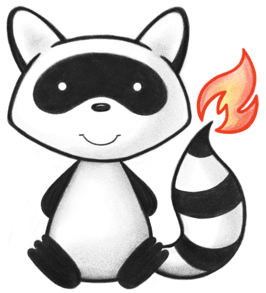
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser; 021 022import ca.uhn.fhir.parser.json.BaseJsonLikeValue.ScalarType; 023import ca.uhn.fhir.parser.json.BaseJsonLikeValue.ValueType; 024 025/** 026 * Error handler 027 */ 028public interface IParserErrorHandler { 029 030 /** 031 * Invoked when a contained resource is parsed that has no ID specified (and is therefore invalid) 032 * 033 * @param theLocation The location in the document. WILL ALWAYS BE NULL currently, as this is not yet implemented, but this parameter is included so that locations can be added in the future without 034 * changing the API. 035 * @since 2.0 036 */ 037 void containedResourceWithNoId(IParseLocation theLocation); 038 039 /** 040 * Invoked if the wrong type of element is found while parsing JSON. For example if a given element is 041 * expected to be a JSON Object and is a JSON array 042 * 043 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 044 * @param theElementName The name of the element that was found. 045 * @param theExpectedValueType The datatype that was expected at this location 046 * @param theExpectedScalarType If theExpectedValueType is {@link ValueType#SCALAR}, this is the specific scalar type expected. Otherwise this parameter will be null. 047 * @param theFoundValueType The datatype that was found at this location 048 * @param theFoundScalarType If theFoundValueType is {@link ValueType#SCALAR}, this is the specific scalar type found. Otherwise this parameter will be null. 049 * @since 2.2 050 */ 051 void incorrectJsonType( 052 IParseLocation theLocation, 053 String theElementName, 054 ValueType theExpectedValueType, 055 ScalarType theExpectedScalarType, 056 ValueType theFoundValueType, 057 ScalarType theFoundScalarType); 058 059 /** 060 * The parser detected an attribute value that was invalid (such as: empty "" values are not permitted) 061 * 062 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 063 * @param theValue The actual value 064 * @param theError A description of why the value was invalid 065 * @since 2.2 066 */ 067 void invalidValue(IParseLocation theLocation, String theValue, String theError); 068 069 /** 070 * Resource was missing a required element 071 * 072 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 073 * @param theElementName The missing element name 074 * @since 2.1 075 */ 076 void missingRequiredElement(IParseLocation theLocation, String theElementName); 077 078 /** 079 * Invoked when an element repetition (e.g. a second repetition of something) is found for a field 080 * which is non-repeating. 081 * 082 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 083 * @param theElementName The name of the element that was found. 084 * @since 1.2 085 */ 086 void unexpectedRepeatingElement(IParseLocation theLocation, String theElementName); 087 088 /** 089 * Invoked when an unknown element is found in the document. 090 * 091 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 092 * @param theAttributeName The name of the attribute that was found. 093 */ 094 void unknownAttribute(IParseLocation theLocation, String theAttributeName); 095 096 /** 097 * Invoked when an unknown element is found in the document. 098 * 099 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 100 * @param theElementName The name of the element that was found. 101 */ 102 void unknownElement(IParseLocation theLocation, String theElementName); 103 104 /** 105 * Resource contained a reference that could not be resolved and needs to be resolvable (e.g. because 106 * it is a local reference to an unknown contained resource) 107 * 108 * @param theLocation The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 109 * @param theReference The actual invalid reference (e.g. "#3") 110 * @since 2.0 111 */ 112 void unknownReference(IParseLocation theLocation, String theReference); 113 114 /** 115 * An extension contains both a value and at least one nested extension 116 * 117 * @param theLoc The location in the document. Note that this may be <code>null</code> as the ParseLocation feature is experimental. Use with caution, as the API may change. 118 */ 119 void extensionContainsValueAndNestedExtensions(IParseLocation theLocation); 120 121 /** 122 * For now this is an empty interface. Error handling methods include a parameter of this 123 * type which will currently always be set to null. This interface is included here so that 124 * locations can be added to the API in a future release without changing the API. 125 */ 126 interface IParseLocation { 127 128 /** 129 * Returns the name of the parent element (the element containing the element currently being parsed) 130 * 131 * @since 2.1 132 */ 133 String getParentElementName(); 134 } 135}