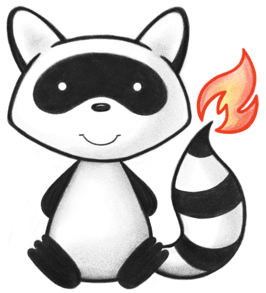
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import ca.uhn.fhir.context.api.BundleInclusionRule; 023import ca.uhn.fhir.model.api.Include; 024import ca.uhn.fhir.model.valueset.BundleTypeEnum; 025import jakarta.annotation.Nonnull; 026import jakarta.annotation.Nullable; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028import org.hl7.fhir.instance.model.api.IPrimitiveType; 029 030import java.util.ArrayList; 031import java.util.Date; 032import java.util.List; 033import java.util.Set; 034 035/** 036 * This interface should be considered experimental and will likely change in future releases of HAPI. Use with caution! 037 */ 038public interface IVersionSpecificBundleFactory { 039 040 void addResourcesToBundle( 041 List<IBaseResource> theResult, 042 BundleTypeEnum theBundleType, 043 String theServerBase, 044 @Nullable BundleInclusionRule theBundleInclusionRule, 045 @Nullable Set<Include> theIncludes); 046 047 void addRootPropertiesToBundle( 048 String theId, 049 @Nonnull BundleLinks theBundleLinks, 050 Integer theTotalResults, 051 IPrimitiveType<Date> theLastUpdated); 052 053 IBaseResource getResourceBundle(); 054 055 /** 056 * @deprecated This was deprecated in HAPI FHIR 4.1.0 as it provides duplicate functionality to the {@link #addRootPropertiesToBundle(String, BundleLinks, Integer, IPrimitiveType<Date>)} 057 * and {@link #addResourcesToBundle(List, BundleTypeEnum, String, BundleInclusionRule, Set)} methods 058 */ 059 @Deprecated 060 default void initializeBundleFromResourceList( 061 String theAuthor, 062 List<? extends IBaseResource> theResult, 063 String theServerBase, 064 String theCompleteUrl, 065 int theTotalResults, 066 BundleTypeEnum theBundleType) { 067 addTotalResultsToBundle(theResult.size(), theBundleType); 068 addResourcesToBundle(new ArrayList<>(theResult), theBundleType, null, null, null); 069 } 070 071 void initializeWithBundleResource(IBaseResource theResource); 072 073 List<IBaseResource> toListOfResources(); 074 075 void addTotalResultsToBundle(Integer theTotalResults, BundleTypeEnum theBundleType); 076}