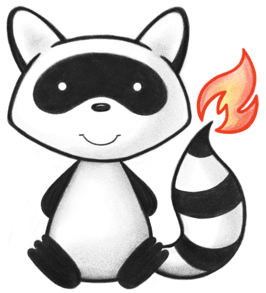
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.model.api.IValueSetEnumBinder; 023import ca.uhn.fhir.model.api.annotation.DatatypeDef; 024import org.apache.commons.lang3.Validate; 025 026import java.io.IOException; 027import java.io.ObjectInput; 028import java.io.ObjectOutput; 029 030@DatatypeDef(name = "code", isSpecialization = true) 031public class BoundCodeDt<T extends Enum<?>> extends CodeDt { 032 033 private IValueSetEnumBinder<T> myBinder; 034 035 /** 036 * @deprecated This constructor is provided only for serialization support. Do not call it directly! 037 */ 038 @Deprecated 039 public BoundCodeDt() { 040 // nothing 041 } 042 043 public BoundCodeDt(IValueSetEnumBinder<T> theBinder) { 044 Validate.notNull(theBinder, "theBinder must not be null"); 045 myBinder = theBinder; 046 } 047 048 public BoundCodeDt(IValueSetEnumBinder<T> theBinder, T theValue) { 049 Validate.notNull(theBinder, "theBinder must not be null"); 050 myBinder = theBinder; 051 setValueAsEnum(theValue); 052 } 053 054 public IValueSetEnumBinder<T> getBinder() { 055 return myBinder; 056 } 057 058 public T getValueAsEnum() { 059 Validate.notNull( 060 myBinder, "This object does not have a binder. Constructor BoundCodeDt() should not be called!"); 061 T retVal = myBinder.fromCodeString(getValue()); 062 if (retVal == null) { 063 // TODO: throw special exception type? 064 } 065 return retVal; 066 } 067 068 @SuppressWarnings("unchecked") 069 @Override 070 public void readExternal(ObjectInput theIn) throws IOException, ClassNotFoundException { 071 super.readExternal(theIn); 072 myBinder = (IValueSetEnumBinder<T>) theIn.readObject(); 073 } 074 075 public void setValueAsEnum(T theValue) { 076 Validate.notNull( 077 myBinder, "This object does not have a binder. Constructor BoundCodeDt() should not be called!"); 078 if (theValue == null) { 079 setValue(null); 080 } else { 081 setValue(myBinder.toCodeString(theValue)); 082 } 083 } 084 085 @Override 086 public void writeExternal(ObjectOutput theOut) throws IOException { 087 super.writeExternal(theOut); 088 theOut.writeObject(myBinder); 089 } 090}