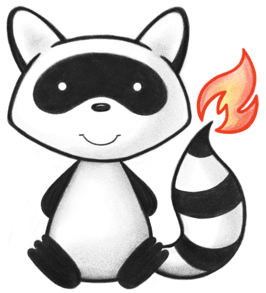
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser; 021 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.parser.json.BaseJsonLikeWriter; 024import ca.uhn.fhir.parser.json.JsonLikeStructure; 025import org.hl7.fhir.instance.model.api.IAnyResource; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028import java.io.IOException; 029 030/** 031 * An extension to the parser interface that is implemented by parsers that understand a generalized form of 032 * JSON data. This generalized form uses Map-like, List-like, and scalar elements to construct resources. 033 * <p> 034 * Thread safety: <b>Parsers are not guaranteed to be thread safe</b>. Create a new parser instance for every thread or 035 * every message being parsed/encoded. 036 * </p> 037 */ 038public interface IJsonLikeParser extends IParser { 039 040 void encodeResourceToJsonLikeWriter(IBaseResource theResource, BaseJsonLikeWriter theJsonLikeWriter) 041 throws IOException, DataFormatException; 042 043 /** 044 * Parses a resource from a JSON-like data structure 045 * 046 * @param theResourceType 047 * The resource type to use. This can be used to explicitly specify a class which extends a built-in type 048 * (e.g. a custom type extending the default Patient class) 049 * @param theJsonLikeStructure 050 * The JSON-like structure to parse 051 * @return A parsed resource 052 * @throws DataFormatException 053 * If the resource can not be parsed because the data is not recognized or invalid for any reason 054 */ 055 <T extends IBaseResource> T parseResource(Class<T> theResourceType, JsonLikeStructure theJsonLikeStructure) 056 throws DataFormatException; 057 058 /** 059 * Parses a resource from a JSON-like data structure 060 * 061 * @param theJsonLikeStructure 062 * The JSON-like structure to parse 063 * @return A parsed resource. Note that the returned object will be an instance of {@link IResource} or 064 * {@link IAnyResource} depending on the specific FhirContext which created this parser. 065 * @throws DataFormatException 066 * If the resource can not be parsed because the data is not recognized or invalid for any reason 067 */ 068 IBaseResource parseResource(JsonLikeStructure theJsonLikeStructure) throws DataFormatException; 069}