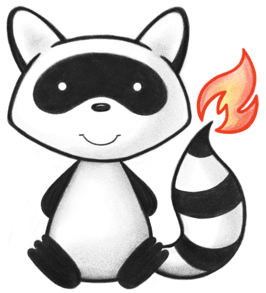
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.base.composite; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.BaseIdentifiableElement; 025import ca.uhn.fhir.model.api.ICompositeDatatype; 026import ca.uhn.fhir.model.api.IQueryParameterType; 027import ca.uhn.fhir.model.primitive.BoundCodeDt; 028import ca.uhn.fhir.model.primitive.CodeDt; 029import ca.uhn.fhir.model.primitive.DecimalDt; 030import ca.uhn.fhir.model.primitive.StringDt; 031import ca.uhn.fhir.model.primitive.UriDt; 032import ca.uhn.fhir.rest.param.ParamPrefixEnum; 033import ca.uhn.fhir.rest.param.QuantityParam; 034import org.apache.commons.lang3.StringUtils; 035 036import java.math.BigDecimal; 037 038public abstract class BaseQuantityDt extends BaseIdentifiableElement 039 implements ICompositeDatatype, IQueryParameterType { 040 041 private static final long serialVersionUID = 1L; 042 043 /** 044 * Sets the value(s) for <b>value</b> (Numerical value (with implicit precision)) 045 * 046 * <p> 047 * <b>Definition:</b> 048 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 049 * </p> 050 */ 051 public abstract BaseQuantityDt setValue(BigDecimal theValue); 052 053 @Override 054 public void setValueAsQueryToken( 055 FhirContext theContext, String theParamName, String theQualifier, String theValue) { 056 getComparatorElement().setValue(null); 057 setCode(null); 058 setSystem(null); 059 setUnits(null); 060 setValue(null); 061 062 if (theValue == null) { 063 return; 064 } 065 String[] parts = theValue.split("\\|"); 066 if (parts.length > 0 && StringUtils.isNotBlank(parts[0])) { 067 if (parts[0].startsWith("le")) { 068 // TODO: Use of a deprecated method should be resolved. 069 getComparatorElement().setValue(ParamPrefixEnum.LESSTHAN_OR_EQUALS.getValue()); 070 setValue(new BigDecimal(parts[0].substring(2))); 071 } else if (parts[0].startsWith("lt")) { 072 // TODO: Use of a deprecated method should be resolved. 073 getComparatorElement().setValue(ParamPrefixEnum.LESSTHAN.getValue()); 074 setValue(new BigDecimal(parts[0].substring(1))); 075 } else if (parts[0].startsWith("ge")) { 076 // TODO: Use of a deprecated method should be resolved. 077 getComparatorElement().setValue(ParamPrefixEnum.GREATERTHAN_OR_EQUALS.getValue()); 078 setValue(new BigDecimal(parts[0].substring(2))); 079 } else if (parts[0].startsWith("gt")) { 080 // TODO: Use of a deprecated method should be resolved. 081 getComparatorElement().setValue(ParamPrefixEnum.GREATERTHAN.getValue()); 082 setValue(new BigDecimal(parts[0].substring(1))); 083 } else { 084 setValue(new BigDecimal(parts[0])); 085 } 086 } 087 if (parts.length > 1 && StringUtils.isNotBlank(parts[1])) { 088 setSystem(parts[1]); 089 } 090 if (parts.length > 2 && StringUtils.isNotBlank(parts[2])) { 091 setUnits(parts[2]); 092 } 093 } 094 095 /** 096 * Gets the value(s) for <b>comparator</b> (< | <= | >= | > - how to understand the value). 097 * creating it if it does 098 * not exist. Will not return <code>null</code>. 099 * 100 * <p> 101 * <b>Definition:</b> 102 * How the value should be understood and represented - whether the actual value is greater or less than 103 * the stated value due to measurement issues. E.g. if the comparator is \"<\" , then the real value is < stated value 104 * </p> 105 */ 106 public abstract BoundCodeDt<?> getComparatorElement(); 107 108 @Override 109 public String getValueAsQueryToken(FhirContext theContext) { 110 StringBuilder b = new StringBuilder(); 111 if (getComparatorElement() != null) { 112 b.append(getComparatorElement().getValue()); 113 } 114 if (!getValueElement().isEmpty()) { 115 b.append(getValueElement().getValueAsString()); 116 } 117 b.append('|'); 118 if (!getSystemElement().isEmpty()) { 119 b.append(getSystemElement().getValueAsString()); 120 } 121 b.append('|'); 122 if (!getUnitsElement().isEmpty()) { 123 b.append(getUnitsElement().getValueAsString()); 124 } 125 126 return b.toString(); 127 } 128 129 @Override 130 public String getQueryParameterQualifier() { 131 return null; 132 } 133 134 /** 135 * Sets the value for <b>units</b> (Unit representation) 136 * 137 * <p> 138 * <b>Definition:</b> 139 * A human-readable form of the units 140 * </p> 141 */ 142 public abstract BaseQuantityDt setUnits(String theString); 143 144 /** 145 * Gets the value(s) for <b>system</b> (System that defines coded unit form). 146 * creating it if it does 147 * not exist. Will not return <code>null</code>. 148 * 149 * <p> 150 * <b>Definition:</b> 151 * The identification of the system that provides the coded form of the unit 152 * </p> 153 */ 154 public abstract UriDt getSystemElement(); 155 156 /** 157 * Sets the value for <b>system</b> (System that defines coded unit form) 158 * 159 * <p> 160 * <b>Definition:</b> 161 * The identification of the system that provides the coded form of the unit 162 * </p> 163 */ 164 public abstract BaseQuantityDt setSystem(String theUri); 165 166 /** 167 * Gets the value(s) for <b>code</b> (Coded form of the unit). 168 * creating it if it does 169 * not exist. Will not return <code>null</code>. 170 * 171 * <p> 172 * <b>Definition:</b> 173 * A computer processable form of the units in some unit representation system 174 * </p> 175 */ 176 public abstract CodeDt getCodeElement(); 177 178 /** 179 * Sets the value for <b>code</b> (Coded form of the unit) 180 * 181 * <p> 182 * <b>Definition:</b> 183 * A computer processable form of the units in some unit representation system 184 * </p> 185 */ 186 public abstract BaseQuantityDt setCode(String theCode); 187 /** 188 * Gets the value(s) for <b>units</b> (Unit representation). 189 * creating it if it does 190 * not exist. Will not return <code>null</code>. 191 * 192 * <p> 193 * <b>Definition:</b> 194 * A human-readable form of the units 195 * </p> 196 */ 197 public abstract StringDt getUnitsElement(); 198 /** 199 * Gets the value(s) for <b>value</b> (Numerical value (with implicit precision)). 200 * creating it if it does 201 * not exist. Will not return <code>null</code>. 202 * 203 * <p> 204 * <b>Definition:</b> 205 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 206 * </p> 207 */ 208 public abstract DecimalDt getValueElement(); 209 210 /** 211 * <b>Not supported!</b> 212 * 213 * @deprecated get/setMissing is not supported in StringDt. Use {@link QuantityParam} instead if you 214 * need this functionality 215 */ 216 @Deprecated 217 @Override 218 public Boolean getMissing() { 219 return null; 220 } 221 222 /** 223 * <b>Not supported!</b> 224 * 225 * @deprecated get/setMissing is not supported in StringDt. Use {@link QuantityParam} instead if you 226 * need this functionality 227 */ 228 @Deprecated 229 @Override 230 public IQueryParameterType setMissing(Boolean theMissing) { 231 throw new UnsupportedOperationException( 232 Msg.code(1904) 233 + "get/setMissing is not supported in StringDt. Use {@link StringParam} instead if you need this functionality"); 234 } 235}