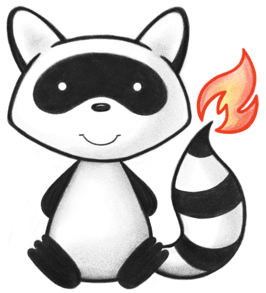
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.annotation; 021 022import ca.uhn.fhir.rest.api.ValidationModeEnum; 023import org.hl7.fhir.instance.model.api.IBaseResource; 024 025import java.lang.annotation.ElementType; 026import java.lang.annotation.Retention; 027import java.lang.annotation.RetentionPolicy; 028import java.lang.annotation.Target; 029 030/** 031 * RESTful method annotation to be used for the FHIR 032 * <a href="http://hl7.org/implement/standards/fhir/http.html#validate">validate</a> method. 033 * 034 * <p> 035 * Validate is used to accept a resource, and test whether it would be acceptable for 036 * storing (e.g. using an update or create method) 037 * </p> 038 * <p> 039 * <b>FHIR Version Note:</b> The validate operation was defined as a type operation in DSTU1 040 * using a URL syntax like <code>http://example.com/Patient/_validate</code>. In DSTU2, validation 041 * has been switched to being an extended operation using a URL syntax like 042 * <code>http://example.com/Patient/$validate</code>, with a n 043 * </p> 044 */ 045@Retention(RetentionPolicy.RUNTIME) 046@Target(value = ElementType.METHOD) 047public @interface Validate { 048 049 /** 050 * The return type for this method. This generally does not need 051 * to be populated for a server implementation (using an IResourceProvider, 052 * since resource providers will return only one resource type per class, 053 * but generally does need to be populated for client implementations. 054 */ 055 // NB: Read, Search (maybe others) share this annotation, so update the javadocs everywhere 056 Class<? extends IBaseResource> type() default IBaseResource.class; 057 058 /** 059 * This method allows the return type for this method to be specified in a 060 * non-type-specific way, using the text name of the resource, e.g. "Patient". 061 * 062 * This attribute should be populate, or {@link #type()} should be, but not both. 063 * 064 * @since 5.4.0 065 */ 066 String typeName() default ""; 067 068 /** 069 * Validation mode parameter annotation for the validation mode parameter (only supported 070 * in FHIR DSTU2+). Parameter must be of type {@link ValidationModeEnum}. 071 */ 072 @Retention(RetentionPolicy.RUNTIME) 073 @Target(value = ElementType.PARAMETER) 074 @interface Mode { 075 // nothing 076 } 077 078 /** 079 * Validation mode parameter annotation for the validation URI parameter (only supported 080 * in FHIR DSTU2+). Parameter must be of type {@link String}. 081 */ 082 @Retention(RetentionPolicy.RUNTIME) 083 @Target(value = ElementType.PARAMETER) 084 @interface Profile { 085 // nothing 086 } 087}