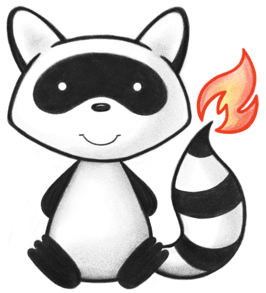
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.narrative; 021 022import ca.uhn.fhir.narrative2.NarrativeTemplateManifest; 023import org.apache.commons.lang3.Validate; 024 025import java.util.Arrays; 026import java.util.List; 027 028public class CustomThymeleafNarrativeGenerator extends BaseThymeleafNarrativeGenerator { 029 030 private volatile List<String> myPropertyFile; 031 private volatile NarrativeTemplateManifest myManifest; 032 033 /** 034 * Constructor. If this constructor is used you must explicitly call 035 * {@link #setManifest(NarrativeTemplateManifest)} to provide a template 036 * manifest before using the generator. 037 */ 038 public CustomThymeleafNarrativeGenerator() { 039 super(); 040 } 041 042 /** 043 * Create a new narrative generator 044 * 045 * @param theNarrativePropertyFiles The name of the property file, in one of the following formats: 046 * <ul> 047 * <li>file:/path/to/file/file.properties</li> 048 * <li>classpath:/com/package/file.properties</li> 049 * </ul> 050 */ 051 public CustomThymeleafNarrativeGenerator(String... theNarrativePropertyFiles) { 052 this(); 053 setPropertyFile(theNarrativePropertyFiles); 054 } 055 056 /** 057 * Create a new narrative generator 058 * 059 * @param theNarrativePropertyFiles The name of the property file, in one of the following formats: 060 * <ul> 061 * <li>file:/path/to/file/file.properties</li> 062 * <li>classpath:/com/package/file.properties</li> 063 * </ul> 064 */ 065 public CustomThymeleafNarrativeGenerator(List<String> theNarrativePropertyFiles) { 066 this(theNarrativePropertyFiles.toArray(new String[0])); 067 } 068 069 public CustomThymeleafNarrativeGenerator(CustomThymeleafNarrativeGenerator theNarrativeGenerator) { 070 setManifest(theNarrativeGenerator.getManifest()); 071 } 072 073 @Override 074 public NarrativeTemplateManifest getManifest() { 075 NarrativeTemplateManifest retVal = myManifest; 076 if (myManifest == null) { 077 Validate.isTrue(myPropertyFile != null, "Neither a property file or a manifest has been provided"); 078 retVal = NarrativeTemplateManifest.forManifestFileLocation(myPropertyFile); 079 setManifest(retVal); 080 } 081 return retVal; 082 } 083 084 public void setManifest(NarrativeTemplateManifest theManifest) { 085 myManifest = theManifest; 086 } 087 088 /** 089 * Set the property file to use 090 * 091 * @param thePropertyFile The name of the property file, in one of the following formats: 092 * <ul> 093 * <li>file:/path/to/file/file.properties</li> 094 * <li>classpath:/com/package/file.properties</li> 095 * </ul> 096 */ 097 public void setPropertyFile(String... thePropertyFile) { 098 Validate.notNull(thePropertyFile, "Property file can not be null"); 099 myPropertyFile = Arrays.asList(thePropertyFile); 100 myManifest = null; 101 } 102}