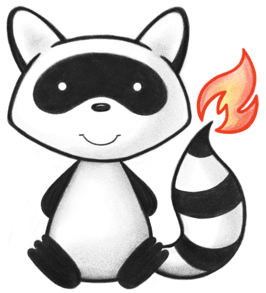
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.base.composite; 021 022import ca.uhn.fhir.model.api.BaseIdentifiableElement; 023import ca.uhn.fhir.model.primitive.StringDt; 024import ca.uhn.fhir.util.DatatypeUtil; 025import org.apache.commons.lang3.builder.ToStringBuilder; 026import org.apache.commons.lang3.builder.ToStringStyle; 027 028import java.util.ArrayList; 029import java.util.List; 030 031public abstract class BaseHumanNameDt extends BaseIdentifiableElement { 032 033 private static final long serialVersionUID = 2765500013165698259L; 034 035 /** 036 * Gets the value(s) for <b>family</b> (Family name (often called 'Surname')). creating it if it does not exist. Will not return <code>null</code>. 037 * 038 * <p> 039 * <b>Definition:</b> The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 040 * </p> 041 */ 042 public abstract java.util.List<StringDt> getFamily(); 043 044 /** 045 * Returns all repetitions of {@link #getFamily() family name} as a space separated string 046 * 047 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 048 */ 049 public String getFamilyAsSingleString() { 050 return ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated(getFamily()); 051 } 052 053 /** 054 * Gets the value(s) for <b>given</b> (Given names (not always 'first'). Includes middle names). creating it if it does not exist. Will not return <code>null</code>. 055 * 056 * <p> 057 * <b>Definition:</b> Given name 058 * </p> 059 */ 060 public abstract java.util.List<StringDt> getGiven(); 061 062 /** 063 * Returns all repetitions of {@link #getGiven() given name} as a space separated string 064 * 065 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 066 */ 067 public String getGivenAsSingleString() { 068 return ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated(getGiven()); 069 } 070 071 /** 072 * Gets the value(s) for <b>prefix</b> (Parts that come before the name). creating it if it does not exist. Will not return <code>null</code>. 073 * 074 * <p> 075 * <b>Definition:</b> Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 076 * </p> 077 */ 078 public abstract java.util.List<StringDt> getPrefix(); 079 080 /** 081 * Returns all repetitions of {@link #getPrefix() prefix name} as a space separated string 082 * 083 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 084 */ 085 public String getPrefixAsSingleString() { 086 return ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated(getPrefix()); 087 } 088 089 /** 090 * Gets the value(s) for <b>suffix</b> (Parts that come after the name). creating it if it does not exist. Will not return <code>null</code>. 091 * 092 * <p> 093 * <b>Definition:</b> Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 094 * </p> 095 */ 096 public abstract java.util.List<StringDt> getSuffix(); 097 098 /** 099 * Returns all repetitions of {@link #getSuffix() suffix} as a space separated string 100 * 101 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 102 */ 103 public String getSuffixAsSingleString() { 104 return ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated(getSuffix()); 105 } 106 107 /** 108 * Gets the value(s) for <b>text</b> (Text representation of the full name). creating it if it does not exist. Will not return <code>null</code>. 109 * 110 * <p> 111 * <b>Definition:</b> A full text representation of the name 112 * </p> 113 */ 114 public abstract StringDt getTextElement(); 115 116 /** 117 * Sets the value(s) for <b>text</b> (Text representation of the full name) 118 * 119 * <p> 120 * <b>Definition:</b> A full text representation of the name 121 * </p> 122 */ 123 public abstract BaseHumanNameDt setText(StringDt theValue); 124 125 @Override 126 public String toString() { 127 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 128 b.append("family", getFamilyAsSingleString()); 129 b.append("given", getGivenAsSingleString()); 130 return b.toString(); 131 } 132 133 /** 134 * Returns all of the components of the name (prefix, given, family, suffix) as a 135 * single string with a single spaced string separating each part. 136 * <p> 137 * If none of the parts are populated, returns the {@link #getTextElement() text} 138 * element value instead. 139 * </p> 140 */ 141 public String getNameAsSingleString() { 142 List<StringDt> nameParts = new ArrayList<StringDt>(); 143 nameParts.addAll(getPrefix()); 144 nameParts.addAll(getGiven()); 145 nameParts.addAll(getFamily()); 146 nameParts.addAll(getSuffix()); 147 if (nameParts.size() > 0) { 148 return ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated(nameParts); 149 } 150 return getTextElement().getValue(); 151 } 152}