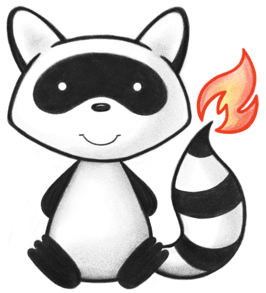
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020 021package ca.uhn.fhir.model.valueset; 022 023import ca.uhn.fhir.model.api.IValueSetEnumBinder; 024import ca.uhn.fhir.util.CoverageIgnore; 025 026import java.util.HashMap; 027import java.util.Map; 028 029/** 030 * This Enum is only used to support using the DSTU1 Bundle structure (<code>ca.uhn.fhir.model.api.Bundle</code>) 031 * on a DSTU2 server. It is preferably to use the new DSTU2 Bundle (<code>ca.uhn.fhir.model.dstu2.resource.Bundle</code>) 032 * for this purpose. 033 */ 034@CoverageIgnore 035public enum BundleEntryTransactionMethodEnum { 036 GET("GET", "http://hl7.org/fhir/http-verb"), 037 POST("POST", "http://hl7.org/fhir/http-verb"), 038 PUT("PUT", "http://hl7.org/fhir/http-verb"), 039 PATCH("PATCH", "http://hl7.org/fhir/http-verb"), 040 DELETE("DELETE", "http://hl7.org/fhir/http-verb"); 041 042 /** 043 * Identifier for this Value Set: 044 * http://hl7.org/fhir/vs/address-use 045 */ 046 public static final String VALUESET_IDENTIFIER = "http://hl7.org/fhir/http-verb"; 047 048 /** 049 * Name for this Value Set: 050 * AddressUse 051 */ 052 public static final String VALUESET_NAME = "BundleEntryStatus"; 053 054 private static Map<String, BundleEntryTransactionMethodEnum> CODE_TO_ENUM = 055 new HashMap<String, BundleEntryTransactionMethodEnum>(); 056 private static Map<String, Map<String, BundleEntryTransactionMethodEnum>> SYSTEM_TO_CODE_TO_ENUM = 057 new HashMap<String, Map<String, BundleEntryTransactionMethodEnum>>(); 058 059 private final String myCode; 060 private final String mySystem; 061 062 static { 063 for (BundleEntryTransactionMethodEnum next : BundleEntryTransactionMethodEnum.values()) { 064 CODE_TO_ENUM.put(next.getCode(), next); 065 066 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 067 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, BundleEntryTransactionMethodEnum>()); 068 } 069 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 070 } 071 } 072 073 /** 074 * Returns the code associated with this enumerated value 075 */ 076 public String getCode() { 077 return myCode; 078 } 079 080 /** 081 * Returns the code system associated with this enumerated value 082 */ 083 public String getSystem() { 084 return mySystem; 085 } 086 087 /** 088 * Returns the enumerated value associated with this code 089 */ 090 public BundleEntryTransactionMethodEnum forCode(String theCode) { 091 BundleEntryTransactionMethodEnum retVal = CODE_TO_ENUM.get(theCode); 092 return retVal; 093 } 094 095 /** 096 * Converts codes to their respective enumerated values 097 */ 098 public static final IValueSetEnumBinder<BundleEntryTransactionMethodEnum> VALUESET_BINDER = 099 new IValueSetEnumBinder<BundleEntryTransactionMethodEnum>() { 100 101 private static final long serialVersionUID = 7569681479045998433L; 102 103 @Override 104 public String toCodeString(BundleEntryTransactionMethodEnum theEnum) { 105 return theEnum.getCode(); 106 } 107 108 @Override 109 public String toSystemString(BundleEntryTransactionMethodEnum theEnum) { 110 return theEnum.getSystem(); 111 } 112 113 @Override 114 public BundleEntryTransactionMethodEnum fromCodeString(String theCodeString) { 115 return CODE_TO_ENUM.get(theCodeString); 116 } 117 118 @Override 119 public BundleEntryTransactionMethodEnum fromCodeString(String theCodeString, String theSystemString) { 120 Map<String, BundleEntryTransactionMethodEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 121 if (map == null) { 122 return null; 123 } 124 return map.get(theCodeString); 125 } 126 }; 127 128 /** 129 * Constructor 130 */ 131 BundleEntryTransactionMethodEnum(String theCode, String theSystem) { 132 myCode = theCode; 133 mySystem = theSystem; 134 } 135}