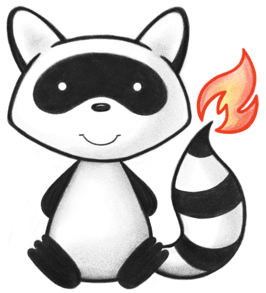
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser.json; 021 022import java.io.IOException; 023import java.io.Writer; 024import java.math.BigDecimal; 025import java.math.BigInteger; 026 027public abstract class BaseJsonLikeWriter { 028 029 private boolean prettyPrint; 030 private Writer writer; 031 032 public BaseJsonLikeWriter() { 033 super(); 034 } 035 036 public boolean isPrettyPrint() { 037 return prettyPrint; 038 } 039 040 public void setPrettyPrint(boolean tf) { 041 prettyPrint = tf; 042 } 043 044 public Writer getWriter() { 045 return writer; 046 } 047 048 public void setWriter(Writer writer) { 049 this.writer = writer; 050 } 051 052 public abstract BaseJsonLikeWriter init() throws IOException; 053 054 public abstract BaseJsonLikeWriter flush() throws IOException; 055 056 public abstract void close() throws IOException; 057 058 public abstract BaseJsonLikeWriter beginObject() throws IOException; 059 060 public abstract BaseJsonLikeWriter beginObject(String name) throws IOException; 061 062 public abstract BaseJsonLikeWriter beginArray(String name) throws IOException; 063 064 public abstract BaseJsonLikeWriter write(String value) throws IOException; 065 066 public abstract BaseJsonLikeWriter write(BigInteger value) throws IOException; 067 068 public abstract BaseJsonLikeWriter write(BigDecimal value) throws IOException; 069 070 public abstract BaseJsonLikeWriter write(long value) throws IOException; 071 072 public abstract BaseJsonLikeWriter write(double value) throws IOException; 073 074 public abstract BaseJsonLikeWriter write(Boolean value) throws IOException; 075 076 public abstract BaseJsonLikeWriter write(boolean value) throws IOException; 077 078 public abstract BaseJsonLikeWriter writeNull() throws IOException; 079 080 public abstract BaseJsonLikeWriter write(String name, String value) throws IOException; 081 082 public abstract BaseJsonLikeWriter write(String name, BigInteger value) throws IOException; 083 084 public abstract BaseJsonLikeWriter write(String name, BigDecimal value) throws IOException; 085 086 public abstract BaseJsonLikeWriter write(String name, long value) throws IOException; 087 088 public abstract BaseJsonLikeWriter write(String name, double value) throws IOException; 089 090 public abstract BaseJsonLikeWriter write(String name, Boolean value) throws IOException; 091 092 public abstract BaseJsonLikeWriter write(String name, boolean value) throws IOException; 093 094 public abstract BaseJsonLikeWriter endObject() throws IOException; 095 096 public abstract BaseJsonLikeWriter endArray() throws IOException; 097 098 public abstract BaseJsonLikeWriter endBlock() throws IOException; 099}