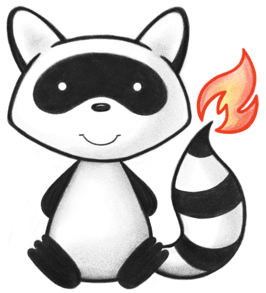
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.primitive; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.BasePrimitive; 025import ca.uhn.fhir.model.api.IQueryParameterType; 026import ca.uhn.fhir.model.api.annotation.DatatypeDef; 027import ca.uhn.fhir.model.api.annotation.SimpleSetter; 028import ca.uhn.fhir.rest.param.StringParam; 029import org.apache.commons.lang3.StringUtils; 030 031@DatatypeDef(name = "string") 032public class StringDt extends BasePrimitive<String> implements IQueryParameterType { 033 034 /** 035 * Create a new String 036 */ 037 public StringDt() { 038 super(); 039 } 040 041 /** 042 * Create a new String 043 */ 044 @SimpleSetter 045 public StringDt(@SimpleSetter.Parameter(name = "theString") String theValue) { 046 setValue(theValue); 047 } 048 049 public String getValueNotNull() { 050 return StringUtils.defaultString(getValue()); 051 } 052 053 /** 054 * Returns the value of this string, or <code>null</code> 055 */ 056 @Override 057 public String toString() { 058 return getValue(); 059 } 060 061 @Override 062 public int hashCode() { 063 final int prime = 31; 064 int result = 1; 065 result = prime * result + ((getValue() == null) ? 0 : getValue().hashCode()); 066 return result; 067 } 068 069 @Override 070 public boolean equals(Object obj) { 071 if (this == obj) return true; 072 if (obj == null) return false; 073 if (getClass() != obj.getClass()) return false; 074 StringDt other = (StringDt) obj; 075 if (getValue() == null) { 076 if (other.getValue() != null) return false; 077 } else if (!getValue().equals(other.getValue())) return false; 078 return true; 079 } 080 081 /** 082 * {@inheritDoc} 083 */ 084 @Override 085 public void setValueAsQueryToken( 086 FhirContext theContext, String theParamName, String theQualifier, String theValue) { 087 setValue(theValue); 088 } 089 090 /** 091 * {@inheritDoc} 092 */ 093 @Override 094 public String getValueAsQueryToken(FhirContext theContext) { 095 return getValue(); 096 } 097 098 /** 099 * Returns <code>true</code> if this datatype has no extensions, and has either a <code>null</code> value or an empty ("") value. 100 */ 101 @Override 102 public boolean isEmpty() { 103 boolean retVal = super.isBaseEmpty() && StringUtils.isBlank(getValue()); 104 return retVal; 105 } 106 107 @Override 108 public String getQueryParameterQualifier() { 109 return null; 110 } 111 112 @Override 113 protected String parse(String theValue) { 114 return theValue; 115 } 116 117 @Override 118 protected String encode(String theValue) { 119 return theValue; 120 } 121 122 /** 123 * <b>Not supported!</b> 124 * 125 * @deprecated get/setMissing is not supported in StringDt. Use {@link StringParam} instead if you 126 * need this functionality 127 */ 128 @Deprecated 129 @Override 130 public Boolean getMissing() { 131 return null; 132 } 133 134 /** 135 * <b>Not supported!</b> 136 * 137 * @deprecated get/setMissing is not supported in StringDt. Use {@link StringParam} instead if you 138 * need this functionality 139 */ 140 @Deprecated 141 @Override 142 public IQueryParameterType setMissing(Boolean theMissing) { 143 throw new UnsupportedOperationException( 144 Msg.code(1874) 145 + "get/setMissing is not supported in StringDt. Use {@link StringParam} instead if you need this functionality"); 146 } 147}