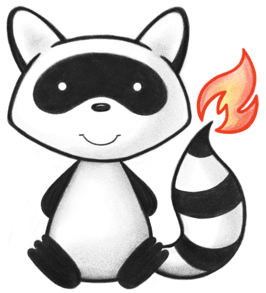
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.parser.json.BaseJsonLikeValue.ScalarType; 025import ca.uhn.fhir.parser.json.BaseJsonLikeValue.ValueType; 026import ca.uhn.fhir.util.UrlUtil; 027 028/** 029 * Parser error handler which throws a {@link DataFormatException} any time an 030 * issue is found while parsing. 031 * 032 * @see IParser#setParserErrorHandler(IParserErrorHandler) 033 * @see FhirContext#setParserErrorHandler(IParserErrorHandler) 034 */ 035public class StrictErrorHandler extends ParseErrorHandler implements IParserErrorHandler { 036 037 @Override 038 public void containedResourceWithNoId(IParseLocation theLocation) { 039 throw new DataFormatException(Msg.code(1819) + "Resource has contained child resource with no ID"); 040 } 041 042 @Override 043 public void incorrectJsonType( 044 IParseLocation theLocation, 045 String theElementName, 046 ValueType theExpected, 047 ScalarType theExpectedScalarType, 048 ValueType theFound, 049 ScalarType theFoundScalarType) { 050 String message = LenientErrorHandler.createIncorrectJsonTypeMessage( 051 theElementName, theExpected, theExpectedScalarType, theFound, theFoundScalarType); 052 throw new DataFormatException(Msg.code(1820) + message); 053 } 054 055 @Override 056 public void invalidValue(IParseLocation theLocation, String theValue, String theError) { 057 throw new DataFormatException(Msg.code(1821) + describeLocation(theLocation) + "Invalid attribute value \"" 058 + UrlUtil.sanitizeUrlPart(theValue) + "\": " + theError); 059 } 060 061 @Override 062 public void missingRequiredElement(IParseLocation theLocation, String theElementName) { 063 StringBuilder b = new StringBuilder(); 064 b.append("Resource is missing required element '"); 065 b.append(theElementName); 066 b.append("'"); 067 if (theLocation != null) { 068 b.append(" in parent element '"); 069 b.append(theLocation.getParentElementName()); 070 b.append("'"); 071 } 072 throw new DataFormatException(Msg.code(1822) + b.toString()); 073 } 074 075 @Override 076 public void unexpectedRepeatingElement(IParseLocation theLocation, String theElementName) { 077 throw new DataFormatException(Msg.code(1823) + describeLocation(theLocation) 078 + "Multiple repetitions of non-repeatable element '" + theElementName + "' found during parse"); 079 } 080 081 @Override 082 public void unknownAttribute(IParseLocation theLocation, String theAttributeName) { 083 throw new DataFormatException(Msg.code(1824) + describeLocation(theLocation) + "Unknown attribute '" 084 + theAttributeName + "' found during parse"); 085 } 086 087 @Override 088 public void unknownElement(IParseLocation theLocation, String theElementName) { 089 throw new DataFormatException(Msg.code(1825) + describeLocation(theLocation) + "Unknown element '" 090 + theElementName + "' found during parse"); 091 } 092 093 @Override 094 public void unknownReference(IParseLocation theLocation, String theReference) { 095 throw new DataFormatException( 096 Msg.code(1826) + describeLocation(theLocation) + "Resource has invalid reference: " + theReference); 097 } 098 099 @Override 100 public void extensionContainsValueAndNestedExtensions(IParseLocation theLocation) { 101 throw new DataFormatException(Msg.code(1827) + describeLocation(theLocation) 102 + "Extension contains both a value and nested extensions"); 103 } 104}