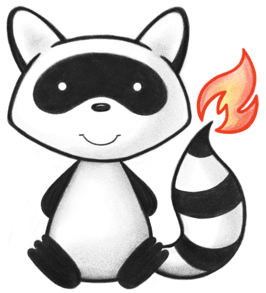
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import jakarta.annotation.Nullable; 023 024import java.util.HashMap; 025 026/** 027 * Represents values for "return" value as provided in the the <a href="https://tools.ietf.org/html/rfc7240#section-4.2">HTTP Prefer header</a>. 028 */ 029public enum PreferReturnEnum { 030 REPRESENTATION(Constants.HEADER_PREFER_RETURN_REPRESENTATION), 031 MINIMAL(Constants.HEADER_PREFER_RETURN_MINIMAL), 032 OPERATION_OUTCOME(Constants.HEADER_PREFER_RETURN_OPERATION_OUTCOME); 033 034 private static HashMap<String, PreferReturnEnum> ourValues; 035 private final String myHeaderValue; 036 037 PreferReturnEnum(String theHeaderValue) { 038 myHeaderValue = theHeaderValue; 039 } 040 041 public String getHeaderValue() { 042 return myHeaderValue; 043 } 044 045 @Nullable 046 public static PreferReturnEnum fromHeaderValue(String theHeaderValue) { 047 if (ourValues == null) { 048 HashMap<String, PreferReturnEnum> values = new HashMap<>(); 049 for (PreferReturnEnum next : PreferReturnEnum.values()) { 050 values.put(next.getHeaderValue(), next); 051 } 052 ourValues = values; 053 } 054 return ourValues.get(theHeaderValue); 055 } 056}