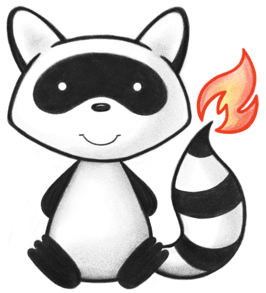
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.model.api.IQueryParameterType; 023import ca.uhn.fhir.rest.param.CompositeParam; 024import ca.uhn.fhir.rest.param.ReferenceParam; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027import java.lang.annotation.ElementType; 028import java.lang.annotation.Retention; 029import java.lang.annotation.RetentionPolicy; 030import java.lang.annotation.Target; 031 032/** 033 * Parameter annotation which specifies a search parameter for a {@link Search} method. 034 */ 035@Retention(RetentionPolicy.RUNTIME) 036@Target(value = ElementType.PARAMETER) 037public @interface OptionalParam { 038 039 public static final String ALLOW_CHAIN_ANY = "*"; 040 041 public static final String ALLOW_CHAIN_NOTCHAINED = ""; 042 043 /** 044 * For reference parameters ({@link ReferenceParam}) this value may be 045 * used to indicate which chain values (if any) are <b>not</b> valid 046 * for the given parameter. Values here will supercede any values specified 047 * in {@link #chainWhitelist()} 048 * <p> 049 * If the parameter annotated with this annotation is not a {@link ReferenceParam}, 050 * this value must not be populated. 051 * </p> 052 */ 053 String[] chainBlacklist() default {}; 054 055 /** 056 * For reference parameters ({@link ReferenceParam}) this value may be 057 * used to indicate which chain values (if any) are valid for the given 058 * parameter. If the list contains the value {@link #ALLOW_CHAIN_ANY}, all values are valid. (this is the default) 059 * If the list contains the value {@link #ALLOW_CHAIN_NOTCHAINED} 060 * then the reference param only supports the empty chain (i.e. the resource 061 * ID). 062 * <p> 063 * Valid values for this parameter include: 064 * </p> 065 * <ul> 066 * <li><code>chainWhitelist={ OptionalParam.ALLOW_CHAIN_NOTCHAINED }</code> - Only allow resource reference (no chaining allowed for this parameter)</li> 067 * <li><code>chainWhitelist={ OptionalParam.ALLOW_CHAIN_ANY }</code> - Allow any chaining at all (including a non chained value, <b>this is the default</b>)</li> 068 * <li><code>chainWhitelist={ "foo", "bar" }</code> - Allow property.foo and property.bar</li> 069 * </ul> 070 * <p> 071 * Any values specified in 072 * {@link #chainBlacklist()} will supercede (have priority over) values 073 * here. 074 * </p> 075 * <p> 076 * If the parameter annotated with this annotation is not a {@link ReferenceParam}, 077 * this value must not be populated. 078 * </p> 079 */ 080 String[] chainWhitelist() default {ALLOW_CHAIN_ANY}; 081 082 /** 083 * For composite parameters ({@link CompositeParam}) this value may be 084 * used to indicate the parameter type(s) which may be referenced by this param. 085 * <p> 086 * If the parameter annotated with this annotation is not a {@link CompositeParam}, 087 * this value must not be populated. 088 * </p> 089 */ 090 Class<? extends IQueryParameterType>[] compositeTypes() default {}; 091 092 /** 093 * This is the name for the parameter. Generally this should be a 094 * simple string (e.g. "name", or "identifier") which will be the name 095 * of the URL parameter used to populate this method parameter. 096 * <p> 097 * Most resource model classes have constants which may be used to 098 * supply values for this field, e.g. <code>Patient.SP_NAME</code> or 099 * <code>Observation.SP_DATE</code> 100 * </p> 101 * <p> 102 * If you wish to specify a parameter for a resource reference which 103 * only accepts a specific chained value, it is also valid to supply 104 * a chained name here, such as "patient.name". It is recommended to 105 * supply this using constants where possible, e.g. 106 * <code>Observation.SP_SUBJECT + '.' + Patient.SP_IDENTIFIER</code> 107 * </p> 108 */ 109 String name(); 110 111 /** 112 * For resource reference parameters ({@link ReferenceParam}) this value may be 113 * used to indicate the resource type(s) which may be referenced by this param. 114 * <p> 115 * If the parameter annotated with this annotation is not a {@link ReferenceParam}, 116 * this value must not be populated. 117 * </p> 118 */ 119 Class<? extends IBaseResource>[] targetTypes() default {}; 120}