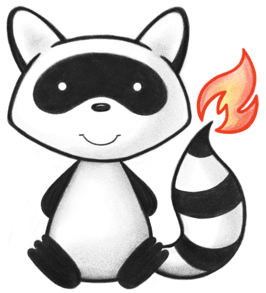
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.parser.path; 021 022import org.apache.commons.lang3.Validate; 023import org.apache.commons.lang3.builder.EqualsBuilder; 024import org.apache.commons.lang3.builder.HashCodeBuilder; 025 026public class EncodeContextPathElement { 027 private final String myName; 028 private final boolean myResource; 029 030 public EncodeContextPathElement(String theName, boolean theResource) { 031 Validate.notBlank(theName); 032 myName = theName; 033 myResource = theResource; 034 } 035 036 public boolean matches(EncodeContextPathElement theOther) { 037 if (myResource != theOther.isResource()) { 038 return false; 039 } 040 String otherName = theOther.getName(); 041 if (myName.equals(otherName)) { 042 return true; 043 } 044 /* 045 * This is here to handle situations where a path like 046 * Observation.valueQuantity has been specified as an include/exclude path, 047 * since we only know that path as 048 * Observation.value 049 * until we get to actually looking at the values there. 050 */ 051 if (myName.length() > otherName.length() && myName.startsWith(otherName)) { 052 char ch = myName.charAt(otherName.length()); 053 if (Character.isUpperCase(ch)) { 054 return true; 055 } 056 } 057 return myName.equals("*") || otherName.equals("*"); 058 } 059 060 @Override 061 public boolean equals(Object theO) { 062 if (this == theO) { 063 return true; 064 } 065 066 if (theO == null || getClass() != theO.getClass()) { 067 return false; 068 } 069 070 EncodeContextPathElement that = (EncodeContextPathElement) theO; 071 072 return new EqualsBuilder() 073 .append(myResource, that.myResource) 074 .append(myName, that.myName) 075 .isEquals(); 076 } 077 078 @Override 079 public int hashCode() { 080 return new HashCodeBuilder(17, 37).append(myName).append(myResource).toHashCode(); 081 } 082 083 @Override 084 public String toString() { 085 if (myResource) { 086 return myName + "(res)"; 087 } 088 return myName; 089 } 090 091 public String getName() { 092 return myName; 093 } 094 095 public boolean isResource() { 096 return myResource; 097 } 098}