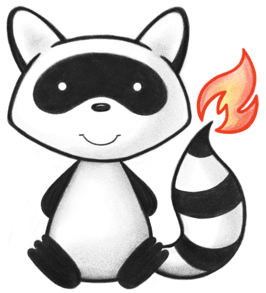
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser.json.jackson; 021 022import ca.uhn.fhir.parser.json.BaseJsonLikeWriter; 023import com.fasterxml.jackson.core.JsonFactory; 024import com.fasterxml.jackson.core.JsonGenerator; 025import com.fasterxml.jackson.core.util.DefaultIndenter; 026import com.fasterxml.jackson.core.util.DefaultPrettyPrinter; 027import com.fasterxml.jackson.core.util.Separators; 028 029import java.io.IOException; 030import java.io.Writer; 031import java.math.BigDecimal; 032import java.math.BigInteger; 033 034public class JacksonWriter extends BaseJsonLikeWriter { 035 036 private JsonGenerator myJsonGenerator; 037 038 public JacksonWriter(JsonFactory theJsonFactory, Writer theWriter) throws IOException { 039 myJsonGenerator = theJsonFactory.createGenerator(theWriter); 040 setWriter(theWriter); 041 } 042 043 public JacksonWriter() {} 044 045 @Override 046 public BaseJsonLikeWriter init() { 047 if (isPrettyPrint()) { 048 DefaultPrettyPrinter prettyPrinter = new DefaultPrettyPrinter() 049 .withSeparators(new Separators( 050 Separators.DEFAULT_ROOT_VALUE_SEPARATOR, 051 ':', 052 Separators.Spacing.AFTER, 053 ',', 054 Separators.Spacing.NONE, 055 ',', 056 Separators.Spacing.NONE)); 057 prettyPrinter = prettyPrinter.withObjectIndenter(new DefaultIndenter(" ", "\n")); 058 059 myJsonGenerator.setPrettyPrinter(prettyPrinter); 060 } 061 return this; 062 } 063 064 @Override 065 public BaseJsonLikeWriter flush() { 066 return this; 067 } 068 069 @Override 070 public void close() throws IOException { 071 myJsonGenerator.close(); 072 } 073 074 @Override 075 public BaseJsonLikeWriter beginObject() throws IOException { 076 myJsonGenerator.writeStartObject(); 077 return this; 078 } 079 080 @Override 081 public BaseJsonLikeWriter beginObject(String name) throws IOException { 082 myJsonGenerator.writeObjectFieldStart(name); 083 return this; 084 } 085 086 @Override 087 public BaseJsonLikeWriter beginArray(String name) throws IOException { 088 myJsonGenerator.writeArrayFieldStart(name); 089 return this; 090 } 091 092 @Override 093 public BaseJsonLikeWriter write(String value) throws IOException { 094 myJsonGenerator.writeObject(value); 095 return this; 096 } 097 098 @Override 099 public BaseJsonLikeWriter write(BigInteger value) throws IOException { 100 myJsonGenerator.writeObject(value); 101 return this; 102 } 103 104 @Override 105 public BaseJsonLikeWriter write(BigDecimal value) throws IOException { 106 myJsonGenerator.writeObject(value); 107 return this; 108 } 109 110 @Override 111 public BaseJsonLikeWriter write(long value) throws IOException { 112 myJsonGenerator.writeObject(value); 113 return this; 114 } 115 116 @Override 117 public BaseJsonLikeWriter write(double value) throws IOException { 118 myJsonGenerator.writeObject(value); 119 return this; 120 } 121 122 @Override 123 public BaseJsonLikeWriter write(Boolean value) throws IOException { 124 myJsonGenerator.writeObject(value); 125 return this; 126 } 127 128 @Override 129 public BaseJsonLikeWriter write(boolean value) throws IOException { 130 myJsonGenerator.writeObject(value); 131 return this; 132 } 133 134 @Override 135 public BaseJsonLikeWriter writeNull() throws IOException { 136 myJsonGenerator.writeNull(); 137 return this; 138 } 139 140 @Override 141 public BaseJsonLikeWriter write(String name, String value) throws IOException { 142 myJsonGenerator.writeObjectField(name, value); 143 return this; 144 } 145 146 @Override 147 public BaseJsonLikeWriter write(String name, BigInteger value) throws IOException { 148 myJsonGenerator.writeObjectField(name, value); 149 return this; 150 } 151 152 @Override 153 public BaseJsonLikeWriter write(String name, BigDecimal value) throws IOException { 154 myJsonGenerator.writeObjectField(name, value); 155 return this; 156 } 157 158 @Override 159 public BaseJsonLikeWriter write(String name, long value) throws IOException { 160 myJsonGenerator.writeObjectField(name, value); 161 return this; 162 } 163 164 @Override 165 public BaseJsonLikeWriter write(String name, double value) throws IOException { 166 myJsonGenerator.writeObjectField(name, value); 167 return this; 168 } 169 170 @Override 171 public BaseJsonLikeWriter write(String name, Boolean value) throws IOException { 172 myJsonGenerator.writeObjectField(name, value); 173 return this; 174 } 175 176 @Override 177 public BaseJsonLikeWriter write(String name, boolean value) throws IOException { 178 myJsonGenerator.writeObjectField(name, value); 179 return this; 180 } 181 182 @Override 183 public BaseJsonLikeWriter endObject() throws IOException { 184 myJsonGenerator.writeEndObject(); 185 return this; 186 } 187 188 @Override 189 public BaseJsonLikeWriter endArray() throws IOException { 190 myJsonGenerator.writeEndArray(); 191 return this; 192 } 193 194 @Override 195 public BaseJsonLikeWriter endBlock() throws IOException { 196 myJsonGenerator.writeEndObject(); 197 return this; 198 } 199}