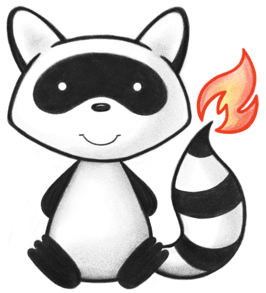
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.valueset; 021 022import ca.uhn.fhir.model.api.IValueSetEnumBinder; 023import ca.uhn.fhir.util.CoverageIgnore; 024 025import java.util.HashMap; 026import java.util.Map; 027 028@CoverageIgnore 029public enum BundleEntrySearchModeEnum { 030 MATCH("match", "http://hl7.org/fhir/search-entry-mode"), 031 INCLUDE("include", "http://hl7.org/fhir/search-entry-mode"), 032 OUTCOME("outcome", "http://hl7.org/fhir/search-entry-mode"), 033 ; 034 035 /** 036 * Identifier for this Value Set: 037 * http://hl7.org/fhir/vs/address-use 038 */ 039 public static final String VALUESET_IDENTIFIER = "http://hl7.org/fhir/bundle-entry-status"; 040 041 /** 042 * Name for this Value Set: 043 * AddressUse 044 */ 045 public static final String VALUESET_NAME = "BundleEntryStatus"; 046 047 private static Map<String, BundleEntrySearchModeEnum> CODE_TO_ENUM = 048 new HashMap<String, BundleEntrySearchModeEnum>(); 049 private static Map<String, Map<String, BundleEntrySearchModeEnum>> SYSTEM_TO_CODE_TO_ENUM = 050 new HashMap<String, Map<String, BundleEntrySearchModeEnum>>(); 051 052 private final String myCode; 053 private final String mySystem; 054 055 static { 056 for (BundleEntrySearchModeEnum next : BundleEntrySearchModeEnum.values()) { 057 CODE_TO_ENUM.put(next.getCode(), next); 058 059 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 060 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, BundleEntrySearchModeEnum>()); 061 } 062 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 063 } 064 } 065 066 /** 067 * Returns the code associated with this enumerated value 068 */ 069 public String getCode() { 070 return myCode; 071 } 072 073 /** 074 * Returns the code system associated with this enumerated value 075 */ 076 public String getSystem() { 077 return mySystem; 078 } 079 080 /** 081 * Returns the enumerated value associated with this code 082 */ 083 public static BundleEntrySearchModeEnum forCode(String theCode) { 084 BundleEntrySearchModeEnum retVal = CODE_TO_ENUM.get(theCode); 085 return retVal; 086 } 087 088 /** 089 * Converts codes to their respective enumerated values 090 */ 091 public static final IValueSetEnumBinder<BundleEntrySearchModeEnum> VALUESET_BINDER = 092 new IValueSetEnumBinder<BundleEntrySearchModeEnum>() { 093 094 private static final long serialVersionUID = -3836039426814809083L; 095 096 @Override 097 public String toCodeString(BundleEntrySearchModeEnum theEnum) { 098 return theEnum.getCode(); 099 } 100 101 @Override 102 public String toSystemString(BundleEntrySearchModeEnum theEnum) { 103 return theEnum.getSystem(); 104 } 105 106 @Override 107 public BundleEntrySearchModeEnum fromCodeString(String theCodeString) { 108 return CODE_TO_ENUM.get(theCodeString); 109 } 110 111 @Override 112 public BundleEntrySearchModeEnum fromCodeString(String theCodeString, String theSystemString) { 113 Map<String, BundleEntrySearchModeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 114 if (map == null) { 115 return null; 116 } 117 return map.get(theCodeString); 118 } 119 }; 120 121 /** 122 * Constructor 123 */ 124 BundleEntrySearchModeEnum(String theCode, String theSystem) { 125 myCode = theCode; 126 mySystem = theSystem; 127 } 128}