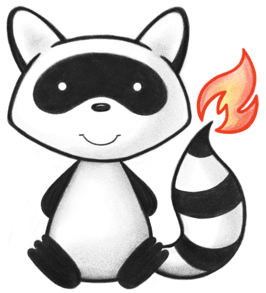
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 023import ca.uhn.fhir.model.api.annotation.DatatypeDef; 024import ca.uhn.fhir.model.api.annotation.SimpleSetter; 025import ca.uhn.fhir.parser.DataFormatException; 026 027import java.util.Date; 028import java.util.TimeZone; 029 030/** 031 * Represents a FHIR dateTime datatype. Valid precisions values for this type are: 032 * <ul> 033 * <li>{@link TemporalPrecisionEnum#YEAR} 034 * <li>{@link TemporalPrecisionEnum#MONTH} 035 * <li>{@link TemporalPrecisionEnum#DAY} 036 * <li>{@link TemporalPrecisionEnum#SECOND} 037 * <li>{@link TemporalPrecisionEnum#MILLI} 038 * </ul> 039 */ 040@DatatypeDef(name = "dateTime") 041public class DateTimeDt extends BaseDateTimeDt { 042 043 /** 044 * The default precision for this type 045 */ 046 public static final TemporalPrecisionEnum DEFAULT_PRECISION = TemporalPrecisionEnum.SECOND; 047 048 /** 049 * Constructor 050 */ 051 public DateTimeDt() { 052 super(); 053 } 054 055 /** 056 * Create a new DateTimeDt with seconds precision and the local time zone 057 */ 058 @SimpleSetter(suffix = "WithSecondsPrecision") 059 public DateTimeDt(@SimpleSetter.Parameter(name = "theDate") Date theDate) { 060 super(theDate, DEFAULT_PRECISION, TimeZone.getDefault()); 061 } 062 063 /** 064 * Constructor which accepts a date value and a precision value. Valid precisions values for this type are: 065 * <ul> 066 * <li>{@link TemporalPrecisionEnum#YEAR} 067 * <li>{@link TemporalPrecisionEnum#MONTH} 068 * <li>{@link TemporalPrecisionEnum#DAY} 069 * <li>{@link TemporalPrecisionEnum#SECOND} 070 * <li>{@link TemporalPrecisionEnum#MILLI} 071 * </ul> 072 * 073 * @throws DataFormatException 074 * If the specified precision is not allowed for this type 075 */ 076 @SimpleSetter 077 public DateTimeDt( 078 @SimpleSetter.Parameter(name = "theDate") Date theDate, 079 @SimpleSetter.Parameter(name = "thePrecision") TemporalPrecisionEnum thePrecision) { 080 super(theDate, thePrecision, TimeZone.getDefault()); 081 } 082 083 /** 084 * Create a new instance using a string date/time 085 * 086 * @throws DataFormatException 087 * If the specified precision is not allowed for this type 088 */ 089 public DateTimeDt(String theValue) { 090 super(theValue); 091 } 092 093 /** 094 * Constructor which accepts a date value, precision value, and time zone. Valid precisions values for this type 095 * are: 096 * <ul> 097 * <li>{@link TemporalPrecisionEnum#YEAR} 098 * <li>{@link TemporalPrecisionEnum#MONTH} 099 * <li>{@link TemporalPrecisionEnum#DAY} 100 * <li>{@link TemporalPrecisionEnum#SECOND} 101 * <li>{@link TemporalPrecisionEnum#MILLI} 102 * </ul> 103 */ 104 public DateTimeDt(Date theDate, TemporalPrecisionEnum thePrecision, TimeZone theTimezone) { 105 super(theDate, thePrecision, theTimezone); 106 } 107 108 @Override 109 protected boolean isPrecisionAllowed(TemporalPrecisionEnum thePrecision) { 110 switch (thePrecision) { 111 case YEAR: 112 case MONTH: 113 case DAY: 114 case SECOND: 115 case MILLI: 116 return true; 117 default: 118 return false; 119 } 120 } 121 122 /** 123 * Returns a new instance of DateTimeDt with the current system time and SECOND precision and the system local time 124 * zone 125 */ 126 public static DateTimeDt withCurrentTime() { 127 return new DateTimeDt(new Date(), TemporalPrecisionEnum.SECOND, TimeZone.getDefault()); 128 } 129 130 /** 131 * Returns the default precision for this datatype 132 * 133 * @see #DEFAULT_PRECISION 134 */ 135 @Override 136 protected TemporalPrecisionEnum getDefaultPrecisionForDatatype() { 137 return DEFAULT_PRECISION; 138 } 139}