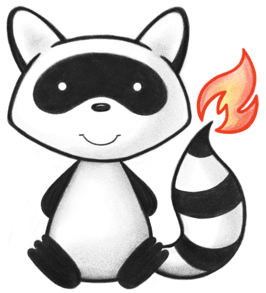
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 023import ca.uhn.fhir.model.api.annotation.DatatypeDef; 024import ca.uhn.fhir.model.api.annotation.SimpleSetter; 025import ca.uhn.fhir.parser.DataFormatException; 026 027import java.util.Calendar; 028import java.util.Date; 029import java.util.GregorianCalendar; 030import java.util.TimeZone; 031 032/** 033 * Represents a FHIR date datatype. Valid precisions values for this type are: 034 * <ul> 035 * <li>{@link TemporalPrecisionEnum#YEAR} 036 * <li>{@link TemporalPrecisionEnum#MONTH} 037 * <li>{@link TemporalPrecisionEnum#DAY} 038 * </ul> 039 * 040 * <p> 041 * <b>Note on using Java Date objects:</b> This type stores the date as a Java Date. Note that 042 * the Java Date has more precision (millisecond precision), and does not store a timezone. As such, 043 * it could potentially cause issues. For example, if a Date contains the number of milliseconds at 044 * midnight in a timezone across the date line from your location, it might refer to a different date than 045 * intended. 046 * </p> 047 * <p> 048 * As such, it is recommended to use the <code>Calendar<code> or <code>int,int,int</code> constructors 049 * </p> 050 */ 051@DatatypeDef(name = "date") 052public class DateDt extends BaseDateTimeDt { 053 054 /** 055 * The default precision for this type 056 */ 057 public static final TemporalPrecisionEnum DEFAULT_PRECISION = TemporalPrecisionEnum.DAY; 058 059 /** 060 * Constructor 061 */ 062 public DateDt() { 063 super(); 064 } 065 066 /** 067 * Constructor which accepts a date value and uses the {@link #DEFAULT_PRECISION} for this type. 068 */ 069 public DateDt(Calendar theCalendar) { 070 super(theCalendar.getTime(), DEFAULT_PRECISION); 071 setTimeZone(theCalendar.getTimeZone()); 072 } 073 074 /** 075 * Constructor which accepts a date value and uses the {@link #DEFAULT_PRECISION} for this type. 076 * <b>Please see the note on timezones</b> on the {@link DateDt class documentation} for considerations 077 * when using this constructor! 078 */ 079 @SimpleSetter(suffix = "WithDayPrecision") 080 public DateDt(@SimpleSetter.Parameter(name = "theDate") Date theDate) { 081 super(theDate, DEFAULT_PRECISION); 082 } 083 084 /** 085 * Constructor which accepts a date value and a precision value. Valid precisions values for this type are: 086 * <ul> 087 * <li>{@link TemporalPrecisionEnum#YEAR} 088 * <li>{@link TemporalPrecisionEnum#MONTH} 089 * <li>{@link TemporalPrecisionEnum#DAY} 090 * </ul> 091 * <b>Please see the note on timezones</b> on the {@link DateDt class documentation} for considerations 092 * when using this constructor! 093 * 094 * @throws DataFormatException 095 * If the specified precision is not allowed for this type 096 */ 097 @SimpleSetter 098 public DateDt( 099 @SimpleSetter.Parameter(name = "theDate") Date theDate, 100 @SimpleSetter.Parameter(name = "thePrecision") TemporalPrecisionEnum thePrecision) { 101 super(theDate, thePrecision); 102 } 103 104 /** 105 * Constructor which accepts a date value and uses the {@link #DEFAULT_PRECISION} for this type. 106 * 107 * @param theYear The year, e.g. 2015 108 * @param theMonth The month, e.g. 0 for January 109 * @param theDay The day (1 indexed) e.g. 1 for the first day of the month 110 */ 111 public DateDt(int theYear, int theMonth, int theDay) { 112 this(toCalendarZulu(theYear, theMonth, theDay)); 113 } 114 115 /** 116 * Constructor which accepts a date as a string in FHIR format 117 * 118 * @throws DataFormatException 119 * If the precision in the date string is not allowed for this type 120 */ 121 public DateDt(String theDate) { 122 super(theDate); 123 } 124 125 /** 126 * Returns the default precision for this datatype 127 * 128 * @see #DEFAULT_PRECISION 129 */ 130 @Override 131 protected TemporalPrecisionEnum getDefaultPrecisionForDatatype() { 132 return DEFAULT_PRECISION; 133 } 134 135 @Override 136 protected boolean isPrecisionAllowed(TemporalPrecisionEnum thePrecision) { 137 switch (thePrecision) { 138 case YEAR: 139 case MONTH: 140 case DAY: 141 return true; 142 default: 143 return false; 144 } 145 } 146 147 private static GregorianCalendar toCalendarZulu(int theYear, int theMonth, int theDay) { 148 GregorianCalendar retVal = new GregorianCalendar(TimeZone.getTimeZone("GMT")); 149 retVal.set(Calendar.YEAR, theYear); 150 retVal.set(Calendar.MONTH, theMonth); 151 retVal.set(Calendar.DATE, theDay); 152 return retVal; 153 } 154}