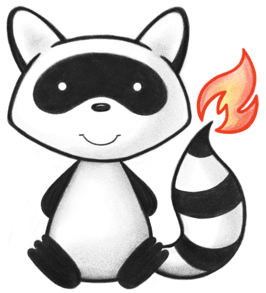
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.model.api.BasePrimitive; 023import ca.uhn.fhir.model.api.annotation.DatatypeDef; 024import ca.uhn.fhir.model.api.annotation.SimpleSetter; 025import org.hl7.fhir.instance.model.api.IBaseDecimalDatatype; 026 027import java.math.BigDecimal; 028import java.math.MathContext; 029import java.math.RoundingMode; 030 031@DatatypeDef(name = "decimal") 032public class DecimalDt extends BasePrimitive<BigDecimal> implements Comparable<DecimalDt>, IBaseDecimalDatatype { 033 034 /** 035 * Constructor 036 */ 037 public DecimalDt() { 038 super(); 039 } 040 041 /** 042 * Constructor 043 */ 044 @SimpleSetter 045 public DecimalDt(@SimpleSetter.Parameter(name = "theValue") BigDecimal theValue) { 046 setValue(theValue); 047 } 048 049 /** 050 * Constructor 051 */ 052 @SimpleSetter 053 public DecimalDt(@SimpleSetter.Parameter(name = "theValue") double theValue) { 054 // Use the valueOf here because the constructor gives crazy precision 055 // changes due to the floating point conversion 056 setValue(BigDecimal.valueOf(theValue)); 057 } 058 059 /** 060 * Constructor 061 */ 062 @SimpleSetter 063 public DecimalDt(@SimpleSetter.Parameter(name = "theValue") long theValue) { 064 setValue(new BigDecimal(theValue)); 065 } 066 067 /** 068 * Constructor 069 */ 070 public DecimalDt(String theValue) { 071 setValue(new BigDecimal(theValue)); 072 } 073 074 @Override 075 public int compareTo(DecimalDt theObj) { 076 if (getValue() == null && theObj.getValue() == null) { 077 return 0; 078 } 079 if (getValue() != null && (theObj == null || theObj.getValue() == null)) { 080 return 1; 081 } 082 if (getValue() == null && theObj.getValue() != null) { 083 return -1; 084 } 085 return getValue().compareTo(theObj.getValue()); 086 } 087 088 @Override 089 protected String encode(BigDecimal theValue) { 090 return getValue().toPlainString(); 091 } 092 093 /** 094 * Gets the value as an integer, using {@link BigDecimal#intValue()} 095 */ 096 public int getValueAsInteger() { 097 return getValue().intValue(); 098 } 099 100 public Number getValueAsNumber() { 101 return getValue(); 102 } 103 104 @Override 105 protected BigDecimal parse(String theValue) { 106 return new BigDecimal(theValue); 107 } 108 109 /** 110 * Rounds the value to the given prevision 111 * 112 * @see MathContext#getPrecision() 113 */ 114 public void round(int thePrecision) { 115 if (getValue() != null) { 116 BigDecimal newValue = getValue().round(new MathContext(thePrecision)); 117 setValue(newValue); 118 } 119 } 120 121 /** 122 * Rounds the value to the given prevision 123 * 124 * @see MathContext#getPrecision() 125 * @see MathContext#getRoundingMode() 126 */ 127 public void round(int thePrecision, RoundingMode theRoundingMode) { 128 if (getValue() != null) { 129 BigDecimal newValue = getValue().round(new MathContext(thePrecision, theRoundingMode)); 130 setValue(newValue); 131 } 132 } 133 134 /** 135 * Sets a new value using an integer 136 */ 137 public void setValueAsInteger(int theValue) { 138 setValue(new BigDecimal(theValue)); 139 } 140}