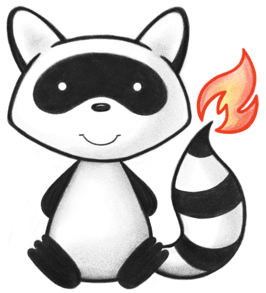
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.model.api.BasePrimitive; 023import ca.uhn.fhir.model.api.annotation.DatatypeDef; 024import ca.uhn.fhir.model.api.annotation.SimpleSetter; 025 026import static org.apache.commons.lang3.StringUtils.defaultString; 027import static org.apache.commons.lang3.StringUtils.isBlank; 028 029@DatatypeDef(name = "code", profileOf = StringDt.class) 030public class CodeDt extends BasePrimitive<String> implements Comparable<CodeDt> { 031 032 /** 033 * Constructor 034 */ 035 public CodeDt() { 036 super(); 037 } 038 039 /** 040 * Constructor which accepts a string code 041 */ 042 @SimpleSetter() 043 public CodeDt(@SimpleSetter.Parameter(name = "theCode") String theCode) { 044 setValue(theCode); 045 } 046 047 @Override 048 public boolean isEmpty() { 049 return super.isBaseEmpty() && isBlank(getValueAsString()); 050 } 051 052 @Override 053 public int compareTo(CodeDt theCode) { 054 if (theCode == null) { 055 return 1; 056 } 057 return defaultString(getValue()).compareTo(defaultString(theCode.getValue())); 058 } 059 060 @Override 061 protected String parse(String theValue) { 062 return theValue.trim(); 063 } 064 065 @Override 066 protected String encode(String theValue) { 067 return theValue; 068 } 069}