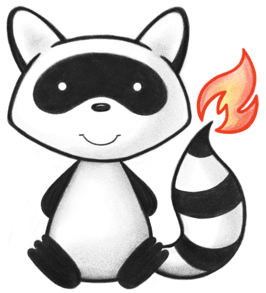
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.model.api.Include; 023 024import java.lang.annotation.ElementType; 025import java.lang.annotation.Retention; 026import java.lang.annotation.RetentionPolicy; 027import java.lang.annotation.Target; 028 029/** 030 * Method parameter which is used to indicate a parameter that will 031 * be populated with the "_include" (or "_revinclude") values for a search param. 032 * The parameter annotated with this annotation is used for either "_include" 033 * or "_revinclude", depending on whether {@link #reverse()} has been 034 * set to <code>true</code> (default is <code>false</code>). 035 * 036 * <p> 037 * Only up to two parameters may be annotated with this annotation (one each 038 * for <code>reverse=false</code> and <code>reverse=true</code>. That 039 * parameter should be one of the following: 040 * </p> 041 * <ul> 042 * <li><code>Collection<Include></code></li> 043 * <li><code>List<Include></code></li> 044 * <li><code>Set<Include></code></li> 045 * </ul> 046 * 047 * @see Include 048 */ 049@Retention(RetentionPolicy.RUNTIME) 050@Target(value = {ElementType.PARAMETER}) 051public @interface IncludeParam { 052 053 /** 054 * Optional value, if provided the server will only allow the values 055 * within the given set. If an _include parameter is passed to the server 056 * which does not match any allowed values the server will return an error. 057 * <p> 058 * Values for this parameter take the form that the FHIR specification 059 * defines for <code>_include</code> values, namely <code>[Resource Name].[path]</code>. 060 * For example: <code>"Patient.link.other"</code> 061 * or <code>"Encounter.partOf"</code> 062 * </p> 063 * <p> 064 * You may also pass in a value of "*" which indicates means that the 065 * client may request <code>_include=*</code>. This is a request to 066 * include all referenced resources as well as any resources referenced 067 * by those resources, etc. 068 * </p> 069 * <p> 070 * Leave this parameter empty if you do not want the server to declare or 071 * restrict which includes are allowable. In this case, the client may add 072 * any _include value they want, and that value will be accepted by the server 073 * and passed to the handling method. Note that this means that the server 074 * will not declare which _include values it supports in its conformance 075 * statement. 076 * </p> 077 */ 078 String[] allow() default {}; 079 080 /** 081 * If set to <code>true</code> (default is <code>false</code>), the values 082 * for this parameter correspond to the <code>_revinclude<code> parameter 083 * instead of the <code>_include<code> parameter. 084 */ 085 boolean reverse() default false; 086}