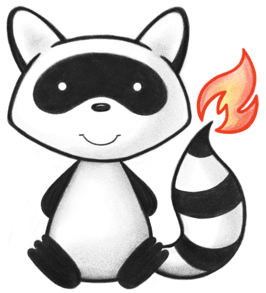
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.model.api.TagList; 024import ca.uhn.fhir.model.primitive.IdDt; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027import java.lang.annotation.ElementType; 028import java.lang.annotation.Retention; 029import java.lang.annotation.RetentionPolicy; 030import java.lang.annotation.Target; 031 032/** 033 * RESTful method annotation to be used for the FHIR <a 034 * href="http://hl7.org/implement/standards/fhir/http.html#tags">Tag 035 * Operations</a> which have to do with adding tags. 036 * <ul> 037 * <li> 038 * To add tag(s) <b>to the given resource 039 * instance</b>, this annotation should contain a {@link #type()} attribute 040 * specifying the resource type, and the method should have a parameter of type 041 * {@link IdDt} annotated with the {@link IdParam} annotation, as well as 042 * a parameter of type {@link TagList}. Note that this {@link TagList} parameter 043 * does not need to contain a complete list of tags for the resource, only a list 044 * of tags to be added. Server implementations must not remove tags based on this 045 * operation. 046 * Note that for a 047 * server implementation, the {@link #type()} annotation is optional if the 048 * method is defined in a <a href= 049 * "https://hapifhir.io/hapi-fhir/docs/server_plain/resource_providers.html" 050 * >resource provider</a>, since the type is implied.</li> 051 * <li> 052 * To add tag(s) on the server <b>to the given version of the 053 * resource instance</b>, this annotation should contain a {@link #type()} 054 * attribute specifying the resource type, and the method should have a 055 * parameter of type {@link IdDt} annotated with the {@link VersionIdParam} 056 * annotation, <b>and</b> a parameter of type {@link IdDt} annotated with the 057 * {@link IdParam} annotation, as well as 058 * a parameter of type {@link TagList}. Note that this {@link TagList} parameter 059 * does not need to contain a complete list of tags for the resource, only a list 060 * of tags to be added. Server implementations must not remove tags based on this 061 * operation. 062 * Note that for a server implementation, the 063 * {@link #type()} annotation is optional if the method is defined in a <a href= 064 * "https://hapifhir.io/hapi-fhir/docs/server_plain/resource_providers.html" 065 * >resource provider</a>, since the type is implied.</li> 066 * </ul> 067 */ 068@Target(value = ElementType.METHOD) 069@Retention(value = RetentionPolicy.RUNTIME) 070public @interface AddTags { 071 072 /** 073 * If set to a type other than the default (which is {@link IResource} 074 * , this method is expected to return a TagList containing only tags which 075 * are specific to the given resource type. 076 */ 077 Class<? extends IBaseResource> type() default IBaseResource.class; 078 079 /** 080 * This method allows the return type for this method to be specified in a 081 * non-type-specific way, using the text name of the resource, e.g. "Patient". 082 * 083 * This attribute should be populate, or {@link #type()} should be, but not both. 084 * 085 * @since 5.4.0 086 */ 087 String typeName() default ""; 088}