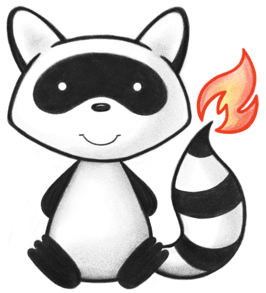
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.model.primitive.StringDt; 023import ca.uhn.fhir.rest.param.StringParam; 024import org.hl7.fhir.instance.model.api.IBase; 025 026import java.lang.annotation.ElementType; 027import java.lang.annotation.Retention; 028import java.lang.annotation.RetentionPolicy; 029import java.lang.annotation.Target; 030 031/** 032 */ 033@Retention(RetentionPolicy.RUNTIME) 034@Target(value = ElementType.PARAMETER) 035public @interface OperationParam { 036 037 /** 038 * Value for {@link OperationParam#max()} indicating no maximum 039 */ 040 int MAX_UNLIMITED = -1; 041 042 /** 043 * Value for {@link OperationParam#max()} indicating that the maximum will be inferred 044 * from the type. If the type is a single parameter type (e.g. <code>StringDt</code>, 045 * <code>TokenParam</code>, <code>IBaseResource</code>) the maximum will be 046 * <code>1</code>. 047 * <p> 048 * If the type is a collection, e.g. 049 * <code>List<StringDt></code> or <code>List<TokenOrListParam></code> 050 * the maximum will be set to <code>*</code>. If the param is a search parameter 051 * "and" type, such as <code>TokenAndListParam</code> the maximum will also be 052 * set to <code>*</code> 053 * </p> 054 * 055 * @since 1.5 056 */ 057 int MAX_DEFAULT = -2; 058 059 /** 060 * The name of the parameter 061 */ 062 String name(); 063 064 /** 065 * The type of the parameter. This will only have effect on <code>@OperationParam</code> 066 * annotations specified as values for {@link Operation#returnParameters()}, otherwise the 067 * value will be ignored. Value should be one of: 068 * <ul> 069 * <li>A resource type, e.g. <code>Patient.class</code></li> 070 * <li>A datatype, e.g. <code>{@link StringDt}.class</code> or </code>CodeableConceptDt.class</code> 071 * <li>A RESTful search parameter type, e.g. <code>{@link StringParam}.class</code> 072 * </ul> 073 */ 074 Class<? extends IBase> type() default IBase.class; 075 076 /** 077 * Optionally specifies the type of the parameter as a string, such as <code>Coding</code> or 078 * <code>base64Binary</code>. This can be useful if you want to use a generic interface type 079 * on the actual method,such as {@link org.hl7.fhir.instance.model.api.IPrimitiveType} or 080 * {@link org.hl7.fhir.instance.model.api.ICompositeType}. 081 */ 082 String typeName() default ""; 083 084 /** 085 * The minimum number of repetitions allowed for this child (default is 0) 086 */ 087 int min() default 0; 088 089 /** 090 * The maximum number of repetitions allowed for this child. Should be 091 * set to {@link #MAX_UNLIMITED} if there is no limit to the number of 092 * repetitions. See {@link #MAX_DEFAULT} for a description of the default 093 * behaviour. 094 */ 095 int max() default MAX_DEFAULT; 096}