (e.g. you are extending 039 * the built-in Patient class) you do not need to supply a value for this property, as it 040 * will be inferred from the parent class. 041 */ 042 String name() default ""; 043 044 /** 045 * if set, will be used as the id for any profiles generated for this resource. This property 046 * should be set for custom profile definition classes, and will be used as the resource ID 047 * for the generated profile exported by the server. For example, if you set this value to 048 * "hello" on a custom resource class, your server will automatically export a profile with the 049 * identity: <code>http://localhost:8080/fhir/Profile/hello</code> (the base URL will be whatever 050 * your server uses, not necessarily "http://localhost:8080/fhir") 051 */ 052 String id() default ""; 053 054 /** 055 * The URL indicating the profile for this resource definition. If specified, this URL will be 056 * automatically added to the meta tag when the resource is serialized. 057 * <p> 058 * This URL should be fully qualified to indicate the complete URL of 059 * the profile being used, e.g. <code>http://example.com/fhir/StructureDefiniton/some_profile</code> 060 * </p> 061 */ 062 String profile() default ""; 063}