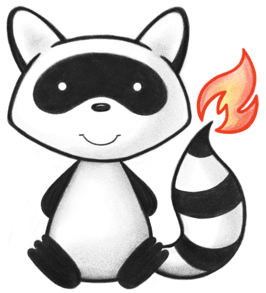
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.valueset; 021 022import ca.uhn.fhir.model.api.IValueSetEnumBinder; 023import ca.uhn.fhir.util.CoverageIgnore; 024 025import java.util.HashMap; 026import java.util.Map; 027 028@CoverageIgnore 029public enum BundleTypeEnum { 030 TRANSACTION("transaction", "http://hl7.org/fhir/bundle-type"), 031 032 DOCUMENT("document", "http://hl7.org/fhir/bundle-type"), 033 034 MESSAGE("message", "http://hl7.org/fhir/bundle-type"), 035 036 BATCH_RESPONSE("batch-response", "http://hl7.org/fhir/bundle-type"), 037 038 TRANSACTION_RESPONSE("transaction-response", "http://hl7.org/fhir/bundle-type"), 039 040 HISTORY("history", "http://hl7.org/fhir/bundle-type"), 041 042 SEARCHSET("searchset", "http://hl7.org/fhir/bundle-type"), 043 044 COLLECTION("collection", "http://hl7.org/fhir/bundle-type"), 045 ; 046 047 /** 048 * Identifier for this Value Set: 049 * http://hl7.org/fhir/vs/address-use 050 */ 051 public static final String VALUESET_IDENTIFIER = "http://hl7.org/fhir/bundle-type"; 052 053 /** 054 * Name for this Value Set: 055 * AddressUse 056 */ 057 public static final String VALUESET_NAME = "BundleType"; 058 059 private static Map<String, BundleTypeEnum> CODE_TO_ENUM = new HashMap<String, BundleTypeEnum>(); 060 private static Map<String, Map<String, BundleTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = 061 new HashMap<String, Map<String, BundleTypeEnum>>(); 062 063 private final String myCode; 064 private final String mySystem; 065 066 static { 067 for (BundleTypeEnum next : BundleTypeEnum.values()) { 068 CODE_TO_ENUM.put(next.getCode(), next); 069 070 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 071 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, BundleTypeEnum>()); 072 } 073 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 074 } 075 } 076 077 /** 078 * Returns the code associated with this enumerated value 079 */ 080 public String getCode() { 081 return myCode; 082 } 083 084 /** 085 * Returns the code system associated with this enumerated value 086 */ 087 public String getSystem() { 088 return mySystem; 089 } 090 091 /** 092 * Returns the enumerated value associated with this code 093 */ 094 public static BundleTypeEnum forCode(String theCode) { 095 BundleTypeEnum retVal = CODE_TO_ENUM.get(theCode); 096 return retVal; 097 } 098 099 /** 100 * Converts codes to their respective enumerated values 101 */ 102 public static final IValueSetEnumBinder<BundleTypeEnum> VALUESET_BINDER = 103 new IValueSetEnumBinder<BundleTypeEnum>() { 104 105 private static final long serialVersionUID = -305725916208867517L; 106 107 @Override 108 public String toCodeString(BundleTypeEnum theEnum) { 109 return theEnum.getCode(); 110 } 111 112 @Override 113 public String toSystemString(BundleTypeEnum theEnum) { 114 return theEnum.getSystem(); 115 } 116 117 @Override 118 public BundleTypeEnum fromCodeString(String theCodeString) { 119 return CODE_TO_ENUM.get(theCodeString); 120 } 121 122 @Override 123 public BundleTypeEnum fromCodeString(String theCodeString, String theSystemString) { 124 Map<String, BundleTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 125 if (map == null) { 126 return null; 127 } 128 return map.get(theCodeString); 129 } 130 }; 131 132 /** 133 * Constructor 134 */ 135 BundleTypeEnum(String theCode, String theSystem) { 136 myCode = theCode; 137 mySystem = theSystem; 138 } 139}