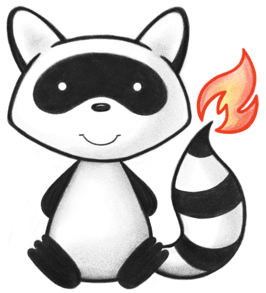
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.rest.client.api.IBasicClient; 023import ca.uhn.fhir.rest.client.api.IRestfulClient; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.lang.annotation.ElementType; 027import java.lang.annotation.Retention; 028import java.lang.annotation.RetentionPolicy; 029import java.lang.annotation.Target; 030 031/** 032 * RESTful method annotation to be used for the FHIR <a href="http://hl7.org/implement/standards/fhir/http.html#read">read</a> and <a 033 * href="http://hl7.org/implement/standards/fhir/http.html#vread">vread</a> method. 034 * 035 * <p> 036 * If this method has a parameter annotated with the {@link IdParam} annotation and a parameter annotated with the {@link VersionIdParam} annotation, the method will be treated as a vread method. If 037 * the method has only a parameter annotated with the {@link IdParam} annotation, it will be treated as a read operation. 038 * the 039 * </p> 040 * <p> 041 * If you wish for your server to support both read and vread operations, you will need 042 * two methods annotated with this annotation. 043 * </p> 044 */ 045@Retention(RetentionPolicy.RUNTIME) 046@Target(ElementType.METHOD) 047public @interface Read { 048 049 /** 050 * The return type for this method. This generally does not need to be populated for IResourceProvider in a server implementation, but often does need to be populated in 051 * client implementations using {@link IBasicClient} or {@link IRestfulClient}, or in plain providers on a server. 052 * <p> 053 * This value also does not need to be populated if the return type for a method annotated with this annotation is sufficient to determine the type of resource provided. E.g. if the method returns 054 * <code>Patient</code> or <code>List<Patient></code>, the server/client will automatically determine that the Patient resource is the return type, and this value may be left blank. 055 * </p> 056 */ 057 // NB: Read, Search (maybe others) share this annotation, so update the javadocs everywhere 058 Class<? extends IBaseResource> type() default IBaseResource.class; 059 060 /** 061 * This method allows the return type for this method to be specified in a 062 * non-type-specific way, using the text name of the resource, e.g. "Patient". 063 * 064 * This attribute should be populate, or {@link #type()} should be, but not both. 065 * 066 * @since 5.4.0 067 */ 068 String typeName() default ""; 069 070 /** 071 * If set to true (default is false), this method supports vread operation as well as read 072 */ 073 boolean version() default false; 074}